BaseCurve Class Reference
Clothoids
|
#include <BaseCurve.hxx>
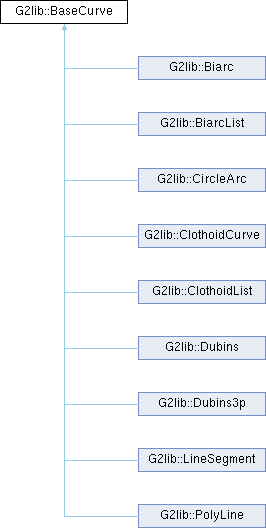
Detailed Description
Base classe for all the curve ìn in the library.
Constructor & Destructor Documentation
◆ BaseCurve()
|
inline |
Initialize the class storing the curve type.
Member Function Documentation
◆ bb_triangles()
|
pure virtual |
Build a cover with triangles of the curve.
- Parameters
-
[out] tvec list of covering triangles [out] max_angle maximum angle variation of the curve covered by a triangle [out] max_size maximum admissible size of the covering tirnagles [out] icurve index of the covering triangles
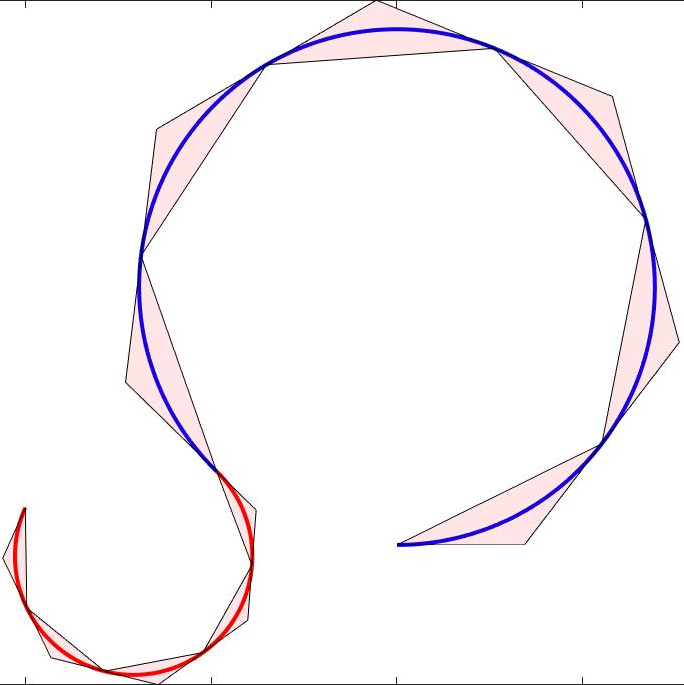
Implemented in G2lib::Biarc, G2lib::BiarcList, G2lib::CircleArc, G2lib::ClothoidCurve, G2lib::ClothoidList, G2lib::Dubins3p, G2lib::Dubins, G2lib::LineSegment, and G2lib::PolyLine.
◆ bb_triangles_ISO()
|
pure virtual |
Build a cover with triangles of the curve with offset (ISO).
- Parameters
-
[out] offs curve offset [out] tvec list of covering triangles [out] max_angle maximum angle variation of the curve covered by a triangle [out] max_size maximum admissible size of the covering tirnagles [out] icurve index of the covering triangles
Implemented in G2lib::Biarc, G2lib::BiarcList, G2lib::CircleArc, G2lib::ClothoidCurve, G2lib::ClothoidList, G2lib::Dubins3p, G2lib::Dubins, G2lib::LineSegment, and G2lib::PolyLine.
◆ bb_triangles_SAE()
|
pure virtual |
Build a cover with triangles of the curve with offset (SAE).
- Parameters
-
[out] offs curve offset [out] tvec list of covering triangles [out] max_angle maximum angle variation of the arc covered by a triangle [out] max_size maximum admissible size of the covering tirnagles [out] icurve index of the covering triangles
Implemented in G2lib::Biarc, G2lib::BiarcList, G2lib::CircleArc, G2lib::ClothoidCurve, G2lib::ClothoidList, G2lib::Dubins3p, G2lib::Dubins, G2lib::LineSegment, and G2lib::PolyLine.
◆ bbox()
|
pure virtual |
Compute the bounding box of the curve.
