ClothoidList Class Reference
Clothoids
|
#include <ClothoidList.hxx>
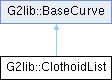
Friends | |
ostream_type & | operator<< (ostream_type &stream, ClothoidList const &CL) |
Detailed Description
Manage a piecewise clothoids \( G(s) \) composed by \( n \) clothoids (not necessarily \( G^2 \) or \( G^1 \) connected)
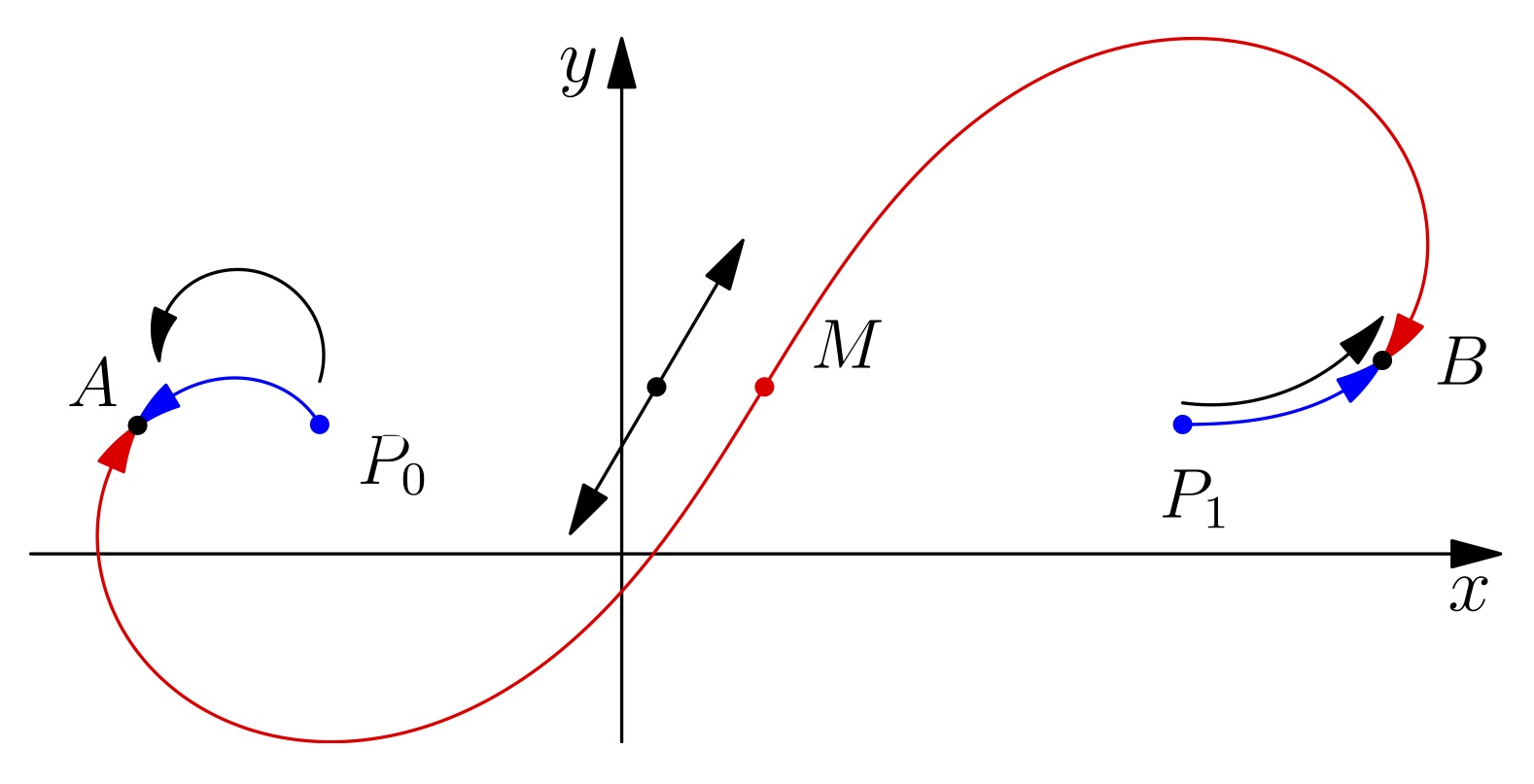
Constructor & Destructor Documentation
◆ ClothoidList() [1/13]
|
delete |
eof: BaseCurve_using.hxx
◆ ClothoidList() [2/13]
|
inline |
Build an empty clothoid list
◆ ClothoidList() [3/13]
|
inline |
Build a copy of an existing clothoid list
◆ ClothoidList() [4/13]
|
explicit |
Build a clothoid from a line segment
◆ ClothoidList() [5/13]
|
explicit |
Build a clothoid from a circle arc
◆ ClothoidList() [6/13]
|
explicit |
Build a clothoid from a biarc
◆ ClothoidList() [7/13]
|
explicit |
Build a clothoid from a list of biarc
◆ ClothoidList() [8/13]
|
explicit |
Build a clothoid from a clothoid curve
◆ ClothoidList() [9/13]
|
explicit |
Build a clothoid from a list line segment
◆ ClothoidList() [10/13]
|
explicit |
Build a clothoid from G2solve2arc
◆ ClothoidList() [11/13]
|
explicit |
Build a clothoid from G2solve3arc
◆ ClothoidList() [12/13]
|
explicit |
Build a clothoid from G2solveCLC
◆ ClothoidList() [13/13]
|
explicit |
Build a clothoid from a curve
Member Function Documentation
◆ bb_triangles()
|
overridevirtual |
Build a cover with triangles of the curve.
