G2lib Namespace Reference
Clothoids
|
Classes | |
class | AsyPlot |
class | BaseCurve |
class | BBox |
class | Biarc |
class | BiarcList |
class | CircleArc |
class | ClothoidCurve |
class | ClothoidList |
class | ClothoidSplineG2 |
class | Dubins |
class | Dubins3p |
class | G2solve2arc |
class | G2solve3arc |
class | G2solveCLC |
class | LineSegment |
class | PolyLine |
Class to manage a collection of straight segment. More... | |
class | Solve2x2 |
class | Triangle2D |
Typedefs | |
using | istream_type = std::basic_istream<char> |
input streaming | |
using | ostream_type = std::basic_ostream<char> |
output streaming | |
using | real_type = double |
real type number | |
using | integer = int |
integer type number | |
using | AABB_TREE = Utils::AABBtree<real_type> |
AABB tree type | |
using | AABB_SET = Utils::AABBtree<real_type>::AABB_SET |
Set type used in AABB tree object. | |
using | AABB_MAP = Utils::AABBtree<real_type>::AABB_MAP |
Map type used in AABB tree object. | |
using | GenericContainer = GC_namespace::GenericContainer |
Generic container object. | |
using | CurveType |
using | Ipair = std::pair<real_type,real_type> |
Pair of two real number. | |
using | IntersectList = std::vector<Ipair> |
Vector of pair of two real number. | |
using | DubinsType |
using | Dubins3pBuildType |
Detailed Description
file: BBox.cc
file: Biarc.cc
file: BaseCurve.hh
file: BBox.hxx
file: Biarc.hxx
file: BiarcList.hh
file: Circle.hxx
file: Clothoid.hxx
file: ClothoidList.hxx
file: Dubins.hxx
file: Dubins3p.hxx
