Dubins3p Class Reference
Clothoids
|
#include <Dubins3p.hxx>
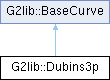
Public Member Functions | |
Dubins3p ()=delete | |
Dubins3p (string const &name) | |
void | setup (GenericContainer const &gc) override |
Dubins3p (Dubins3p const &s) | |
Dubins3p (real_type xi, real_type yi, real_type thetai, real_type xm, real_type ym, real_type xf, real_type yf, real_type thetaf, real_type k_max, Dubins3pBuildType method, string const &name) | |
void | copy (Dubins3p const &d3p) |
bool | build (real_type xi, real_type yi, real_type thetai, real_type xm, real_type ym, real_type xf, real_type yf, real_type thetaf, real_type k_max, Dubins3pBuildType method) |
void | set_tolerance (real_type tol) |
void | set_sample_angle (real_type ang) |
void | set_max_evaluation (integer max_eval) |
void | set_sample_points (integer npts) |
real_type | tolerance () const |
real_type | sample_angle () const |
DubinsType | solution_type0 () const |
DubinsType | solution_type1 () const |
integer | icode () const |
integer | icode0 () const |
integer | icode1 () const |
integer | num_evaluation () const |
integer | max_num_evaluation () const |
string | solution_type_string () const |
string | solution_type_string_short () const |
void | build (LineSegment const &L) |
void | build (CircleArc const &C) |
void | build (ClothoidCurve const &) |
void | build (Biarc const &) |
void | build (BiarcList const &) |
void | build (PolyLine const &) |
void | build (ClothoidList const &) |
void | build (Dubins const &) |
void | build (Dubins3p const &) |
integer | get_range_angles (real_type xi, real_type yi, real_type thetai, real_type xm, real_type ym, real_type xf, real_type yf, real_type thetaf, real_type k_max, real_type angles[]) const |
void | get_sample_angles (real_type xi, real_type yi, real_type thetai, real_type xm, real_type ym, real_type xf, real_type yf, real_type thetaf, real_type k_max, real_type tolerance, vector< real_type > &angles) const |
void | bbox (real_type &xmin, real_type &ymin, real_type &xmax, real_type &ymax) const override |
void | bbox_ISO (real_type offs, real_type &xmin, real_type &ymin, real_type &xmax, real_type &ymax) const override |
CircleArc const & | C0 () const |
Return the first cicle of the Dubins solution. | |
CircleArc const & | C1 () const |
Return the second cicle of the Dubins solution. | |
CircleArc const & | C2 () const |
Return the third cicle of the Dubins solution. | |
CircleArc const & | C3 () const |
Return the first cicle of the Dubins solution. | |
CircleArc const & | C4 () const |
Return the seco4d cicle of the Dubins solution. | |
CircleArc const & | C5 () const |
Return the third cicle of the Dubins solution. | |
void | get_solution (ClothoidList &CL) const |
real_type | length () const override |
real_type | length_ISO (real_type offs) const override |
real_type | length0 () const |
real_type | length1 () const |
real_type | length2 () const |
real_type | length3 () const |
real_type | length4 () const |
real_type | length5 () const |
real_type | kappa0 () const |
real_type | kappa1 () const |
real_type | kappa2 () const |
real_type | kappa3 () const |
real_type | kappa4 () const |
real_type | kappa5 () const |
real_type | X0 (real_type s) const |
real_type | Y0 (real_type s) const |
real_type | X1 (real_type s) const |
real_type | Y1 (real_type s) const |
real_type | X2 (real_type s) const |
real_type | Y2 (real_type s) const |
real_type | X3 (real_type s) const |
real_type | Y3 (real_type s) const |
real_type | X4 (real_type s) const |
real_type | Y4 (real_type s) const |
real_type | X5 (real_type s) const |
real_type | Y5 (real_type s) const |
real_type | theta0 (real_type s) const |
real_type | theta1 (real_type s) const |
real_type | theta2 (real_type s) const |
real_type | theta3 (real_type s) const |
real_type | theta4 (real_type s) const |