- Parameters
-
[out] tvec list of covering triangles [out] max_angle maximum angle variation of the curve covered by a triangle [out] max_size maximum admissible size of the covering tirnagles [out] icurve index of the covering triangles
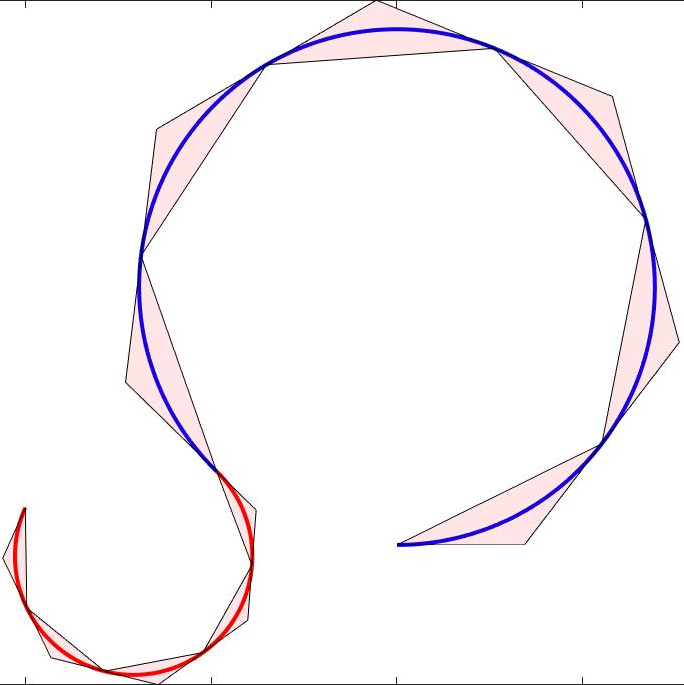
Implements G2lib::BaseCurve.
◆ bb_triangles_ISO()
|
overridevirtual |
Build a cover with triangles of the curve with offset (ISO).
- Parameters
-
[out] offs curve offset [out] tvec list of covering triangles [out] max_angle maximum angle variation of the curve covered by a triangle [out] max_size maximum admissible size of the covering tirnagles [out] icurve index of the covering triangles
Implements G2lib::BaseCurve.
◆ bb_triangles_SAE()
|
inlineoverridevirtual |
Build a cover with triangles of the curve with offset (SAE).
- Parameters
-
[out] offs curve offset [out] tvec list of covering triangles [out] max_angle maximum angle variation of the arc covered by a triangle [out] max_size maximum admissible size of the covering tirnagles [out] icurve index of the covering triangles
Implements G2lib::BaseCurve.
◆ bbox()
|
inlineoverridevirtual |
Compute the bounding box of the curve.
- Parameters
-
[out] xmin left bottom [out] ymin left bottom [out] xmax right top [out] ymax right top
Implements G2lib::BaseCurve.
◆ bbox_ISO()
|
overridevirtual |
Compute the bounding box of the curve with offset (ISO).
- Parameters
-
[in] offs curve offset [out] xmin left bottom [out] ymin left bottom [out] xmax right top [out] ymax right top
Implements G2lib::BaseCurve.
◆ build() [1/2]
bool G2lib::ClothoidList::build | ( | real_type | x0, |
real_type | y0, | ||
real_type | theta0, | ||
integer | n, | ||
real_type const | s[], | ||
real_type const | kappa[] ) |
Build clothoid list with \( G^2 \) continuity. The vector s
contains the breakpoints of the curve. Between two breakpoint the curvature change linearly (is a clothoid)
- Parameters
-
[in] x0 initial x [in] y0 initial y [in] theta0 initial angle [in] n number of segments [in] s break point of the piecewise curve [in] kappa curvature at the break point
- Returns
- true if curve is closed
◆ build() [2/2]
|
inline |
Build clothoid list with \( G^2 \) continuity. The vector s
contains the breakpoints of the curve. Between two breakpoint the curvature change linearly (is a clothoid)
- Parameters
-
[in] x0 initial x [in] y0 initial y [in] theta0 initial angle [in] s break point of the piecewise curve [in] kappa curvature at the break point
- Returns
- true if curve is closed
◆ build_G1() [1/2]
Build clothoid list passing to a list of points solving a series of \( G^1 \) fitting problems. The angle at points are estimated using the routine xy_to_guess_angle
- Parameters
-
[in] n number of points [in] x \(x\)-coordinates [in] y \(y\)-coordinates
- Returns
- false if routine fails
◆ build_G1() [2/2]
bool G2lib::ClothoidList::build_G1 | ( | integer | n, |
real_type const | x[], | ||
real_type const | y[], | ||
real_type const | theta[] ) |
Build clothoid list passing to a list of points solving a series of \( G^1 \) fitting problems.
- Parameters
-
[in] n number of points [in] x \(x\)-coordinates [in] y \(y\)-coordinates [in] theta angles at the points
- Returns
- false if routine fails
◆ build_raw() [1/2]
bool G2lib::ClothoidList::build_raw | ( | integer | n, |
real_type const | x[], | ||
real_type const | y[], | ||
real_type const | abscissa[], | ||
real_type const | theta[], | ||
real_type const | kappa[] ) |
Build clothoid listy using raw data.
- Parameters
-
[in] n number of points [in] x \(x\)-coordinates [in] y \(y\)-coordinates [in] abscissa break point of the piecewise curve [in] theta angles at breakpoints [in] kappa curvature at the break point
- Returns
- false if fails
◆ build_raw() [2/2]
|
inline |
Build clothoid listy using raw data.