file: Fresnel.hxx
file: G2lib.hh Clothoid computations routine namespace
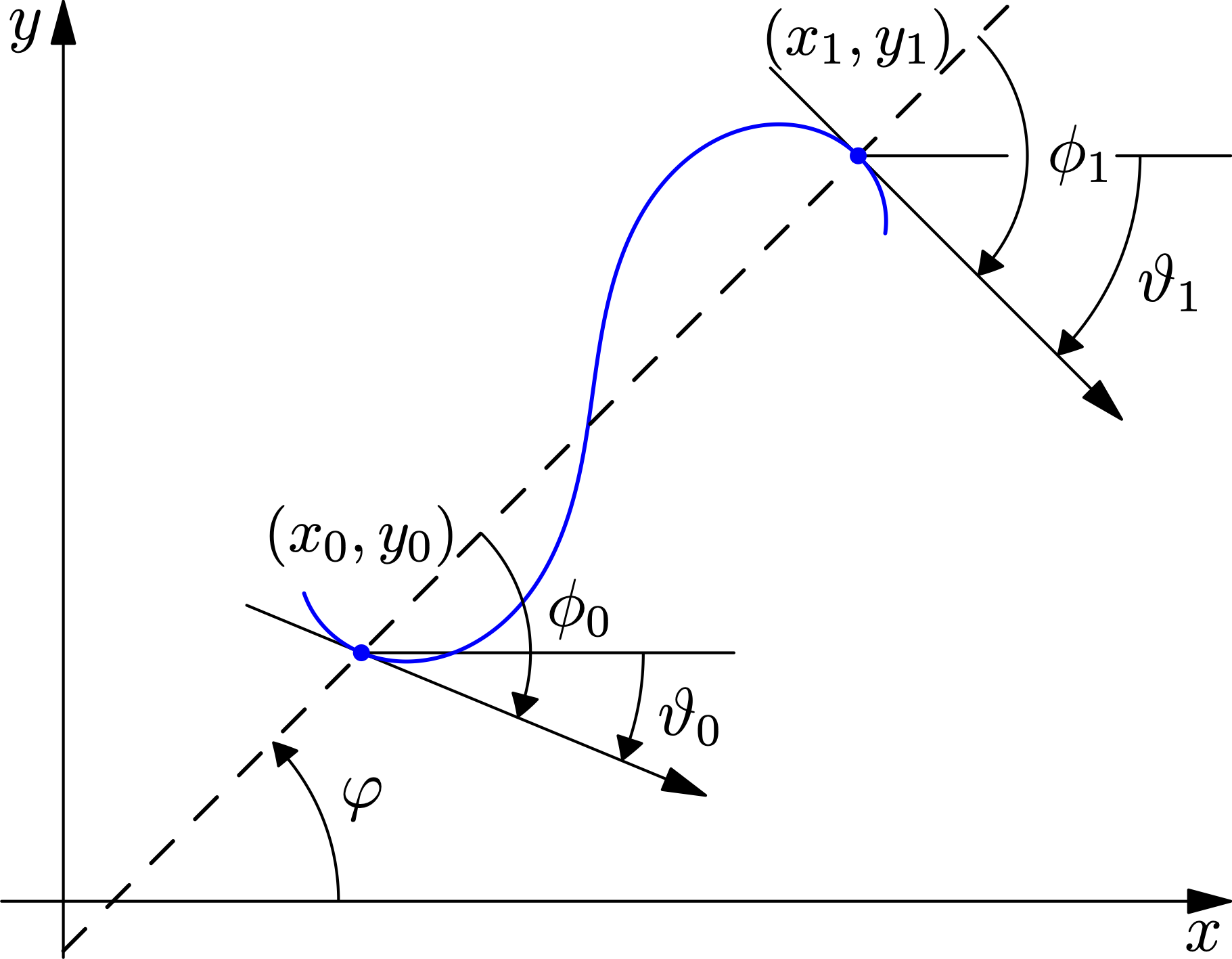
file: Line.hxx
file: PolyLine.hxx
file: Triangle2D.hxx
file: Dubins.cc
file: Dubins3p.cc
file: Dubins3p_ellipse.cc
file: Dubins3p_pattern.cc
Typedef Documentation
◆ CurveType
using G2lib::CurveType |
Enumeration type for curve type
◆ Dubins3pBuildType
using G2lib::Dubins3pBuildType |
Type of Dubins for three points construction algorithm
- SAMPLE_ONE_DEGREE search solution by sampling a fixed angles
- PATTERN_SEARCH search solution by using pattern search
- PATTERN_TRICHOTOMY search solution by using pattern search based on tricotomy
- PATTERN_SEARCH_WITH_ALGO748 search solution by using pattern search and refinement using ALGO748
- PATTERN_TRICHOTOMY_WITH_ALGO748 search solution by using pattern search with tricotomy and refinement using ALGO748
- ELLIPSE search solution by using ellipse geometric construction
- POLYNOMIAL_SYSTEM search solution by solving polinomial system
◆ DubinsType
using G2lib::DubinsType |
Type of Dubins solution
- LSL left-stright-left solution
- RSR right-stright-right solution
- LSR left-stright-right solution
- RSL right-stright-left solution
- LRL left-right-left solution
- RLR right-left-right solution
Function Documentation
◆ build_guess_theta()
bool G2lib::build_guess_theta | ( | integer | n, |
real_type const | x[], | ||
real_type const | y[], | ||
real_type | theta[] ) |
Given a list of points \( (x_i,y_i) \) build a guess of angles for a spline of biarc.