real_type | theta5 (real_type s) const |
real_type | theta_begin () const override |
real_type | theta_end () const override |
real_type | kappa_begin () const override |
real_type | kappa_end () const override |
real_type | x_begin () const override |
real_type | x_end () const override |
real_type | y_begin () const override |
real_type | y_end () const override |
real_type | tx_begin () const override |
real_type | tx_end () const override |
real_type | ty_begin () const override |
real_type | ty_end () const override |
real_type | nx_begin_ISO () const override |
real_type | nx_end_ISO () const override |
real_type | ny_begin_ISO () const override |
real_type | ny_end_ISO () const override |
real_type | theta0_begin () const |
real_type | theta0_end () const |
real_type | theta1_begin () const |
real_type | theta1_end () const |
real_type | theta2_begin () const |
real_type | theta2_end () const |
real_type | theta3_begin () const |
real_type | theta3_end () const |
real_type | theta4_begin () const |
real_type | theta4_end () const |
real_type | theta5_begin () const |
real_type | theta5_end () const |
real_type | x0_begin () const |
real_type | x0_end () const |
real_type | y0_begin () const |
real_type | y0_end () const |
real_type | x1_begin () const |
real_type | x1_end () const |
real_type | y1_begin () const |
real_type | y1_end () const |
real_type | x2_begin () const |
real_type | x2_end () const |
real_type | y2_begin () const |
real_type | y2_end () const |
real_type | x3_begin () const |
real_type | x3_end () const |
real_type | y3_begin () const |
real_type | y3_end () const |
real_type | x4_begin () const |
real_type | x4_end () const |
real_type | y4_begin () const |
real_type | y4_end () const |
real_type | x5_begin () const |
real_type | x5_end () const |
real_type | y5_begin () const |
real_type | y5_end () const |
real_type | theta (real_type s) const override |
real_type | theta_D (real_type) const override |
real_type | theta_DD (real_type) const override |
real_type | theta_DDD (real_type) const override |
real_type | X (real_type s) const override |
real_type | Y (real_type s) const override |
real_type | X_D (real_type s) const override |
real_type | Y_D (real_type s) const override |
real_type | X_DD (real_type s) const override |
real_type | Y_DD (real_type s) const override |
real_type | X_DDD (real_type s) const override |
real_type | Y_DDD (real_type s) const override |
void | translate (real_type tx, real_type ty) override |
translate curve by \((t_x,t_y)\) | |
void | rotate (real_type angle, real_type cx, real_type cy) override |
void | reverse () override |
void | change_origin (real_type newx0, real_type newy0) override |
void | trim (real_type, real_type) override |
void | scale (real_type s) override |
void | eval (real_type s, real_type &theta, real_type &kappa, real_type &x, real_type &y) const |
void | eval (real_type s, real_type &x, real_type &y) const override |
void | eval_D (real_type s, real_type &x_D, real_type &y_D) const override |
void | eval_DD (real_type s, real_type &x_DD, real_type &y_DD) const override |
void | eval_DDD (real_type s, real_type &x_DDD, real_type &y_DDD) const override |
void | eval_ISO (real_type s, real_type offs, real_type &x, real_type &y) const override |
void | eval_ISO_D (real_type s, real_type offs, real_type &x_D, real_type &y_D) const override |
void | eval_ISO_DD (real_type s, real_type offs, real_type &x_DD, real_type &y_DD) const override |
void | eval_ISO_DDD (real_type s, real_type offs, real_type &x_DDD, real_type &y_DDD) const override |
void | bb_triangles (vector< Triangle2D > &tvec, real_type max_angle=Utils::m_pi/18, real_type max_size=1e100, integer icurve=0) const override |
void | bb_triangles_ISO (real_type offs, vector< Triangle2D > &tvec, real_type max_angle=Utils::m_pi/18, real_type max_size=1e100, integer icurve=0) const override |