- Parameters
-
[in] x \(x\)-coordinates [in] y \(y\)-coordinates [in] abscissa break point of the piecewise curve [in] theta angles at breakpoints [in] kappa curvature at the break point
- Returns
- false if fails
◆ change_origin()
Translate curve so that origin will be (newx0
, newy0
).
Implements G2lib::BaseCurve.
◆ closest_point_by_sample()
real_type G2lib::ClothoidList::closest_point_by_sample | ( | real_type | ds, |
real_type | qx, | ||
real_type | qy, | ||
real_type & | X, | ||
real_type & | Y, | ||
real_type & | S ) const |
Compute the point on clothoid at minimal distance from a given point using the optimized algorithm described in the publication:
- Parameters
-
ds sampling step qx \(x\)-coordinate of the given point qy \(y\)-coordinate of the given point X \(x\)-coordinate of the point on clothoid at minimal distance Y \(y\)-coordinate of the point on clothoid at minimal distance S curvilinear coordinate of the point (X,Y) on the clothoid
- Returns
- the distance of the point from the clothoid
◆ closest_point_in_range_ISO()
integer G2lib::ClothoidList::closest_point_in_range_ISO | ( | real_type | qx, |
real_type | qy, | ||
integer | icurve_begin, | ||
integer | icurve_end, | ||
real_type & | x, | ||
real_type & | y, | ||
real_type & | s, | ||
real_type & | t, | ||
real_type & | dst, | ||
integer & | icurve ) const |
- Parameters
-
qx \(x\)-coordinate of the point qy \(y\)-coordinate of the point icurve_begin index of the initial segment icurve_end index of the past to the last segment x \(x\)-coordinate of the projected point on the curve y \(y\)-coordinate of the projected point on the curve s parameter on the curve of the projection t curvilinear coordinate of the point x,y (if orthogonal projection) dst distance point projected point icurve number of the segment with the projected point
- Returns
- 1 point is projected orthogonal
0 = more than one projection (first returned)
-1 = minimum point is not othogonal projection to curve
◆ closest_point_in_range_SAE()
|
inline |
- Parameters
-
qx \(x\)-coordinate of the point qy \(y\)-coordinate of the point icurve_begin index of the initial segment icurve_end index of the past to the last segment x \(x\)-coordinate of the projected point on the curve y \(y\)-coordinate of the projected point on the curve s parameter on the curve of the projection t curvilinear coordinate of the point x,y (if orthogonal projection) dst distance point projected point icurve number of the segment with the projected point
- Returns
- 1 point is projected orthogonal
0 = more than one projection (first returned)
-1 = minimum point is not othogonal projection to curve
◆ closest_point_in_s_range_ISO()
integer G2lib::ClothoidList::closest_point_in_s_range_ISO | ( | real_type | qx, |
real_type | qy, | ||
real_type | s_begin, | ||
real_type | s_end, | ||
real_type & | x, | ||
real_type & | y, | ||
real_type & | s, | ||
real_type & | t, | ||
real_type & | dst, | ||
integer & | icurve ) const |
- Parameters
-
qx \(x\)-coordinate of the point qy \(y\)-coordinate of the point s_begin initial curvilinear coordinate of the search range s_end final curvilinear coordinate of the search range x \(x\)-coordinate of the projected point on the curve y \(y\)-coordinate of the projected point on the curve s parameter on the curve of the projection t curvilinear coordinate of the point x,y (if orthogonal projection) dst distance point projected point icurve number of the segment with the projected point
- Returns
- 1 ok -1 projection failed
◆ closest_point_in_s_range_SAE()
|
inline |
- Parameters
-
qx \(x\)-coordinate of the point qy \(y\)-coordinate of the point s_begin initial curvilinear coordinate of the search range s_end final curvilinear coordinate of the search range x \(x\)-coordinate of the projected point on the curve y \(y\)-coordinate of the projected point on the curve s parameter on the curve of the projection t curvilinear coordinate of the point x,y (if orthogonal projection) dst distance point projected point icurve number of the segment with the projected point
- Returns
- 1 ok -1 projection failed
◆ closest_point_ISO() [1/2]
|
overridevirtual |
- Parameters
-
qx \(x\)-coordinate of the point qy \(y\)-coordinate of the point x \(x\)-coordinate of the projected point on the curve y \(y\)-coordinate of the projected point on the curve s parameter on the curve of the projection t curvilinear coordinate of the point x,y (if orthogonal projection) dst distance point projected point
- Returns
- n >= 0 point is projected orthogonal, n is the number of the segment at minimum distance
-(n+1) minimum point is not othogonal projection to curve
Implements G2lib::BaseCurve.
◆ closest_point_ISO() [2/2]
|
overridevirtual |
- Parameters
-
qx \(x\)-coordinate of the point qy \(y\)-coordinate of the point offs offset of the curve x \(x\)-coordinate of the projected point on the curve y \(y\)-coordinate of the projected point on the curve s parameter on the curve of the projection t curvilinear coordinate of the point x,y (if orthogonal projection) dst distance point projected point
- Returns
- n > 0 point is projected orthogonal, n-1 is the number of the segment at minimum distance
-(n+1) minimum point is not othogonal projection to curve
Implements G2lib::BaseCurve.
◆ closest_point_SAE() [1/2]
|
inline |
Given a point find closest point on the curve.