- Parameters
-
[out] xmin left bottom [out] ymin left bottom [out] xmax right top [out] ymax right top
Implemented in G2lib::Biarc, G2lib::BiarcList, G2lib::CircleArc, G2lib::ClothoidCurve, G2lib::ClothoidList, G2lib::Dubins3p, G2lib::Dubins, G2lib::LineSegment, and G2lib::PolyLine.
◆ bbox_ISO()
|
pure virtual |
Compute the bounding box of the curve with offset (ISO).
- Parameters
-
[in] offs curve offset [out] xmin left bottom [out] ymin left bottom [out] xmax right top [out] ymax right top
Implemented in G2lib::Biarc, G2lib::BiarcList, G2lib::CircleArc, G2lib::ClothoidCurve, G2lib::ClothoidList, G2lib::Dubins3p, G2lib::Dubins, G2lib::LineSegment, and G2lib::PolyLine.
◆ bbox_SAE()
|
inline |
Compute the bounding box of the curve (SAE).
- Parameters
-
[in] offs curve offset [out] xmin left bottom [out] ymin left bottom [out] xmax right top [out] ymax right top
◆ change_origin()
Translate curve so that origin will be (newx0
, newy0
).
Implemented in G2lib::Biarc, G2lib::BiarcList, G2lib::CircleArc, G2lib::ClothoidCurve, G2lib::ClothoidList, G2lib::Dubins3p, G2lib::Dubins, G2lib::LineSegment, and G2lib::PolyLine.
◆ closest_point_ISO() [1/2]
|
pure virtual |
Given a point find closest point on the curve.
- Parameters
-
qx \(x\)-coordinate of the point qy \(y\)-coordinate of the point x \(x\)-coordinate of the projected point on the curve y \(y\)-coordinate of the projected point on the curve s parameter on the curve of the projection t curvilinear coordinate of the point x,y (if orthogonal projection) dst distance point projected point
- Returns
- 1 = point is projected orthogonal 0 = more than one projection (first returned) -1 = minimum point is not othogonal projection to curve
Implemented in G2lib::Biarc, G2lib::BiarcList, G2lib::CircleArc, G2lib::ClothoidCurve, G2lib::ClothoidList, G2lib::Dubins3p, G2lib::Dubins, G2lib::LineSegment, and G2lib::PolyLine.
◆ closest_point_ISO() [2/2]
|
pure virtual |
Given a point find closest point on the curve.
- Parameters
-
qx \(x\)-coordinate of the point qy \(y\)-coordinate of the point offs offset of the curve x \(x\)-coordinate of the projected point on the curve y \(y\)-coordinate of the projected point on the curve s parameter on the curve of the projection t curvilinear coordinate of the point x,y (if orthogonal projection) dst distance point projected point
- Returns
- 1 = point is projected orthogonal 0 = more than one projection (first returned) -1 = minimum point is not othogonal projection to curve
Implemented in G2lib::Biarc, G2lib::BiarcList, G2lib::CircleArc, G2lib::ClothoidCurve, G2lib::ClothoidList, G2lib::Dubins3p, G2lib::Dubins, G2lib::LineSegment, and G2lib::PolyLine.
◆ closest_point_SAE() [1/2]
|
inline |
Given a point find closest point on the curve.
- Parameters
-
qx \(x\)-coordinate of the point qy \(y\)-coordinate of the point x \(x\)-coordinate of the projected point on the curve y \(y\)-coordinate of the projected point on the curve s parameter on the curve of the projection t curvilinear coordinate of the point x,y (if orthogonal projection) dst distance point projected point
- Returns
- 1 = point is projected orthogonal 0 = more than one projection (first returned) -1 = minimum point is not othogonal projection to curve
◆ closest_point_SAE() [2/2]
|
inline |
Given a point find closest point on the curve.
- Parameters
-
qx \(x\)-coordinate of the point qy \(y\)-coordinate of the point offs offset of the curve x \(x\)-coordinate of the projected point on the curve y \(y\)-coordinate of the projected point on the curve s parameter on the curve of the projection t curvilinear coordinate of the point x,y (if orthogonal projection) dst distance point projected point
- Returns
- 1 = point is projected orthogonal 0 = more than one projection (first returned) -1 = minimum point is not othogonal projection to curve
◆ collision()
|
pure virtual |
Check collision with another curve.
Implemented in G2lib::Biarc, G2lib::BiarcList, G2lib::CircleArc, G2lib::ClothoidCurve, G2lib::ClothoidList, G2lib::Dubins3p, G2lib::Dubins, G2lib::LineSegment, and G2lib::PolyLine.