void | bb_triangles_SAE (real_type offs, vector< Triangle2D > &tvec, real_type max_angle=Utils::m_pi/18, real_type max_size=1e100, integer icurve=0) const override |
integer | closest_point_ISO (real_type qx, real_type qy, real_type &x, real_type &y, real_type &s, real_type &t, real_type &dst) const override |
integer | closest_point_ISO (real_type qx, real_type qy, real_type offs, real_type &x, real_type &y, real_type &s, real_type &t, real_type &dst) const override |
bool | collision (Dubins3p const &B) const |
bool | collision_ISO (real_type offs, Dubins3p const &B, real_type offs_B) const |
bool | collision (BaseCurve const *pC) const override |
bool | collision_ISO (real_type offs, BaseCurve const *pC, real_type offs_C) const override |
void | intersect (Dubins3p const &B, IntersectList &ilist) const |
void | intersect_ISO (real_type offs, Dubins3p const &B, real_type offs_B, IntersectList &ilist) const |
void | intersect (BaseCurve const *pC, IntersectList &ilist) const override |
void | intersect_ISO (real_type offs, BaseCurve const *pC, real_type offs_LS, IntersectList &ilist) const override |
string | info () const |
void | info (ostream_type &stream) const override |
CurveType | type () const override |
![]() | |
BaseCurve (BaseCurve const &)=delete | |
BaseCurve const & | operator= (BaseCurve const &)=delete |
BaseCurve (string const &name) | |
void | build (GenericContainer const &gc) |
string | name () const |
string | type_name () const |
real_type | length_SAE (real_type offs) const |
void | bbox_SAE (real_type offs, real_type &xmin, real_type &ymin, real_type &xmax, real_type &ymax) const |
virtual real_type | x_begin_ISO (real_type offs) const |
virtual real_type | y_begin_ISO (real_type offs) const |
virtual real_type | x_end_ISO (real_type offs) const |
virtual real_type | y_end_ISO (real_type offs) const |
real_type | x_begin_SAE (real_type offs) const |
real_type | y_begin_SAE (real_type offs) const |
real_type | x_end_SAE (real_type offs) const |
real_type | y_end_SAE (real_type offs) const |
real_type | nx_begin_SAE () const |
real_type | ny_begin_SAE () const |
real_type | nx_end_SAE () const |
real_type | ny_end_SAE () const |
real_type | kappa (real_type s) const |
real_type | kappa_D (real_type s) const |
real_type | kappa_DD (real_type s) const |
virtual real_type | tx (real_type s) const |
virtual real_type | ty (real_type s) const |
virtual real_type | tx_D (real_type s) const |
virtual real_type | ty_D (real_type s) const |
virtual real_type | tx_DD (real_type s) const |
virtual real_type | ty_DD (real_type s) const |
virtual real_type | tx_DDD (real_type s) const |
virtual real_type | ty_DDD (real_type s) const |
real_type | nx_ISO (real_type s) const |
real_type | nx_ISO_D (real_type s) const |
real_type | nx_ISO_DD (real_type s) const |
real_type | nx_ISO_DDD (real_type s) const |
real_type | ny_ISO (real_type s) const |
real_type | ny_ISO_D (real_type s) const |
real_type | ny_ISO_DD (real_type s) const |
real_type | ny_ISO_DDD (real_type s) const |
real_type | nx_SAE (real_type s) const |
real_type | nx_SAE_D (real_type s) const |
real_type | nx_SAE_DD (real_type s) const |
real_type | nx_SAE_DDD (real_type s) const |
real_type | ny_SAE (real_type s) const |
real_type | ny_SAE_D (real_type s) const |
real_type | ny_SAE_DD (real_type s) const |
real_type | ny_SAE_DDD (real_type s) const |
virtual void | tg (real_type s, real_type &tg_x, real_type &tg_y) const |
virtual void | tg_D (real_type s, real_type &tg_x_D, real_type &tg_y_D) const |
virtual void | tg_DD (real_type s, real_type &tg_x_DD, real_type &tg_y_DD) const |
virtual void | tg_DDD (real_type s, real_type &tg_x_DDD, real_type &tg_y_DDD) const |
void | nor_ISO (real_type s, real_type &nx, real_type &ny) const |
void | nor_ISO_D (real_type s, real_type &nx_D, real_type &ny_D) const |