- Parameters
-
qx \(x\)-coordinate of the point qy \(y\)-coordinate of the point x \(x\)-coordinate of the projected point on the curve y \(y\)-coordinate of the projected point on the curve s parameter on the curve of the projection t curvilinear coordinate of the point x,y (if orthogonal projection) dst distance point projected point
- Returns
- 1 = point is projected orthogonal 0 = more than one projection (first returned) -1 = minimum point is not othogonal projection to curve
◆ closest_point_SAE() [2/2]
|
inline |
Given a point find closest point on the curve.
- Parameters
-
qx \(x\)-coordinate of the point qy \(y\)-coordinate of the point offs offset of the curve x \(x\)-coordinate of the projected point on the curve y \(y\)-coordinate of the projected point on the curve s parameter on the curve of the projection t curvilinear coordinate of the point x,y (if orthogonal projection) dst distance point projected point
- Returns
- 1 = point is projected orthogonal 0 = more than one projection (first returned) -1 = minimum point is not othogonal projection to curve
◆ closest_segment()
- Parameters
-
qx \(x\)-coordinate of the point qy \(y\)-coordinate of the point
- Returns
- the segment at minimal distance from point (qx,qy)
◆ closure_check()
|
inline |
check if clothoid list is closed
- Parameters
-
[in] tol_xy position tolerance [in] tol_tg angle (tangent) tolerance
- Returns
- true if curve is closed
◆ closure_gap_tx()
|
inline |
Difference initial final tangent x component
◆ closure_gap_ty()
|
inline |
Difference initial final tangent y component
◆ closure_gap_x()
|
inline |
Difference initial final point x component
◆ closure_gap_y()
|
inline |
Difference initial final point y component
◆ collision() [1/2]
|
overridevirtual |
Check collision with another curve.
Implements G2lib::BaseCurve.
◆ collision() [2/2]
|
inline |
Detect a collision with another clothoid list
◆ collision_ISO() [1/2]
|
overridevirtual |
Check collision with another curve with offset (ISO).
- Parameters
-
[in] offs curve offset [in] pC second curve to check collision [in] offs_C curve offset of the second curve
- Returns
- true if collision is detected
Implements G2lib::BaseCurve.
◆ collision_ISO() [2/2]
bool G2lib::ClothoidList::collision_ISO | ( | real_type | offs, |
ClothoidList const & | CL, | ||
real_type | offs_C ) const |
Detect a collision with another clothoid list with offset
- Parameters
-
[in] offs offset of first clothoid list [in] CL second clothoid list [in] offs_C offset of second clothoid list
◆ copy()
void G2lib::ClothoidList::copy | ( | ClothoidList const & | L | ) |
Build a clothoid list copying an existing one
◆ distance_ISO()
|
inline |
Compute the distance between a point \(q=(q_x,q_y)\) and the curve with offset (ISO).
- Parameters
-
[in] qx component \(q_x\) [in] qy component \(q_y\) [in] offs offset of the curve
- Returns
- the computed distance
◆ distance_SAE()
|
inline |
Compute the distance between a point \(q=(q_x,q_y)\) and the curve with offset (SAE).
- Parameters
-
[in] qx component \(q_x\) [in] qy component \(q_y\) [in] offs offset of the curve
- Returns
- the computed distance
◆ eval()
x and \(y\)-coordinate at curvilinear coordinate \(s\).
Implements G2lib::BaseCurve.
◆ eval_D()
|
overridevirtual |
x and \(y\)-coordinate derivative at curvilinear coordinate \(s\).
Implements G2lib::BaseCurve.
◆ eval_DD()
|
overridevirtual |
x and \(y\)-coordinate second derivative at curvilinear coordinate \(s\).
Implements G2lib::BaseCurve.
◆ eval_DDD()
|
overridevirtual |
x and \(y\)-coordinate third derivative at curvilinear coordinate \(s\).
Implements G2lib::BaseCurve.
◆ eval_ISO()
|
overridevirtual |
Compute curve at position s
with offset offs
(ISO).
- Parameters
-
[in] s parameter on the curve [in] offs offset of the curve [out] x coordinate [out] y coordinate
Reimplemented from G2lib::BaseCurve.
◆ eval_ISO_D()
|
overridevirtual |
Compute derivative curve at position s
with offset offs
(ISO).
- Parameters
-
[in] s parameter on the curve [in] offs offset of the curve [out] x_D \(x\)-coordinate [out] y_D \(y\)-coordinate
Reimplemented from G2lib::BaseCurve.
◆ eval_ISO_DD()
|
overridevirtual |
Compute second derivative curve at position s
with offset offs
(ISO).
- Parameters
-
[in] s parameter on the curve [in] offs offset of the curve [out] x_DD \(x\)-coordinate second derivative [out] y_DD \(y\)-coordinate second derivative
Reimplemented from G2lib::BaseCurve.
◆ eval_ISO_DDD()
|
overridevirtual |
Compute third derivative curve at position s
with offset offs
(ISO).
- Parameters
-
[in] s parameter on the curve [in] offs offset of the curve [out] x_DDD \(x\)-coordinate third derivative [out] y_DDD \(y\)-coordinate third derivative
Reimplemented from G2lib::BaseCurve.
◆ eval_SAE()
|
inline |
Compute curve at position s
with offset offs
(SAE).
- Parameters
-
[in] s parameter on the curve [in] offs offset of the curve [out] x coordinate [out] y coordinate
◆ eval_SAE_D()
|
inline |
Compute derivative curve at position s
with offset offs
(SAE).