◆ collision_ISO()
|
pure virtual |
Check collision with another curve with offset (ISO).
- Parameters
-
[in] offs curve offset [in] pC second curve to check collision [in] offs_C curve offset of the second curve
- Returns
- true if collision is detected
Implemented in G2lib::Biarc, G2lib::BiarcList, G2lib::CircleArc, G2lib::ClothoidCurve, G2lib::ClothoidList, G2lib::Dubins3p, G2lib::Dubins, G2lib::LineSegment, and G2lib::PolyLine.
◆ collision_SAE()
|
inline |
Check collision with another curve with offset (SAE).
- Parameters
-
[in] offs curve offset [in] pC second curve to check collision [in] offs_C curve offset of the second curve
- Returns
- true if collision is detected
◆ distance()
Compute the distance between a point \(q=(q_x,q_y)\) and the curve.
- Parameters
-
[in] qx component \(q_x\) [in] qy component \(q_y\)
- Returns
- the computed distance
◆ distance_ISO()
|
inline |
Compute the distance between a point \(q=(q_x,q_y)\) and the curve with offset (ISO).
- Parameters
-
[in] qx component \(q_x\) [in] qy component \(q_y\) [in] offs offset of the curve
- Returns
- the computed distance
◆ distance_SAE()
|
inline |
Compute the distance between a point \(q=(q_x,q_y)\) and the curve with offset (SAE).
- Parameters
-
[in] qx component \(q_x\) [in] qy component \(q_y\) [in] offs offset of the curve
- Returns
- the computed distance
◆ eval()
|
pure virtual |
x and \(y\)-coordinate at curvilinear coordinate \(s\).
Implemented in G2lib::Biarc, G2lib::BiarcList, G2lib::CircleArc, G2lib::ClothoidCurve, G2lib::ClothoidList, G2lib::Dubins3p, G2lib::Dubins, G2lib::LineSegment, and G2lib::PolyLine.
◆ eval_D()
|
pure virtual |
x and \(y\)-coordinate derivative at curvilinear coordinate \(s\).
Implemented in G2lib::Biarc, G2lib::BiarcList, G2lib::CircleArc, G2lib::ClothoidCurve, G2lib::ClothoidList, G2lib::Dubins3p, G2lib::Dubins, G2lib::LineSegment, and G2lib::PolyLine.
◆ eval_DD()
|
pure virtual |
x and \(y\)-coordinate second derivative at curvilinear coordinate \(s\).
Implemented in G2lib::Biarc, G2lib::BiarcList, G2lib::CircleArc, G2lib::ClothoidCurve, G2lib::ClothoidList, G2lib::Dubins3p, G2lib::Dubins, G2lib::LineSegment, and G2lib::PolyLine.
◆ eval_DDD()
|
pure virtual |
x and \(y\)-coordinate third derivative at curvilinear coordinate \(s\).
Implemented in G2lib::Biarc, G2lib::BiarcList, G2lib::CircleArc, G2lib::ClothoidCurve, G2lib::ClothoidList, G2lib::Dubins3p, G2lib::Dubins, G2lib::LineSegment, and G2lib::PolyLine.
◆ eval_ISO()
|
virtual |
Compute curve at position s
with offset offs
(ISO).
- Parameters
-
[in] s parameter on the curve [in] offs offset of the curve [out] x coordinate [out] y coordinate
Reimplemented in G2lib::Biarc, G2lib::BiarcList, G2lib::CircleArc, G2lib::ClothoidCurve, G2lib::ClothoidList, G2lib::Dubins3p, G2lib::Dubins, G2lib::LineSegment, and G2lib::PolyLine.
◆ eval_ISO_D()
|
virtual |
Compute derivative curve at position s
with offset offs
(ISO).
- Parameters
-
[in] s parameter on the curve [in] offs offset of the curve [out] x_D \(x\)-coordinate [out] y_D \(y\)-coordinate
Reimplemented in G2lib::Biarc, G2lib::BiarcList, G2lib::CircleArc, G2lib::ClothoidCurve, G2lib::ClothoidList, G2lib::Dubins3p, G2lib::Dubins, G2lib::LineSegment, and G2lib::PolyLine.
◆ eval_ISO_DD()
|
virtual |
Compute second derivative curve at position s
with offset offs
(ISO).