void | nor_ISO_DD (real_type s, real_type &nx_DD, real_type &ny_DD) const |
void | nor_ISO_DDD (real_type s, real_type &nx_DDD, real_type &ny_DDD) const |
void | nor_SAE (real_type s, real_type &nx, real_type &ny) const |
void | nor_SAE_D (real_type s, real_type &nx_D, real_type &ny_D) const |
void | nor_SAE_DD (real_type s, real_type &nx_DD, real_type &ny_DD) const |
void | nor_SAE_DDD (real_type s, real_type &nx_DDD, real_type &ny_DDD) const |
virtual void | evaluate (real_type s, real_type &th, real_type &k, real_type &x, real_type &y) const |
virtual void | evaluate_ISO (real_type s, real_type offs, real_type &th, real_type &k, real_type &x, real_type &y) const |
virtual void | evaluate_SAE (real_type s, real_type offs, real_type &th, real_type &k, real_type &x, real_type &y) const |
virtual real_type | X_ISO (real_type s, real_type offs) const |
virtual real_type | Y_ISO (real_type s, real_type offs) const |
virtual real_type | X_ISO_D (real_type s, real_type offs) const |
virtual real_type | Y_ISO_D (real_type s, real_type offs) const |
virtual real_type | X_ISO_DD (real_type s, real_type offs) const |
virtual real_type | Y_ISO_DD (real_type s, real_type offs) const |
virtual real_type | X_ISO_DDD (real_type s, real_type offs) const |
virtual real_type | Y_ISO_DDD (real_type s, real_type offs) const |
real_type | X_SAE (real_type s, real_type offs) const |
real_type | Y_SAE (real_type s, real_type offs) const |
real_type | X_SAE_D (real_type s, real_type offs) const |
real_type | Y_SAE_D (real_type s, real_type offs) const |
real_type | X_SAE_DD (real_type s, real_type offs) const |
real_type | Y_SAE_DD (real_type s, real_type offs) const |
real_type | X_SAE_DDD (real_type s, real_type offs) const |
real_type | Y_SAE_DDD (real_type s, real_type offs) const |
void | eval_SAE (real_type s, real_type offs, real_type &x, real_type &y) const |
void | eval_SAE_D (real_type s, real_type offs, real_type &x_D, real_type &y_D) const |
void | eval_SAE_DD (real_type s, real_type offs, real_type &x_DD, real_type &y_DD) const |
void | eval_SAE_DDD (real_type s, real_type offs, real_type &x_DDD, real_type &y_DDD) const |
bool | collision_SAE (real_type offs, BaseCurve const *pC, real_type offs_C) const |
void | intersect_SAE (real_type offs, BaseCurve const *pC, real_type offs_C, IntersectList &ilist) const |
integer | closest_point_SAE (real_type qx, real_type qy, real_type &x, real_type &y, real_type &s, real_type &t, real_type &dst) const |
integer | closest_point_SAE (real_type qx, real_type qy, real_type offs, real_type &x, real_type &y, real_type &s, real_type &t, real_type &dst) const |
virtual real_type | distance (real_type qx, real_type qy) const |
real_type | distance_ISO (real_type qx, real_type qy, real_type offs) const |
real_type | distance_SAE (real_type qx, real_type qy, real_type offs) const |
bool | findST_ISO (real_type x, real_type y, real_type &s, real_type &t) const |
bool | findST_SAE (real_type x, real_type y, real_type &s, real_type &t) const |
Friends | |
ostream_type & | operator<< (ostream_type &stream, Dubins3p const &bi) |
Detailed Description
Class to manage a circle arc
Constructor & Destructor Documentation
◆ Dubins3p() [1/3]
|
delete |
Build an empty circle
◆ Dubins3p() [2/3]
◆ Dubins3p() [3/3]
|
inlineexplicit |
Construct a Dubins3p solution
- Parameters
-
[in] xi initial position \(x\)-coordinate [in] yi initial position \(y\)-coordinate [in] thetai initial angle [in] xm intermediate position \(x\)-coordinate [in] ym intermediate position \(y\)-coordinate [in] xf final position \(x\)-coordinate [in] yf final position \(y\)-coordinate [in] thetaf final angle [in] k_max max curvature [in] method construction method [in] name name of the 3 points Dubins object
Member Function Documentation
◆ bb_triangles()
|
inlineoverridevirtual |
Build a cover with triangles of the curve.