- Parameters
-
[in] s parameter on the curve [in] offs offset of the curve [out] x_D \(x\)-coordinate first derivative [out] y_D \(y\)-coordinate first derivative
◆ eval_SAE_DD()
|
inline |
Compute second derivative curve at position s
with offset offs
(SAE).
- Parameters
-
[in] s parameter on the curve [in] offs offset of the curve [out] x_DD \(x\)-coordinate second derivative [out] y_DD \(y\)-coordinate second derivative
◆ eval_SAE_DDD()
|
inline |
Compute third derivative curve at position s
with offset offs
(SAE).
- Parameters
-
[in] s parameter on the curve [in] offs offset of the curve [out] x_DDD \(x\)-coordinate third derivative [out] y_DDD \(y\)-coordinate third derivative
◆ evaluate()
|
overridevirtual |
Evaluate curve at curvilinear coordinate \(s\).
- Parameters
-
[in] s curvilinear coordinate [out] th angle [out] k curvature [out] x \(x\)-coordinate [out] y \(y\)-coordinate
Reimplemented from G2lib::BaseCurve.
◆ evaluate_ISO()
|
overridevirtual |
Evaluate curve with offset at curvilinear coordinate \(s\) (ISO).
- Parameters
-
[in] s curvilinear coordinate [in] offs offset [out] th angle [out] k curvature [out] x \(x\)-coordinate [out] y \(y\)-coordinate
Reimplemented from G2lib::BaseCurve.
◆ evaluate_SAE()
|
inlinevirtual |
Evaluate curve with offset at curvilinear coordinate \(s\) (SAE).
- Parameters
-
[in] s curvilinear coordinate [in] offs offset [out] th angle [out] k curvature [out] x \(x\)-coordinate [out] y \(y\)-coordinate
Reimplemented from G2lib::BaseCurve.
◆ export_ruby()
void G2lib::ClothoidList::export_ruby | ( | ostream_type & | stream | ) | const |
Save Clothoid list to a stream
- Parameters
-
stream streamstream to save
◆ export_table()
void G2lib::ClothoidList::export_table | ( | ostream_type & | stream | ) | const |
Save Clothoid list to a stream
- Parameters
-
stream stream to save
◆ find_at_s()
Find the clothoid segment whose definiton range contains s
◆ findST1() [1/2]
integer G2lib::ClothoidList::findST1 | ( | integer | ibegin, |
integer | iend, | ||
real_type | x, | ||
real_type | y, | ||
real_type & | s, | ||
real_type & | t ) const |
Find parametric coordinate.
- Parameters
-
ibegin initial segment to compute the distance iend final segment to compute the distance x \(x\)-coordinate point y \(y\)-coordinate point s value \( s \) t value \( t \)
- Returns
- idx the segment with point at minimal distance, otherwise
-(idx+1)
if \( (x,y) \) cannot be projected orthogonally on the segment
◆ findST1() [2/2]
integer G2lib::ClothoidList::findST1 | ( | real_type | x, |
real_type | y, | ||
real_type & | s, | ||
real_type & | t ) const |
Find parametric coordinate.
- Parameters
-
x \(x\)-coordinate point y \(y\)-coordinate point s value \( s \) t value \( t \)
- Returns
- idx the segment with point at minimal distance, otherwise -(idx+1) if \( (x,y) \) cannot be projected orthogonally on the segment
◆ get()
ClothoidCurve const & G2lib::ClothoidList::get | ( | integer | idx | ) | const |
Get the idx
-th clothoid of the list
◆ get_at_s()
ClothoidCurve const & G2lib::ClothoidList::get_at_s | ( | real_type | s | ) | const |
Get the idx
-th clothoid of the list where idx
is the clothoid at parameter s
◆ get_SK() [1/2]
Return the clothoid list as a list of nodes and curvatures
- Parameters
-
[out] s nodes [out] kappa curvature
◆ get_SK() [2/2]
|
inline |
Return the clothoid list as a list of nodes and curvatures
- Parameters
-
[out] s nodes [out] kappa curvature
◆ get_STK() [1/2]
Return the clothoid list as a list of nodes angles and curvatures
- Parameters
-
[out] s nodes [out] theta angles [out] kappa curvature
◆ get_STK() [2/2]
|
inline |
Return the clothoid list as a list of nodes angles and curvatures
- Parameters
-
[out] s nodes [out] theta angles [out] kappa curvature
◆ get_XY()
Return the points of the clothoid list at breakpoints
- Parameters
-
[out] x \(x\)-coordinates [out] y \(y\)-coordinates
◆ info()
|
inlineoverridevirtual |
Pretty print of the curve data.
Implements G2lib::BaseCurve.
◆ init()
void G2lib::ClothoidList::init | ( | ) |
Initialize the clothoid list
◆ intersect() [1/2]
|
overridevirtual |
Intersect the curve with another curve.
- Parameters
-
[in] pC second curve intersect [out] ilist list of the intersection (as parameter on the curves)
Implements G2lib::BaseCurve.
◆ intersect() [2/2]
|
inline |
Intersect a clothoid list with another clothoid list
- Parameters
-
[in] CL second clothoid list [out] ilist list of the intersection (as parameter on the curves)
◆ intersect_ISO() [1/2]
|
overridevirtual |
Intersect the curve with another curve with offset (ISO)
- Parameters
-
[in] offs offset first curve [in] pC second curve intersect [in] offs_C offset second curve [out] ilist list of the intersection (as parameter on the curves)
Implements G2lib::BaseCurve.