- Parameters
-
[in] n number of points [in] x \(x\)-coordinates [in] y \(y\)-coordinates [out] theta guessed angles
◆ collision()
Check curve collision
- Parameters
-
[in] pC1 first curve [in] pC2 second curve
- Returns
true
if the curves collide
◆ collision_ISO()
bool G2lib::collision_ISO | ( | BaseCurve const * | pC1, |
real_type | offs_C1, | ||
BaseCurve const * | pC2, | ||
real_type | offs_C2 ) |
Check curve collision
- Parameters
-
[in] pC1 first curve [in] offs_C1 offset of the first curve [in] pC2 second curve [in] offs_C2 offset of the second curve
- Returns
true
if the curves collide
◆ collision_SAE()
|
inline |
Check curve collision
- Parameters
-
[in] pC1 first curve [in] offs_C1 offset of the first curve [in] pC2 second curve [in] offs_C2 offset of the second curve
- Returns
true
if the curves collide
◆ curve_promote()
Given two curve type determine curve type that cointain both type
- Parameters
-
[in] A first curve type [in] B second curve type
- Returns
- the curve type super type of both
◆ Dubins_build()
bool G2lib::Dubins_build | ( | real_type | x0, |
real_type | y0, | ||
real_type | theta0, | ||
real_type | x3, | ||
real_type | y3, | ||
real_type | theta3, | ||
real_type | k_max, | ||
DubinsType & | type, | ||
real_type & | L1, | ||
real_type & | L2, | ||
real_type & | L3, | ||
real_type | grad[2] ) |
Given two points
\[ (x_0,y_0),\qquad (x_3,y_3) \]
two angles
\[ \theta_0,\qquad \theta_1 \]
and a maximum of curvature \( \kappa_{\max} \)
compute the solution of the Dubins problem
- Parameters
-
[in] x0 coordinate \( x_0 \) [in] y0 coordinate \( y_0 \) [in] theta0 angle \( \theta_0 \) [in] x3 coordinate \( x_3 \) [in] y3 coordinate \( y_3 \) [in] theta3 angle \( \theta_3 \) [in] k_max max curvature \( \kappa_{\max} \) [out] type solution type [out] L1 length of first arc [out] L2 length of second arc [out] L3 length of third arc [out] grad gradient of the solution respect to initial and final angle
- Returns
true
if a solution is found
◆ FresnelCS() [1/2]
Compute Fresnel integrals and its derivatives
\[ C(x) = \int_0^x \cos\left(\frac{\pi}{2}t^2\right) \mathrm{d}t, \qquad S(x) = \int_0^x \sin\left(\frac{\pi}{2}t^2\right) \mathrm{d}t \]
- Parameters
-
nk maximum order of the derivative x the input abscissa S S[0]= \( S(x) \), S[1]= \( S'(x) \), S[2]= \( S''(x) \) C C[0]= \( C(x) \), C[1]= \( C'(x) \), C[2]= \( C''(x) \)
◆ FresnelCS() [2/2]
Purpose:
Compute Fresnel integrals C(x) and S(x)
\[ S(x) = \int_0^x \sin t^2 \,\mathrm{d} t, \qquad C(x) = \int_0^x \cos t^2 \,\mathrm{d} t \]
Example:
\( x \) | \( C(x) \) | \( S(x) \) |
---|---|---|
0.0 | 0.00000000 | 0.00000000 |
0.5 | 0.49234423 | 0.06473243 |
1.0 | 0.77989340 | 0.43825915 |
1.5 | 0.44526118 | 0.69750496 |
2.0 | 0.48825341 | 0.34341568 |
2.5 | 0.45741301 | 0.61918176 |
Adapted from:
- William J. Thompson, Atlas for computing mathematical functions : an illustrated guide for practitioners, with programs in C and Mathematica, Wiley, 1997.