- Parameters
-
[in] s parameter on the curve [in] offs offset of the curve [out] x_DD \(x\)-coordinate second derivative [out] y_DD \(y\)-coordinate second derivative
Reimplemented in G2lib::Biarc, G2lib::BiarcList, G2lib::CircleArc, G2lib::ClothoidCurve, G2lib::ClothoidList, G2lib::Dubins3p, G2lib::Dubins, G2lib::LineSegment, and G2lib::PolyLine.
◆ eval_ISO_DDD()
|
virtual |
Compute third derivative curve at position s
with offset offs
(ISO).
- Parameters
-
[in] s parameter on the curve [in] offs offset of the curve [out] x_DDD \(x\)-coordinate third derivative [out] y_DDD \(y\)-coordinate third derivative
Reimplemented in G2lib::Biarc, G2lib::BiarcList, G2lib::CircleArc, G2lib::ClothoidCurve, G2lib::ClothoidList, G2lib::Dubins3p, G2lib::Dubins, G2lib::LineSegment, and G2lib::PolyLine.
◆ eval_SAE()
|
inline |
Compute curve at position s
with offset offs
(SAE).
- Parameters
-
[in] s parameter on the curve [in] offs offset of the curve [out] x coordinate [out] y coordinate
◆ eval_SAE_D()
|
inline |
Compute derivative curve at position s
with offset offs
(SAE).
- Parameters
-
[in] s parameter on the curve [in] offs offset of the curve [out] x_D \(x\)-coordinate first derivative [out] y_D \(y\)-coordinate first derivative
◆ eval_SAE_DD()
|
inline |
Compute second derivative curve at position s
with offset offs
(SAE).
- Parameters
-
[in] s parameter on the curve [in] offs offset of the curve [out] x_DD \(x\)-coordinate second derivative [out] y_DD \(y\)-coordinate second derivative
◆ eval_SAE_DDD()
|
inline |
Compute third derivative curve at position s
with offset offs
(SAE).
- Parameters
-
[in] s parameter on the curve [in] offs offset of the curve [out] x_DDD \(x\)-coordinate third derivative [out] y_DDD \(y\)-coordinate third derivative
◆ evaluate()
|
inlinevirtual |
Evaluate curve at curvilinear coordinate \(s\).
- Parameters
-
[in] s curvilinear coordinate [out] th angle [out] k curvature [out] x \(x\)-coordinate [out] y \(y\)-coordinate
Reimplemented in G2lib::Biarc, G2lib::BiarcList, G2lib::CircleArc, and G2lib::ClothoidList.
◆ evaluate_ISO()
|
inlinevirtual |
Evaluate curve with offset at curvilinear coordinate \(s\) (ISO).
- Parameters
-
[in] s curvilinear coordinate [in] offs offset [out] th angle [out] k curvature [out] x \(x\)-coordinate [out] y \(y\)-coordinate
Reimplemented in G2lib::Biarc, G2lib::BiarcList, G2lib::CircleArc, G2lib::ClothoidCurve, G2lib::ClothoidList, and G2lib::LineSegment.
◆ evaluate_SAE()
|
inlinevirtual |
Evaluate curve with offset at curvilinear coordinate \(s\) (SAE).
- Parameters
-
[in] s curvilinear coordinate [in] offs offset [out] th angle [out] k curvature [out] x \(x\)-coordinate [out] y \(y\)-coordinate
Reimplemented in G2lib::Biarc, G2lib::BiarcList, G2lib::CircleArc, G2lib::ClothoidCurve, G2lib::ClothoidList, and G2lib::LineSegment.
◆ findST_ISO()
|
inline |
Find the curvilinear coordinate of point \(P=(x,y)\) respect to the curve (ISO), i.e.
\[ P = C(s)+N(s)t \]
where \(C(s)\) is the curve position respect to the curvilinear coordinates and \(C(s)\) is the normal at the point \(C(s)\).
- Parameters
-
[in] x component \(x\) [in] y component \(y\) [out] s curvilinear coordinate [out] t offset respect to the curve of \((x,y)\)
- Returns
- true if the coordinate are found
◆ findST_SAE()
|
inline |
Find the curvilinear coordinate of point \((x,y)\) respect to the curve (SAE), i.e.
\[ P = C(s)+N(s)t \]
where \(C(s)\) is the curve position respect to the curvilinear coordinates and \(C(s)\) is the normal at the point \(C(s)\).