◆ intersect_ISO() [2/2]
void G2lib::ClothoidList::intersect_ISO | ( | real_type | offs, |
ClothoidList const & | CL, | ||
real_type | offs_obj, | ||
IntersectList & | ilist ) const |
Intersect a clothoid list with another clothoid list with offset (ISO)
- Parameters
-
[in] offs offset of first clothoid list [in] CL second clothoid list [in] offs_obj offset of second clothoid list [out] ilist list of the intersection (as parameter on the curves)
◆ is_closed()
|
inline |
True if curve is closed
◆ length()
|
overridevirtual |
The length of the curve
Implements G2lib::BaseCurve.
◆ length_ISO()
The length of the curve with offset (ISO)
Implements G2lib::BaseCurve.
◆ load()
void G2lib::ClothoidList::load | ( | istream_type & | stream, |
real_type | epsi = 1e-8 ) |
Read the clothoid list from a stream. The data is assumed to be saved as follows
# x y theta kappa x0 y0 theta0 kappa0 x1 y1 theta1 kappa1 ... xn yn thetan kappan
◆ make_closed()
|
inline |
Set clousure flag to true
◆ make_open()
|
inline |
Set clousure flag to false
◆ num_segments()
|
inline |
Return the numbber of clothoid of the list
◆ nx_begin_ISO()
|
inlineoverridevirtual |
Intial normal \(x\)-coordinate (ISO).
Reimplemented from G2lib::BaseCurve.
◆ nx_begin_SAE()
|
inline |
Intial normal \(x\)-coordinate (SAE).
◆ nx_end_ISO()
|
inlineoverridevirtual |
Final normal \(x\)-coordinate (ISO).
Reimplemented from G2lib::BaseCurve.
◆ nx_end_SAE()
|
inline |
Final normal \(x\)-coordinate (SAE).
◆ ny_begin_ISO()
|
inlineoverridevirtual |
Intial normal \(y\)-coordinate (ISO).
Reimplemented from G2lib::BaseCurve.
◆ ny_begin_SAE()
|
inline |
Intial normal \(y\)-coordinate (SAE).
◆ ny_end_ISO()
|
inlineoverridevirtual |
Final normal \(y\)-coordinate (ISO).
Reimplemented from G2lib::BaseCurve.
◆ ny_end_SAE()
|
inline |
Intial normal \(y\)-coordinate (SAE).
◆ operator=()
|
inline |
Copy an existing clothoid list
◆ push_back() [1/14]
void G2lib::ClothoidList::push_back | ( | Biarc const & | c | ) |
Add a biarc to the tail of clothoid list
◆ push_back() [2/14]
void G2lib::ClothoidList::push_back | ( | BiarcList const & | c | ) |
Add a biarc list to the tail of clothoid list
◆ push_back() [3/14]
void G2lib::ClothoidList::push_back | ( | CircleArc const & | c | ) |
Add a circle arc to the tail of clothoid list
◆ push_back() [4/14]
void G2lib::ClothoidList::push_back | ( | ClothoidCurve const & | c | ) |
Add a clothoid curve to the tail of clothoid list
◆ push_back() [5/14]
void G2lib::ClothoidList::push_back | ( | ClothoidList const & | c | ) |
Add a clothoid list to the tail of clothoid list
◆ push_back() [6/14]
void G2lib::ClothoidList::push_back | ( | Dubins const & | c | ) |
Add a dubins 3 arc curve to the tail of clothoid list
◆ push_back() [7/14]
void G2lib::ClothoidList::push_back | ( | Dubins3p const & | c | ) |
Add a dubins 6 arc curve to the tail of clothoid list
◆ push_back() [8/14]
void G2lib::ClothoidList::push_back | ( | G2solve2arc const & | c | ) |
Add a G2solve2arc to the tail of clothoid list
◆ push_back() [9/14]
void G2lib::ClothoidList::push_back | ( | G2solve3arc const & | c | ) |
Add a G2solve3arc list to the tail of clothoid list
◆ push_back() [10/14]
void G2lib::ClothoidList::push_back | ( | G2solveCLC const & | c | ) |
Add a clothoid list to the tail of clothoid list
◆ push_back() [11/14]
void G2lib::ClothoidList::push_back | ( | LineSegment const & | c | ) |
Add a line segment to the tail of clothoid list
◆ push_back() [12/14]
void G2lib::ClothoidList::push_back | ( | PolyLine const & | c | ) |
Add a list of line segment to the tail of clothoid list
◆ push_back() [13/14]
Add a clothoid to the tail of the clothoid list.
- Parameters
-
kappa0 initial curvature dkappa derivative of the curvature L length of the segment
◆ push_back() [14/14]
void G2lib::ClothoidList::push_back | ( | real_type | x0, |
real_type | y0, | ||
real_type | theta0, | ||
real_type | kappa0, | ||
real_type | dkappa, | ||
real_type | L ) |
Add a clothoid to the tail of the clothoid list. The builded clothoid is translated to the tail of the clothioid list.
- Parameters
-
x0 initial x y0 initial y theta0 initial angle kappa0 initial curvature dkappa derivative of the curvature L length of the segment
◆ push_back_G1() [1/2]
void G2lib::ClothoidList::push_back_G1 | ( | real_type | x0, |
real_type | y0, | ||
real_type | theta0, | ||
real_type | x1, | ||
real_type | y1, | ||
real_type | theta1 ) |
Add a clothoid to the tail of the clothoid list solving the \( G^1 \) problem. The initial point and angle are taken from the tail of the clothoid list. The builded clothoid is translated to the tail of the clothioid list.