- Parameters
-
[out] tvec list of covering triangles [out] max_angle maximum angle variation of the curve covered by a triangle [out] max_size maximum admissible size of the covering tirnagles [out] icurve index of the covering triangles
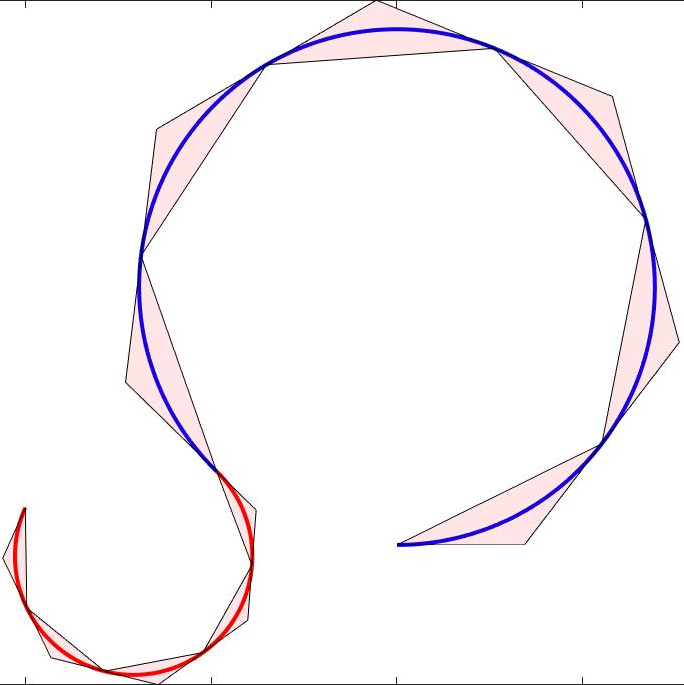
Implements G2lib::BaseCurve.
◆ bb_triangles_ISO()
|
inlineoverridevirtual |
Build a cover with triangles of the curve with offset (ISO).
- Parameters
-
[out] offs curve offset [out] tvec list of covering triangles [out] max_angle maximum angle variation of the curve covered by a triangle [out] max_size maximum admissible size of the covering tirnagles [out] icurve index of the covering triangles
Implements G2lib::BaseCurve.
◆ bb_triangles_SAE()
|
inlineoverridevirtual |
Build a cover with triangles of the curve with offset (SAE).
- Parameters
-
[out] offs curve offset [out] tvec list of covering triangles [out] max_angle maximum angle variation of the arc covered by a triangle [out] max_size maximum admissible size of the covering tirnagles [out] icurve index of the covering triangles
Implements G2lib::BaseCurve.
◆ bbox()
|
overridevirtual |
Compute the bounding box of the curve.
- Parameters
-
[out] xmin left bottom [out] ymin left bottom [out] xmax right top [out] ymax right top
Implements G2lib::BaseCurve.
◆ bbox_ISO()
|
overridevirtual |
Compute the bounding box of the curve with offset (ISO).
- Parameters
-
[in] offs curve offset [out] xmin left bottom [out] ymin left bottom [out] xmax right top [out] ymax right top
Implements G2lib::BaseCurve.
◆ build()
bool G2lib::Dubins3p::build | ( | real_type | xi, |
real_type | yi, | ||
real_type | thetai, | ||
real_type | xm, | ||
real_type | ym, | ||
real_type | xf, | ||
real_type | yf, | ||
real_type | thetaf, | ||
real_type | k_max, | ||
Dubins3pBuildType | method ) |
Construct a Dubins3p solution
- Parameters
-
[in] xi initial position \(x\)-coordinate [in] yi initial position \(y\)-coordinate [in] thetai initial angle [in] xm intermediate position \(x\)-coordinate [in] ym intermediate position \(y\)-coordinate [in] xf final position \(x\)-coordinate [in] yf final position \(y\)-coordinate [in] thetaf final angle [in] k_max max curvature [in] method construction method
◆ change_origin()
Translate curve so that origin will be (newx0
, newy0
).
Implements G2lib::BaseCurve.
◆ closest_point_ISO() [1/2]
|
overridevirtual |
Given a point find closest point on the curve.
- Parameters
-
qx \(x\)-coordinate of the point qy \(y\)-coordinate of the point x \(x\)-coordinate of the projected point on the curve y \(y\)-coordinate of the projected point on the curve s parameter on the curve of the projection t curvilinear coordinate of the point x,y (if orthogonal projection) dst distance point projected point
- Returns
- 1 = point is projected orthogonal 0 = more than one projection (first returned) -1 = minimum point is not othogonal projection to curve
Implements G2lib::BaseCurve.