- Parameters
-
[in] x component \(x\) [in] y component \(y\) [out] s curvilinear coordinate [out] t offset respect to the curve of \((x,y)\)
- Returns
- true if the coordinate are found
◆ info()
|
pure virtual |
Pretty print of the curve data.
Implemented in G2lib::Biarc, G2lib::BiarcList, G2lib::CircleArc, G2lib::ClothoidCurve, G2lib::ClothoidList, G2lib::Dubins3p, G2lib::Dubins, G2lib::LineSegment, and G2lib::PolyLine.
◆ intersect()
|
pure virtual |
Intersect the curve with another curve.
- Parameters
-
[in] pC second curve intersect [out] ilist list of the intersection (as parameter on the curves)
Implemented in G2lib::Biarc, G2lib::BiarcList, G2lib::CircleArc, G2lib::ClothoidCurve, G2lib::ClothoidList, G2lib::Dubins3p, G2lib::Dubins, G2lib::LineSegment, and G2lib::PolyLine.
◆ intersect_ISO()
|
pure virtual |
Intersect the curve with another curve with offset (ISO)
- Parameters
-
[in] offs offset first curve [in] pC second curve intersect [in] offs_C offset second curve [out] ilist list of the intersection (as parameter on the curves)
Implemented in G2lib::Biarc, G2lib::BiarcList, G2lib::CircleArc, G2lib::ClothoidCurve, G2lib::ClothoidList, G2lib::Dubins3p, G2lib::Dubins, G2lib::LineSegment, and G2lib::PolyLine.
◆ intersect_SAE()
|
inline |
Intersect the curve with another curve with offset (SAE).
- Parameters
-
[in] offs offset first curve [in] pC second curve intersect [in] offs_C offset second curve [out] ilist list of the intersection (as parameter on the curves)
◆ kappa()
Ccurvature at curvilinear coordinate \(s\).
◆ kappa_begin()
|
inlinevirtual |
Initial curvature.
Reimplemented in G2lib::Biarc, G2lib::CircleArc, G2lib::ClothoidCurve, G2lib::Dubins3p, and G2lib::Dubins.
◆ kappa_D()
Curvature derivative at curvilinear coordinate \(s\).
◆ kappa_DD()
Curvature second derivative at curvilinear coordinate \(s\).
◆ kappa_end()
|
inlinevirtual |
Final curvature.
Reimplemented in G2lib::Biarc, G2lib::CircleArc, G2lib::Dubins3p, and G2lib::Dubins.
◆ length()
|
pure virtual |
The length of the curve
Implemented in G2lib::Biarc, G2lib::BiarcList, G2lib::CircleArc, G2lib::ClothoidCurve, G2lib::ClothoidList, G2lib::Dubins3p, G2lib::Dubins, G2lib::LineSegment, and G2lib::PolyLine.
◆ length_ISO()
The length of the curve with offset (ISO)
Implemented in G2lib::Biarc, G2lib::BiarcList, G2lib::CircleArc, G2lib::ClothoidCurve, G2lib::ClothoidList, G2lib::Dubins3p, G2lib::Dubins, G2lib::LineSegment, and G2lib::PolyLine.
◆ length_SAE()
The length of the curve with offset (SAE)
◆ nor_ISO()
Normal at curvilinear coordinate \(s\) (ISO).
◆ nor_ISO_D()
Normal derivative at curvilinear coordinate \(s\) (ISO).
◆ nor_ISO_DD()
|
inline |
Normal second derivative at curvilinear coordinate \(s\) (ISO).
◆ nor_ISO_DDD()
|
inline |
Normal third derivative at curvilinear coordinate \(s\) (ISO).
◆ nor_SAE()
Normal at curvilinear coordinate \(s\) (SAE).
◆ nor_SAE_D()
Normal derivative at curvilinear coordinate \(s\) (SAE).
◆ nor_SAE_DD()
|
inline |
Normal second derivative at curvilinear coordinate \(s\) (SAE).
◆ nor_SAE_DDD()
|
inline |
Normal third at curvilinear coordinate \(s\) (SAE).
◆ nx_begin_ISO()
|
inlinevirtual |
Intial normal \(x\)-coordinate (ISO).
Reimplemented in G2lib::Biarc, G2lib::BiarcList, G2lib::CircleArc, G2lib::ClothoidCurve, G2lib::ClothoidList, G2lib::Dubins3p, G2lib::Dubins, and G2lib::LineSegment.