- Parameters
-
x0 initial x y0 initial y theta0 initial angle x1 final x y1 final y theta1 final angle
◆ push_back_G1() [2/2]
Add a clothoid to the tail of the clothoid list solving the \( G^1 \) problem. The initial point and angle are taken from the tail of the clothoid list.
- Parameters
-
x1 final x y1 final y theta1 final angle
◆ reserve()
void G2lib::ClothoidList::reserve | ( | integer | n | ) |
Reserve memory for n
clothoid
◆ reverse()
|
overridevirtual |
Reverse curve parameterization.
Implements G2lib::BaseCurve.
◆ rotate()
Rotate curve by angle \(\theta\) centered at point \((c_x,c_y)\).
- Parameters
-
[in] angle angle \(\theta\) [in] cx \(c_x\) [in] cy \(c_y\)
Implements G2lib::BaseCurve.
◆ save()
void G2lib::ClothoidList::save | ( | ostream_type & | stream | ) | const |
Save the clothoid list on a stream. The data is saved as follows
# x y theta kappa x0 y0 theta0 kappa0 x1 y1 theta1 kappa1 ... xn yn thetan kappan
◆ scale()
|
overridevirtual |
Scale curve by factor sc
.
Implements G2lib::BaseCurve.
◆ segment_length()
Return the length of the nseg
-th clothoid of the list
◆ segment_length_ISO()
Return the length of the nseg
-th clothoid of the list with offset
◆ segment_length_SAE()
Return the length of the nseg
-th clothoid of the list with offset
◆ setup()
|
overridevirtual |
Implements G2lib::BaseCurve.
◆ tg()
|
overridevirtual |
Tangent at curvilinear coordinate \(s\).
Reimplemented from G2lib::BaseCurve.
◆ tg_D()
|
overridevirtual |
Tangent derivative at curvilinear coordinate \(s\).
Reimplemented from G2lib::BaseCurve.
◆ tg_DD()
|
overridevirtual |
Tangent second derivative at curvilinear coordinate \(s\).
Reimplemented from G2lib::BaseCurve.
◆ tg_DDD()
|
overridevirtual |
Tangent third derivative at curvilinear coordinate \(s\).
Reimplemented from G2lib::BaseCurve.
◆ theta()
Angle at curvilinear coordinate \(s\).
Implements G2lib::BaseCurve.
◆ theta_begin()
|
inlineoverridevirtual |
Initial angle of the curve.
Reimplemented from G2lib::BaseCurve.
◆ theta_D()
Angle derivative (curvature) at curvilinear coordinate \(s\).
Implements G2lib::BaseCurve.
◆ theta_DD()
Angle second derivative (devitive of curvature) at curvilinear coordinate \(s\).
Implements G2lib::BaseCurve.
◆ theta_DDD()
Angle third derivative at curvilinear coordinate \(s\).
Implements G2lib::BaseCurve.
◆ theta_end()
|
inlineoverridevirtual |
Final angle of the curve.
Reimplemented from G2lib::BaseCurve.
◆ translate()
translate curve by \((t_x,t_y)\)
Implements G2lib::BaseCurve.
◆ trim()
Cut curve at parametric coordinate s_begin
and s_end
.
Implements G2lib::BaseCurve.
◆ tx()
Tangent \(x\)-coordinate at curvilinear coordinate \(s\).
Reimplemented from G2lib::BaseCurve.
◆ tx_begin()
|
inlineoverridevirtual |
Initial tangent \(x\)-coordinate.
Reimplemented from G2lib::BaseCurve.
◆ tx_D()
Tangent derivative \(x\)-coordinate at curvilinear coordinate \(s\).
Reimplemented from G2lib::BaseCurve.
◆ tx_DD()
Tangent second derivative \(x\)-coordinate at curvilinear coordinate \(s\).
Reimplemented from G2lib::BaseCurve.
◆ tx_DDD()
Tangent third derivative \(x\)-coordinate at curvilinear coordinate \(s\).
Reimplemented from G2lib::BaseCurve.
◆ tx_end()
|
inlineoverridevirtual |
Final tangent \(x\)-coordinate.
Reimplemented from G2lib::BaseCurve.
◆ ty()
Tangent \(y\)-coordinate at curvilinear coordinate \(s\).
Reimplemented from G2lib::BaseCurve.
◆ ty_begin()
|
inlineoverridevirtual |
Initial tangent \(y\)-coordinate.
Reimplemented from G2lib::BaseCurve.
◆ ty_D()
Tangent derivative \(y\)-coordinate at curvilinear coordinate \(s\).
Reimplemented from G2lib::BaseCurve.
◆ ty_DD()
Tangent second derivative \(y\)-coordinate at curvilinear coordinate \(s\).
Reimplemented from G2lib::BaseCurve.
◆ ty_DDD()
Tangent third derivative \(y\)-coordinate at curvilinear coordinate \(s\).
Reimplemented from G2lib::BaseCurve.
◆ ty_end()
|
inlineoverridevirtual |
Final tangent \(y\)-coordinate.
Reimplemented from G2lib::BaseCurve.
◆ type()
|
inlineoverridevirtual |
The name of the curve type
Implements G2lib::BaseCurve.
◆ wrap_in_range()
void G2lib::ClothoidList::wrap_in_range | ( | real_type & | s | ) | const |
The list of clothoid has total length \( L \) the parameter \( s \) us recomputed as \( s+kL \) in such a way \( s+kL\in[0,L) \) with \( k\in\mathbb{Z} \).
◆ X()
\(x\)-coordinate at curvilinear coordinate \(s\).
Implements G2lib::BaseCurve.