Author:
- Venkata Sivakanth Telasula, email: sivak.nosp@m.anth.nosp@m..tela.nosp@m.sula.nosp@m.@gmai.nosp@m.l.co.nosp@m.m, date: August 11, 2005
- Parameters
-
[in] y Argument of \(C(y)\) and \(S(y)\) [out] C \(C(x)\) [out] S \(S(x)\)
◆ GeneralizedFresnelCS() [1/2]
void G2lib::GeneralizedFresnelCS | ( | integer | nk, |
real_type | a, | ||
real_type | b, | ||
real_type | c, | ||
real_type | intC[], | ||
real_type | intS[] ) |
Compute the Fresnel integrals
\[ \int_0^1 t^k \cos\left(a\frac{t^2}{2} + b t + c\right)\,\mathrm{d}t,\qquad \int_0^1 t^k \sin\left(a\frac{t^2}{2} + b t + c\right)\,\mathrm{d}t \]
- Parameters
-
nk number of momentae to compute a parameter \( a \) b parameter \( b \) c parameter \( c \) intC cosine integrals, intS sine integrals
◆ GeneralizedFresnelCS() [2/2]
void G2lib::GeneralizedFresnelCS | ( | real_type | a, |
real_type | b, | ||
real_type | c, | ||
real_type & | intC, | ||
real_type & | intS ) |
Compute the Fresnel integrals
\[ \int_0^1 t^k \cos\left(a\frac{t^2}{2} + b t + c\right)\,\mathrm{d}t,\qquad \int_0^1 t^k \sin\left(a\frac{t^2}{2} + b t + c\right)\,\mathrm{d}t \]
- Parameters
-
a parameter \( a \) b parameter \( b \) c parameter \( c \) intC cosine integrals, intS sine integrals
◆ intersect()
void G2lib::intersect | ( | BaseCurve const * | pC1, |
BaseCurve const * | pC2, | ||
IntersectList & | ilist ) |
Compute curve intersections
- Parameters
-
[in] pC1 first curve [in] pC2 second curve [out] ilist list of the intersection (as parameter on the curves)
◆ intersect_ISO()
void G2lib::intersect_ISO | ( | BaseCurve const * | pC1, |
real_type | offs_C1, | ||
BaseCurve const * | pC2, | ||
real_type | offs_C2, | ||
IntersectList & | ilist ) |
Compute curve intersections
- Parameters
-
[in] pC1 first curve [in] offs_C1 offset of the first curve [in] pC2 second curve [in] offs_C2 offset of the second curve [out] ilist list of the intersection (as parameter on the curves)
◆ intersect_SAE()
|
inline |
Compute curve intersections
- Parameters
-
[in] pC1 first curve [in] offs_C1 offset of the first curve [in] pC2 second curve [in] offs_C2 offset of the second curve [out] ilist list of the intersection (as parameter on the curves)
◆ intersectCircleCircle()
integer G2lib::intersectCircleCircle | ( | real_type | x1, |
real_type | y1, | ||
real_type | theta1, | ||
real_type | kappa1, | ||
real_type | x2, | ||
real_type | y2, | ||
real_type | theta2, | ||
real_type | kappa2, | ||
real_type | s1[], | ||
real_type | s2[] ) |
Intersect the parametric arc
\[ x = x_1+\frac{\sin(\kappa_1 s+\theta_1)-sin(\theta_1)}{\kappa_1} \]
\[ y = y_1+\frac{\cos(\theta_1)-\cos(\kappa_1 s+\theta_1)}{\kappa_1} \]
with the parametric arc
\[ x = x_2+\frac{\sin(\kappa_2 s+\theta_2)-sin(\theta_2)}{\kappa_2} \]
\[ y = y_2+\frac{\cos(\theta_2)-\cos(\kappa_2 s+\theta_2)}{\kappa_2} \]
- Parameters
-
[in] x1 x-origin of the first arc [in] y1 y-origin of the first arc [in] theta1 initial angle of the first arc [in] kappa1 curvature of the first arc [in] x2 x-origin of the second arc [in] y2 y-origin of the second arc [in] theta2 initial angle of the second arc [in] kappa2 curvature of the second arc [out] s1 parameter2 of intersection for the first circle arc [out] s2 parameter2 of intersection for the second circle arc
- Returns
- the number of solution 0, 1 or 2
◆ is_counter_clockwise()
integer G2lib::is_counter_clockwise | ( | real_type const | P1[], |
real_type const | P2[], | ||
real_type const | P3[] ) |
Return the orientation of a triangle
- Parameters
-
[in] P1 first point of the triangle [in] P2 second point of the triangle [in] P3 third point of the triangle
- Returns
- sign of rotation
return +1 = CounterClockwise return -1 = Clockwise return 0 = flat
- CounterClockwise: the path P1->P2->P3 turns Counter-Clockwise, i.e., the point P3 is located "on the left" of the line P1-P2.