◆ nx_begin_SAE()
|
inline |
Intial normal \(x\)-coordinate (SAE).
◆ nx_end_ISO()
|
inlinevirtual |
Final normal \(x\)-coordinate (ISO).
Reimplemented in G2lib::Biarc, G2lib::BiarcList, G2lib::CircleArc, G2lib::ClothoidCurve, G2lib::ClothoidList, G2lib::Dubins3p, G2lib::Dubins, and G2lib::LineSegment.
◆ nx_end_SAE()
|
inline |
Final normal \(x\)-coordinate (SAE).
◆ nx_ISO()
Normal \(x\)-coordinate at curvilinear coordinate \(s\) (ISO).
◆ nx_ISO_D()
Normal derivative \(x\)-coordinate at curvilinear coordinate \(s\) (ISO).
◆ nx_ISO_DD()
Normal second derivative \(x\)-coordinate at curvilinear coordinate \(s\) (ISO).
◆ nx_ISO_DDD()
Normal third derivative \(x\)-coordinate at curvilinear coordinate \(s\) (ISO).
◆ nx_SAE()
Normal \(x\)-coordinate at curvilinear coordinate \(s\) (SAE).
◆ nx_SAE_D()
Normal derivative \(x\)-coordinate at curvilinear coordinate \(s\) (SAE).
◆ nx_SAE_DD()
Normal second derivative \(x\)-coordinate at curvilinear coordinate \(s\) (SAE).
◆ nx_SAE_DDD()
Normal third derivative \(x\)-coordinate at curvilinear coordinate \(s\) (SAE).
◆ ny_begin_ISO()
|
inlinevirtual |
Intial normal \(y\)-coordinate (ISO).
Reimplemented in G2lib::Biarc, G2lib::BiarcList, G2lib::CircleArc, G2lib::ClothoidCurve, G2lib::ClothoidList, G2lib::Dubins3p, G2lib::Dubins, and G2lib::LineSegment.
◆ ny_begin_SAE()
|
inline |
Intial normal \(y\)-coordinate (SAE).
◆ ny_end_ISO()
|
inlinevirtual |
Final normal \(y\)-coordinate (ISO).
Reimplemented in G2lib::Biarc, G2lib::BiarcList, G2lib::CircleArc, G2lib::ClothoidCurve, G2lib::ClothoidList, G2lib::Dubins3p, G2lib::Dubins, and G2lib::LineSegment.
◆ ny_end_SAE()
|
inline |
Intial normal \(y\)-coordinate (SAE).
◆ ny_ISO()
Normal \(y\)-coordinate at curvilinear coordinate \(s\) (ISO).
◆ ny_ISO_D()
Normal derivative \(y\)-coordinate at curvilinear coordinate \(s\) (ISO).
◆ ny_ISO_DD()
Normal second derivative \(y\)-coordinate at curvilinear coordinate \(s\) (ISO).
◆ ny_ISO_DDD()
Normal third derivative \(y\)-coordinate at curvilinear coordinate \(s\) (ISO).
◆ ny_SAE()
Normal \(y\)-coordinate at curvilinear coordinate \(s\) (ISO)
◆ ny_SAE_D()
Normal derivative \(y\)-coordinate at curvilinear coordinate \(s\) (SAE).
◆ ny_SAE_DD()
Normal second derivative \(x\)-coordinate at curvilinear coordinate \(s\) (SAE).
◆ ny_SAE_DDD()
Normal third derivative \(y\)-coordinate at curvilinear coordinate \(s\) (SAE).
◆ reverse()
|
pure virtual |
Reverse curve parameterization.
Implemented in G2lib::Biarc, G2lib::BiarcList, G2lib::CircleArc, G2lib::ClothoidCurve, G2lib::ClothoidList, G2lib::Dubins3p, G2lib::Dubins, G2lib::LineSegment, and G2lib::PolyLine.
◆ rotate()
Rotate curve by angle \(\theta\) centered at point \((c_x,c_y)\).
- Parameters
-
[in] angle angle \(\theta\) [in] cx \(c_x\) [in] cy \(c_y\)
Implemented in G2lib::Biarc, G2lib::BiarcList, G2lib::CircleArc, G2lib::ClothoidCurve, G2lib::ClothoidList, G2lib::Dubins3p, G2lib::Dubins, G2lib::LineSegment, and G2lib::PolyLine.