◆ x_begin()
|
inlineoverridevirtual |
Initial \(x\)-coordinate.
Reimplemented from G2lib::BaseCurve.
◆ x_begin_ISO()
Initial \(x\)-coordinate with offset (ISO standard).
Reimplemented from G2lib::BaseCurve.
◆ x_begin_SAE()
Initial \(x\)-coordinate with offset (SAE standard).
◆ X_D()
\(x\)-coordinate derivative at curvilinear coordinate \(s\).
Implements G2lib::BaseCurve.
◆ X_DD()
\(x\)-coordinate second derivative at curvilinear coordinate \(s\).
Implements G2lib::BaseCurve.
◆ X_DDD()
\(x\)-coordinate third derivative at curvilinear coordinate \(s\).
Implements G2lib::BaseCurve.
◆ x_end()
|
inlineoverridevirtual |
Final \(x\)-coordinate.
Reimplemented from G2lib::BaseCurve.
◆ x_end_ISO()
Final \(x\)-coordinate with offset (ISO standard).
Reimplemented from G2lib::BaseCurve.
◆ x_end_SAE()
Final \(y\)-coordinate with offset (SAE standard).
◆ X_ISO()
\(x\)-coordinate at curvilinear coordinate \(s\) with offset offs
(ISO).
Reimplemented from G2lib::BaseCurve.
◆ X_ISO_D()
\(x\)-coordinate derivative at curvilinear coordinate \(s\) with offset offs
(ISO).
Reimplemented from G2lib::BaseCurve.
◆ X_ISO_DD()
\(x\)-coordinate second derivative at curvilinear coordinate \(s\) with offset offs
(ISO).
Reimplemented from G2lib::BaseCurve.
◆ X_ISO_DDD()
\(x\)-coordinate third derivative at curvilinear coordinate \(s\) with offset offs
(ISO).
Reimplemented from G2lib::BaseCurve.
◆ X_SAE()
\(x\)-coordinate at curvilinear coordinate \(s\) with offset offs
(SAE).
◆ X_SAE_D()
\(x\)-coordinate derivative at curvilinear coordinate \(s\) with offset offs
(SAE).
◆ X_SAE_DD()
\(x\)-coordinate second derivative at curvilinear coordinate \(s\) with offset offs
(SAE).
◆ X_SAE_DDD()
\(x\)-coordinate third derivative at curvilinear coordinate \(s\) with offset offs
(SAE).
◆ Y()
\(y\)-coordinate at curvilinear coordinate \(s\).
Implements G2lib::BaseCurve.
◆ y_begin()
|
inlineoverridevirtual |
Initial \(y\)-coordinate.
Reimplemented from G2lib::BaseCurve.
◆ y_begin_ISO()
Initial \(y\)-coordinate with offset (ISO standard).
Reimplemented from G2lib::BaseCurve.
◆ y_begin_SAE()
Initial \(y\)-coordinate with offset (SAE standard).
◆ Y_D()
\(y\)-coordinate derivative at curvilinear coordinate \(s\).
Implements G2lib::BaseCurve.
◆ Y_DD()
\(y\)-coordinate second derivative at curvilinear coordinate \(s\).
Implements G2lib::BaseCurve.
◆ Y_DDD()
\(y\)-coordinate third derivative at curvilinear coordinate \(s\).
Implements G2lib::BaseCurve.
◆ y_end()
|
inlineoverridevirtual |
Final \(y\)-coordinate.
Reimplemented from G2lib::BaseCurve.
◆ y_end_ISO()
Final \(y\)-coordinate with offset (ISO standard).
Reimplemented from G2lib::BaseCurve.
◆ y_end_SAE()
Final \(y\)-coordinate with offset (ISO standard).
◆ Y_ISO()
\(y\)-coordinate at curvilinear coordinate \(s\) with offset offs
(ISO).
Reimplemented from G2lib::BaseCurve.
◆ Y_ISO_D()
\(y\)-coordinate derivative at curvilinear coordinate \(s\) with offset offs
(ISO).
Reimplemented from G2lib::BaseCurve.
◆ Y_ISO_DD()
\(y\)-coordinate second derivative at curvilinear coordinate \(s\) with offset offs
(ISO).
Reimplemented from G2lib::BaseCurve.
◆ Y_ISO_DDD()
\(y\)-coordinate third derivative at curvilinear coordinate \(s\) with offset offs
(ISO).
Reimplemented from G2lib::BaseCurve.
◆ Y_SAE()
\(y\)-coordinate at curvilinear coordinate \(s\) with offset offs
(SAE).
◆ Y_SAE_D()
\(y\)-coordinate derivative at curvilinear coordinate \(s\) with offset offs
(SAE).
◆ Y_SAE_DD()
\(y\)-coordinate second derivative at curvilinear coordinate \(s\) with offset offs
(SAE).
◆ Y_SAE_DDD()
\(y\)-coordinate third derivative at curvilinear coordinate \(s\) with offset offs
(SAE).
Friends And Related Symbol Documentation
◆ operator<<
|
friend |
Print on strem the ClothoidList
object
- Parameters
-
stream the output stream CL an instance of ClothoidList
object
- Returns
- the output stream
The documentation for this class was generated from the following files:
- /Users/enrico/Ricerca/develop/PINS/pins-mechatronix/LibSources/submodules/Clothoids/src/Clothoids/ClothoidList.hxx
- /Users/enrico/Ricerca/develop/PINS/pins-mechatronix/LibSources/submodules/Clothoids/src/ClothoidList.cc
Generated by