- Clockwise: the path turns Clockwise, i.e., the point P3 lies "on the right" of the line P1-P2.
- flat: the point P3 is located on the line segment [P1 P2].
Algorithm from FileExchage geom2d adapated from Sedgewick's book.
◆ is_point_in_triangle()
integer G2lib::is_point_in_triangle | ( | real_type const | point[], |
real_type const | P1[], | ||
real_type const | P2[], | ||
real_type const | P3[] ) |
Check if a point is inside a triangle
- Parameters
-
[in] point point to check if is inside the triangle [in] P1 first point of the triangle [in] P2 second point of the triangle [in] P3 third point of the triangle
- Returns
- {0,+1,-1} return +1 = Inside return -1 = Outsize return 0 = on border
◆ minmax3()
|
inline |
Return minumum and maximum of three numbers
◆ operator<<() [1/12]
|
inline |
◆ operator<<() [2/12]
ostream_type & G2lib::operator<< | ( | ostream_type & | stream, |
Biarc const & | bi ) |
◆ operator<<() [3/12]
ostream_type & G2lib::operator<< | ( | ostream_type & | stream, |
BiarcList const & | CL ) |
◆ operator<<() [4/12]
ostream_type & G2lib::operator<< | ( | ostream_type & | stream, |
CircleArc const & | c ) |
◆ operator<<() [5/12]
ostream_type & G2lib::operator<< | ( | ostream_type & | stream, |
ClothoidCurve const & | c ) |
Print on strem the ClothoidCurve
object
- Parameters
-
stream the output stream c an instance of ClothoidCurve
object
- Returns
- the output stream
◆ operator<<() [6/12]
ostream_type & G2lib::operator<< | ( | ostream_type & | stream, |
ClothoidList const & | CL ) |
Print on strem the ClothoidList
object
- Parameters
-
stream the output stream CL an instance of ClothoidList
object
- Returns
- the output stream
◆ operator<<() [7/12]
ostream_type & G2lib::operator<< | ( | ostream_type & | stream, |
ClothoidSplineG2 const & | c ) |
Print on strem the ClothoidSplineG2
object
- Parameters
-
stream the output stream c an instance of ClothoidSplineG2
object
- Returns
- the output stream
◆ operator<<() [8/12]
ostream_type & G2lib::operator<< | ( | ostream_type & | stream, |
Dubins const & | bi ) |
◆ operator<<() [9/12]
ostream_type & G2lib::operator<< | ( | ostream_type & | stream, |
Dubins3p const & | bi ) |
◆ operator<<() [10/12]
ostream_type & G2lib::operator<< | ( | ostream_type & | stream, |
LineSegment const & | c ) |
Print on strem the LineSegment
object
- Parameters
-
stream the output stream c an instance of LineSegment
object
- Returns
- the output stream
◆ operator<<() [11/12]
ostream_type & G2lib::operator<< | ( | ostream_type & | stream, |
PolyLine const & | P ) |
◆ operator<<() [12/12]
ostream_type & G2lib::operator<< | ( | ostream_type & | stream, |
Triangle2D const & | t ) |
Print on strem the Triangle2D
object
- Parameters
-
stream the output stream t an instance of Triangle2D
object
- Returns
- the output stream
◆ pointInsideCircle()
|
inline |
Check if point (qx,qy)
is inside the circle passing from (x0,y0)
with tangent direction (c0,s0)
and curvature k
- Parameters
-
[in] x0 starting \(x\)-coordinate of the circle arc [in] y0 starting \(y\)-coordinate of the circle arc [in] c0 \( \cos \theta \) [in] s0 \( \sin \theta \) [in] k Curvature of the circle [in] qx \( x \)-coordinate point to check [in] qy \( y \)-coordinate point to check
- Returns
- true if point is inside
◆ projectPointOnCircle()
real_type G2lib::projectPointOnCircle | ( | real_type | x0, |