◆ closest_point_ISO() [2/2]
|
overridevirtual |
Given a point find closest point on the curve.
- Parameters
-
qx \(x\)-coordinate of the point qy \(y\)-coordinate of the point offs offset of the curve x \(x\)-coordinate of the projected point on the curve y \(y\)-coordinate of the projected point on the curve s parameter on the curve of the projection t curvilinear coordinate of the point x,y (if orthogonal projection) dst distance point projected point
- Returns
- 1 = point is projected orthogonal 0 = more than one projection (first returned) -1 = minimum point is not othogonal projection to curve
Implements G2lib::BaseCurve.
◆ collision() [1/2]
|
overridevirtual |
Check collision with another curve.
Implements G2lib::BaseCurve.
◆ collision() [2/2]
bool G2lib::Dubins3p::collision | ( | Dubins3p const & | B | ) | const |
Detect a collision with another biarc.
◆ collision_ISO() [1/2]
|
overridevirtual |
Check collision with another curve with offset (ISO).
- Parameters
-
[in] offs curve offset [in] pC second curve to check collision [in] offs_C curve offset of the second curve
- Returns
- true if collision is detected
Implements G2lib::BaseCurve.
◆ collision_ISO() [2/2]
Detect a collision with another biarc with offset.
- Parameters
-
[in] offs offset of first biarc [in] B second biarc [in] offs_B offset of second biarc
◆ copy()
|
inline |
Make a copy of an existing Dubins solution.
◆ eval()
x and \(y\)-coordinate at curvilinear coordinate \(s\).
Implements G2lib::BaseCurve.
◆ eval_D()
|
overridevirtual |
x and \(y\)-coordinate derivative at curvilinear coordinate \(s\).
Implements G2lib::BaseCurve.
◆ eval_DD()
|
overridevirtual |
x and \(y\)-coordinate second derivative at curvilinear coordinate \(s\).
Implements G2lib::BaseCurve.
◆ eval_DDD()
|
overridevirtual |
x and \(y\)-coordinate third derivative at curvilinear coordinate \(s\).
Implements G2lib::BaseCurve.
◆ eval_ISO()
|
overridevirtual |
Compute curve at position s
with offset offs
(ISO).
- Parameters
-
[in] s parameter on the curve [in] offs offset of the curve [out] x coordinate [out] y coordinate
Reimplemented from G2lib::BaseCurve.
◆ eval_ISO_D()
|
overridevirtual |
Compute derivative curve at position s
with offset offs
(ISO).
- Parameters
-
[in] s parameter on the curve [in] offs offset of the curve [out] x_D \(x\)-coordinate [out] y_D \(y\)-coordinate
Reimplemented from G2lib::BaseCurve.
◆ eval_ISO_DD()
|
overridevirtual |
Compute second derivative curve at position s
with offset offs
(ISO).
- Parameters
-
[in] s parameter on the curve [in] offs offset of the curve [out] x_DD \(x\)-coordinate second derivative [out] y_DD \(y\)-coordinate second derivative
Reimplemented from G2lib::BaseCurve.
◆ eval_ISO_DDD()
|
overridevirtual |
Compute third derivative curve at position s
with offset offs
(ISO).
- Parameters
-
[in] s parameter on the curve [in] offs offset of the curve [out] x_DDD \(x\)-coordinate third derivative [out] y_DDD \(y\)-coordinate third derivative
Reimplemented from G2lib::BaseCurve.