◆ scale()
|
pure virtual |
Scale curve by factor sc
.
Implemented in G2lib::Biarc, G2lib::BiarcList, G2lib::CircleArc, G2lib::ClothoidCurve, G2lib::ClothoidList, G2lib::Dubins3p, G2lib::Dubins, G2lib::LineSegment, and G2lib::PolyLine.
◆ tg()
|
inlinevirtual |
Tangent at curvilinear coordinate \(s\).
Reimplemented in G2lib::Biarc, G2lib::BiarcList, G2lib::CircleArc, G2lib::ClothoidCurve, G2lib::ClothoidList, and G2lib::LineSegment.
◆ tg_D()
|
inlinevirtual |
Tangent derivative at curvilinear coordinate \(s\).
Reimplemented in G2lib::Biarc, G2lib::BiarcList, G2lib::CircleArc, G2lib::ClothoidCurve, G2lib::ClothoidList, and G2lib::LineSegment.
◆ tg_DD()
|
inlinevirtual |
Tangent second derivative at curvilinear coordinate \(s\).
Reimplemented in G2lib::Biarc, G2lib::BiarcList, G2lib::CircleArc, G2lib::ClothoidCurve, G2lib::ClothoidList, and G2lib::LineSegment.
◆ tg_DDD()
|
inlinevirtual |
Tangent third derivative at curvilinear coordinate \(s\).
Reimplemented in G2lib::Biarc, G2lib::BiarcList, G2lib::CircleArc, G2lib::ClothoidCurve, G2lib::ClothoidList, and G2lib::LineSegment.
◆ theta()
Angle at curvilinear coordinate \(s\).
Implemented in G2lib::Biarc, G2lib::BiarcList, G2lib::CircleArc, G2lib::ClothoidCurve, G2lib::ClothoidList, G2lib::Dubins3p, G2lib::Dubins, G2lib::LineSegment, and G2lib::PolyLine.
◆ theta_begin()
|
inlinevirtual |
Initial angle of the curve.
Reimplemented in G2lib::Biarc, G2lib::BiarcList, G2lib::CircleArc, G2lib::ClothoidCurve, G2lib::ClothoidList, G2lib::Dubins3p, G2lib::Dubins, and G2lib::LineSegment.
◆ theta_D()
Angle derivative (curvature) at curvilinear coordinate \(s\).
Implemented in G2lib::Biarc, G2lib::BiarcList, G2lib::CircleArc, G2lib::ClothoidCurve, G2lib::ClothoidList, G2lib::Dubins3p, G2lib::Dubins, G2lib::LineSegment, and G2lib::PolyLine.
◆ theta_DD()
Angle second derivative (devitive of curvature) at curvilinear coordinate \(s\).
Implemented in G2lib::Biarc, G2lib::BiarcList, G2lib::CircleArc, G2lib::ClothoidCurve, G2lib::ClothoidList, G2lib::Dubins3p, G2lib::Dubins, G2lib::LineSegment, and G2lib::PolyLine.
◆ theta_DDD()
Angle third derivative at curvilinear coordinate \(s\).
Implemented in G2lib::Biarc, G2lib::BiarcList, G2lib::CircleArc, G2lib::ClothoidCurve, G2lib::ClothoidList, G2lib::Dubins3p, G2lib::Dubins, G2lib::LineSegment, and G2lib::PolyLine.
◆ theta_end()
|
inlinevirtual |
Final angle of the curve.
Reimplemented in G2lib::Biarc, G2lib::BiarcList, G2lib::CircleArc, G2lib::ClothoidCurve, G2lib::ClothoidList, G2lib::Dubins3p, G2lib::Dubins, and G2lib::LineSegment.
◆ translate()
translate curve by \((t_x,t_y)\)
Implemented in G2lib::Biarc, G2lib::BiarcList, G2lib::CircleArc, G2lib::ClothoidCurve, G2lib::ClothoidList, G2lib::Dubins3p, G2lib::Dubins, G2lib::LineSegment, and G2lib::PolyLine.
◆ trim()
Cut curve at parametric coordinate s_begin
and s_end
.
Implemented in G2lib::Biarc, G2lib::BiarcList, G2lib::CircleArc, G2lib::ClothoidCurve, G2lib::ClothoidList, G2lib::Dubins3p, G2lib::Dubins, G2lib::LineSegment, and G2lib::PolyLine.