real_type | y0, | ||
real_type | theta0, | ||
real_type | k, | ||
real_type | qx, | ||
real_type | qy ) |
Project point (qx,qy)
to the circle passing from (x0,y0)
with tangent direction (c0,s0)
and curvature k
- Parameters
-
[in] x0 x-starting point of circle arc [in] y0 y-starting point of circle arc [in] theta0 initial angle [in] k curvature [in] qx x-point to be projected [in] qy y-point to be projected
- Returns
- distance point circle
◆ projectPointOnCircleArc()
real_type G2lib::projectPointOnCircleArc | ( | real_type | x0, |
real_type | y0, | ||
real_type | c0, | ||
real_type | s0, | ||
real_type | k, | ||
real_type | L, | ||
real_type | qx, | ||
real_type | qy ) |
Project point (qx,qy)
to the circle arc passing from (x0,y0)
with tangent direction (c0,s0)
curvature k
length L
- Parameters
-
[in] x0 \( x \)-starting point of circle arc [in] y0 \( y \)-starting point of circle arc [in] c0 \( \cos \theta_0 \) [in] s0 \( \sin \theta_0 \) [in] k curvature [in] L arc length [in] qx \( x \)-point to be projected [in] qy \( y \)-point to be projected
- Returns
- distance point circle
◆ rangeSymm()
void G2lib::rangeSymm | ( | real_type & | ang | ) |
Add or remove multiple of \( 2\pi \) to an angle in order to put it in the range \( [-\pi,\pi]\).
◆ solveLinearQuadratic()
integer G2lib::solveLinearQuadratic | ( | real_type | A, |
real_type | B, | ||
real_type | C, | ||
real_type | a, | ||
real_type | b, | ||
real_type | c, | ||
real_type | x[], | ||
real_type | y[] ) |
Solve the nonlinear system
\[ A x + B y = C \]
\[ a x^2 + b y^2 = c \]
- Parameters
-
[in] A first parameter of the linear equation [in] B second parameter of the linear equation [in] C third parameter of the linear equation [in] a first parameter of the quadratic equation [in] b second parameter of the quadratic equation [in] c third parameter of the quadratic equation [out] x \(x\)-coordinates of the solutions [out] y \(y\)-coordinates of the solutions
- Returns
- the number of solution 0, 1 or 2
◆ solveLinearQuadratic2()
integer G2lib::solveLinearQuadratic2 | ( | real_type | A, |
real_type | B, | ||
real_type | C, | ||
real_type | x[], | ||
real_type | y[] ) |
Solve the nonlinear system
\[ A x + B y = C \]
\[ x^2 + y^2 = 1 \]
- Parameters
-
[in] A first parameter of the linear equation [in] B second parameter of the linear equation [in] C third parameter of the linear equation [out] x \( x \)-coordinates of the solutions [out] y \( y \)-coordinates of the solutions
- Returns
- the number of solution 0, 1 or 2
◆ string_to_Dubins3pBuildType()
Dubins3pBuildType G2lib::string_to_Dubins3pBuildType | ( | string const & | str | ) |
- Parameters
-
[in] str name of the Dubins3pBuildType
type
- Returns
- the
Dubins3pBuildType
enumerator
◆ to_integer()
integer G2lib::to_integer | ( | DubinsType | d | ) |
◆ to_string() [1/2]
|
inline |
Convert curve type to a string
◆ to_string() [2/2]
|
inline |
- Parameters
-
[in] n Dubins type solution
- Returns
- the string with the name of the solution
◆ xy_to_guess_angle()
void G2lib::xy_to_guess_angle | ( | integer | npts, |
real_type const | x[], | ||
real_type const | y[], | ||
real_type | theta[], | ||
real_type | theta_min[], | ||
real_type | theta_max[], | ||
real_type | omega[], | ||
real_type | len[] ) |
Given a list of \( n \) points \( (x_i,y_i) \) compute the guess angles for the \( G^2 \) curve construction.