◆ get_range_angles()
integer G2lib::Dubins3p::get_range_angles | ( | real_type | xi, |
real_type | yi, | ||
real_type | thetai, | ||
real_type | xm, | ||
real_type | ym, | ||
real_type | xf, | ||
real_type | yf, | ||
real_type | thetaf, | ||
real_type | k_max, | ||
real_type | angles[] ) const |
Get possible point of discontinuity for the length
- Parameters
-
[in] xi initial position \(x\)-coordinate [in] yi initial position \(y\)-coordinate [in] thetai initial angle [in] xm intermediate position \(x\)-coordinate [in] ym intermediate position \(y\)-coordinate [in] xf final position \(x\)-coordinate [in] yf final position \(y\)-coordinate [in] thetaf final angle [in] k_max max curvature [out] angles angles of possibile dicontinuity
- Returns
- number of point computed
◆ get_sample_angles()
void G2lib::Dubins3p::get_sample_angles | ( | real_type | xi, |
real_type | yi, | ||
real_type | thetai, | ||
real_type | xm, | ||
real_type | ym, | ||
real_type | xf, | ||
real_type | yf, | ||
real_type | thetaf, | ||
real_type | k_max, | ||
real_type | tolerance, | ||
vector< real_type > & | angles ) const |
Get sample point for length minimization
- Parameters
-
[in] xi initial position \(x\)-coordinate [in] yi initial position \(y\)-coordinate [in] thetai initial angle [in] xm intermediate position \(x\)-coordinate [in] ym intermediate position \(y\)-coordinate [in] xf final position \(x\)-coordinate [in] yf final position \(y\)-coordinate [in] thetaf final angle [in] k_max max curvature [in] tolerance tolerance angle used in point approximaton [out] angles sample points
◆ info()
|
inlineoverridevirtual |
Pretty print of the curve data.
Implements G2lib::BaseCurve.
◆ intersect() [1/2]
|
overridevirtual |
Intersect the curve with another curve.
- Parameters
-
[in] pC second curve intersect [out] ilist list of the intersection (as parameter on the curves)
Implements G2lib::BaseCurve.
◆ intersect() [2/2]
void G2lib::Dubins3p::intersect | ( | Dubins3p const & | B, |
IntersectList & | ilist ) const |
Intersect a biarc with another biarc.
- Parameters
-
[in] B second biarc [out] ilist list of the intersection (as parameter on the curves)
◆ intersect_ISO() [1/2]
|
overridevirtual |
Intersect the curve with another curve with offset (ISO)
- Parameters
-
[in] offs offset first curve [in] pC second curve intersect [in] offs_C offset second curve [out] ilist list of the intersection (as parameter on the curves)
Implements G2lib::BaseCurve.
◆ intersect_ISO() [2/2]
void G2lib::Dubins3p::intersect_ISO | ( | real_type | offs, |
Dubins3p const & | B, | ||
real_type | offs_B, | ||
IntersectList & | ilist ) const |
Intersect a biarc with another biarc with offset (ISO).
- Parameters
-
[in] offs offset of first biarc [in] B second Dubins [in] offs_B offset of second biarc [out] ilist list of the intersection (as parameter on the curves)
◆ kappa_begin()
|
inlineoverridevirtual |
Initial curvature.
Reimplemented from G2lib::BaseCurve.
◆ kappa_end()
|
inlineoverridevirtual |
Final curvature.
Reimplemented from G2lib::BaseCurve.
◆ length()
|
overridevirtual |
The length of the curve
Implements G2lib::BaseCurve.
◆ length_ISO()
The length of the curve with offset (ISO)
Implements G2lib::BaseCurve.
◆ nx_begin_ISO()
|
inlineoverridevirtual |
Intial normal \(x\)-coordinate (ISO).
Reimplemented from G2lib::BaseCurve.
◆ nx_end_ISO()
|
inlineoverridevirtual |
Final normal \(x\)-coordinate (ISO).
Reimplemented from G2lib::BaseCurve.
◆ ny_begin_ISO()
|
inlineoverridevirtual |
Intial normal \(y\)-coordinate (ISO).
Reimplemented from G2lib::BaseCurve.
◆ ny_end_ISO()
|
inlineoverridevirtual |
Final normal \(y\)-coordinate (ISO).
Reimplemented from G2lib::BaseCurve.
◆ reverse()
|
overridevirtual |
Reverse curve parameterization.
Implements G2lib::BaseCurve.
◆ rotate()
Rotate curve by angle \(\theta\) centered at point \((c_x,c_y)\).
- Parameters
-
[in] angle angle \(\theta\) [in] cx \(c_x\) [in] cy \(c_y\)
Implements G2lib::BaseCurve.
◆ scale()
|
overridevirtual |
Scale curve by factor sc
.
Implements G2lib::BaseCurve.