- Parameters
-
[in] npts \(n\) [in] x \(x\)-coordinates of the points [in] y \(y\)-coordinates of the points [out] theta guess angles [out] theta_min minimum angles at each nodes [out] theta_max maximum angles at each nodes [out] omega angles of two consecutive points, with accumulated \( 2\pi \) angle rotation [out] len distance between two consecutive poijts
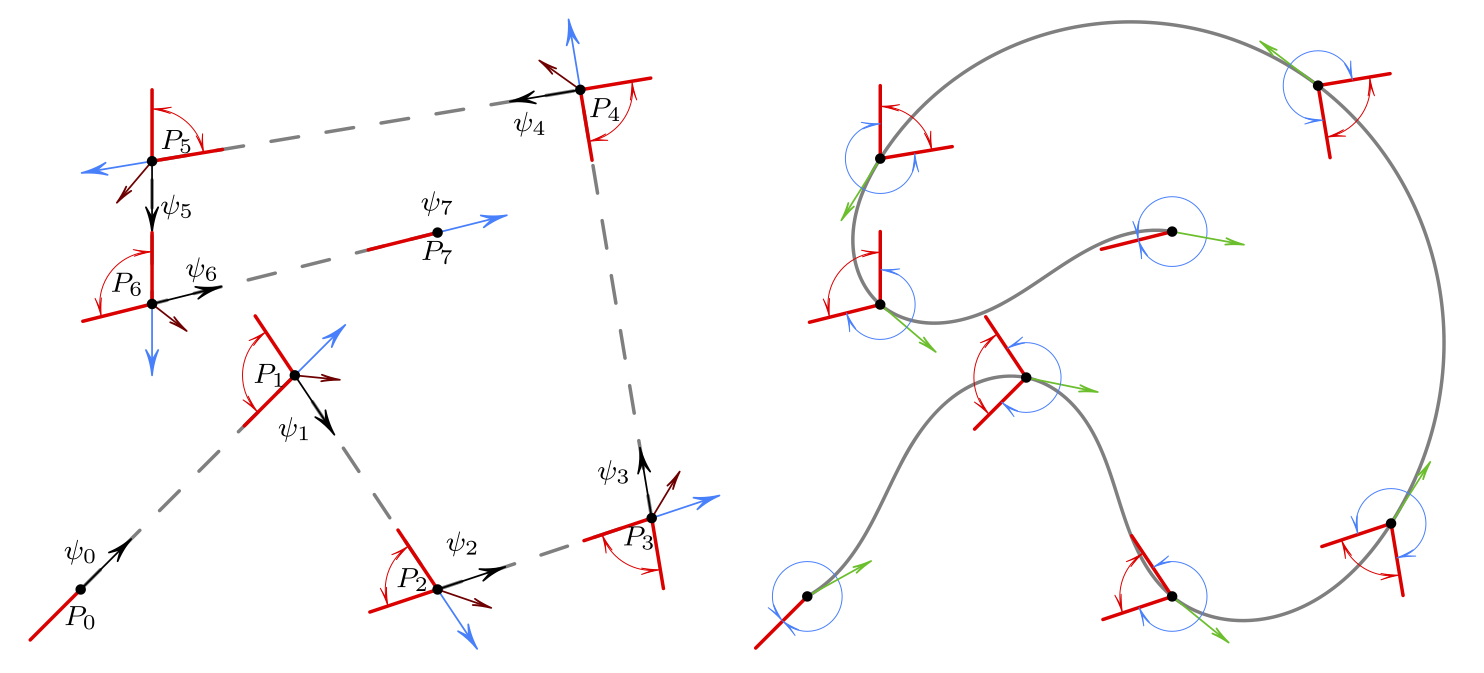
Generated by