◆ setup()
|
overridevirtual |
Implements G2lib::BaseCurve.
◆ theta()
Angle at curvilinear coordinate \(s\).
Implements G2lib::BaseCurve.
◆ theta_begin()
|
inlineoverridevirtual |
Initial angle of the curve.
Reimplemented from G2lib::BaseCurve.
◆ theta_D()
Angle derivative (curvature) at curvilinear coordinate \(s\).
Implements G2lib::BaseCurve.
◆ theta_DD()
Angle second derivative (devitive of curvature) at curvilinear coordinate \(s\).
Implements G2lib::BaseCurve.
◆ theta_DDD()
Angle third derivative at curvilinear coordinate \(s\).
Implements G2lib::BaseCurve.
◆ theta_end()
|
inlineoverridevirtual |
Final angle of the curve.
Reimplemented from G2lib::BaseCurve.
◆ translate()
translate curve by \((t_x,t_y)\)
Implements G2lib::BaseCurve.
◆ trim()
Cut curve at parametric coordinate s_begin
and s_end
.
Implements G2lib::BaseCurve.
◆ tx_begin()
|
inlineoverridevirtual |
Initial tangent \(x\)-coordinate.
Reimplemented from G2lib::BaseCurve.
◆ tx_end()
|
inlineoverridevirtual |
Final tangent \(x\)-coordinate.
Reimplemented from G2lib::BaseCurve.
◆ ty_begin()
|
inlineoverridevirtual |
Initial tangent \(y\)-coordinate.
Reimplemented from G2lib::BaseCurve.
◆ ty_end()
|
inlineoverridevirtual |
Final tangent \(y\)-coordinate.
Reimplemented from G2lib::BaseCurve.
◆ type()
|
inlineoverridevirtual |
The name of the curve type
Implements G2lib::BaseCurve.
◆ X()
\(x\)-coordinate at curvilinear coordinate \(s\).
Implements G2lib::BaseCurve.
◆ x_begin()
|
inlineoverridevirtual |
Initial \(x\)-coordinate.
Reimplemented from G2lib::BaseCurve.
◆ X_D()
\(x\)-coordinate derivative at curvilinear coordinate \(s\).
Implements G2lib::BaseCurve.
◆ X_DD()
\(x\)-coordinate second derivative at curvilinear coordinate \(s\).
Implements G2lib::BaseCurve.
◆ X_DDD()
\(x\)-coordinate third derivative at curvilinear coordinate \(s\).
Implements G2lib::BaseCurve.
◆ x_end()
|
inlineoverridevirtual |
Final \(x\)-coordinate.
Reimplemented from G2lib::BaseCurve.
◆ Y()
\(y\)-coordinate at curvilinear coordinate \(s\).
Implements G2lib::BaseCurve.
◆ y_begin()
|
inlineoverridevirtual |
Initial \(y\)-coordinate.
Reimplemented from G2lib::BaseCurve.
◆ Y_D()
\(y\)-coordinate derivative at curvilinear coordinate \(s\).
Implements G2lib::BaseCurve.
◆ Y_DD()
\(y\)-coordinate second derivative at curvilinear coordinate \(s\).
Implements G2lib::BaseCurve.
◆ Y_DDD()
\(y\)-coordinate third derivative at curvilinear coordinate \(s\).
Implements G2lib::BaseCurve.
◆ y_end()
|
inlineoverridevirtual |
Final \(y\)-coordinate.
Reimplemented from G2lib::BaseCurve.
Friends And Related Symbol Documentation
◆ operator<<
|
friend |
The documentation for this class was generated from the following files:
- /Users/enrico/Ricerca/develop/PINS/pins-mechatronix/LibSources/submodules/Clothoids/src/Clothoids/Dubins3p.hxx
- /Users/enrico/Ricerca/develop/PINS/pins-mechatronix/LibSources/submodules/Clothoids/src/Dubins3p.cc
- /Users/enrico/Ricerca/develop/PINS/pins-mechatronix/LibSources/submodules/Clothoids/src/Dubins3p_ellipse.cc
- /Users/enrico/Ricerca/develop/PINS/pins-mechatronix/LibSources/submodules/Clothoids/src/Dubins3p_pattern.cc
Generated by