◆ tx()
Tangent \(x\)-coordinate at curvilinear coordinate \(s\).
Reimplemented in G2lib::Biarc, G2lib::BiarcList, G2lib::CircleArc, G2lib::ClothoidCurve, G2lib::ClothoidList, and G2lib::LineSegment.
◆ tx_begin()
|
inlinevirtual |
Initial tangent \(x\)-coordinate.
Reimplemented in G2lib::Biarc, G2lib::BiarcList, G2lib::CircleArc, G2lib::ClothoidCurve, G2lib::ClothoidList, G2lib::Dubins3p, G2lib::Dubins, and G2lib::LineSegment.
◆ tx_D()
Tangent derivative \(x\)-coordinate at curvilinear coordinate \(s\).
Reimplemented in G2lib::Biarc, G2lib::BiarcList, G2lib::CircleArc, G2lib::ClothoidCurve, G2lib::ClothoidList, and G2lib::LineSegment.
◆ tx_DD()
Tangent second derivative \(x\)-coordinate at curvilinear coordinate \(s\).
Reimplemented in G2lib::Biarc, G2lib::BiarcList, G2lib::CircleArc, G2lib::ClothoidCurve, G2lib::ClothoidList, and G2lib::LineSegment.
◆ tx_DDD()
Tangent third derivative \(x\)-coordinate at curvilinear coordinate \(s\).
Reimplemented in G2lib::Biarc, G2lib::BiarcList, G2lib::CircleArc, G2lib::ClothoidCurve, G2lib::ClothoidList, and G2lib::LineSegment.
◆ tx_end()
|
inlinevirtual |
Final tangent \(x\)-coordinate.
Reimplemented in G2lib::Biarc, G2lib::BiarcList, G2lib::CircleArc, G2lib::ClothoidCurve, G2lib::ClothoidList, G2lib::Dubins3p, G2lib::Dubins, and G2lib::LineSegment.
◆ ty()
Tangent \(y\)-coordinate at curvilinear coordinate \(s\).
Reimplemented in G2lib::Biarc, G2lib::BiarcList, G2lib::CircleArc, G2lib::ClothoidCurve, G2lib::ClothoidList, and G2lib::LineSegment.
◆ ty_begin()
|
inlinevirtual |
Initial tangent \(y\)-coordinate.
Reimplemented in G2lib::Biarc, G2lib::BiarcList, G2lib::CircleArc, G2lib::ClothoidCurve, G2lib::ClothoidList, G2lib::Dubins3p, G2lib::Dubins, and G2lib::LineSegment.
◆ ty_D()
Tangent derivative \(y\)-coordinate at curvilinear coordinate \(s\).
Reimplemented in G2lib::Biarc, G2lib::BiarcList, G2lib::CircleArc, G2lib::ClothoidCurve, G2lib::ClothoidList, and G2lib::LineSegment.
◆ ty_DD()
Tangent second derivative \(y\)-coordinate at curvilinear coordinate \(s\).
Reimplemented in G2lib::Biarc, G2lib::BiarcList, G2lib::CircleArc, G2lib::ClothoidCurve, G2lib::ClothoidList, and G2lib::LineSegment.
◆ ty_DDD()
Tangent third derivative \(y\)-coordinate at curvilinear coordinate \(s\).
Reimplemented in G2lib::Biarc, G2lib::BiarcList, G2lib::CircleArc, G2lib::ClothoidCurve, G2lib::ClothoidList, and G2lib::LineSegment.
◆ ty_end()
|
inlinevirtual |
Final tangent \(y\)-coordinate.
Reimplemented in G2lib::Biarc, G2lib::BiarcList, G2lib::CircleArc, G2lib::ClothoidCurve, G2lib::ClothoidList, G2lib::Dubins3p, G2lib::Dubins, and G2lib::LineSegment.
◆ type()
|
pure virtual |
The name of the curve type
Implemented in G2lib::Biarc, G2lib::BiarcList, G2lib::CircleArc, G2lib::ClothoidCurve, G2lib::ClothoidList, G2lib::Dubins3p, G2lib::Dubins, G2lib::LineSegment, and G2lib::PolyLine.
◆ X()
\(x\)-coordinate at curvilinear coordinate \(s\).
Implemented in G2lib::Biarc, G2lib::BiarcList, G2lib::CircleArc, G2lib::ClothoidCurve, G2lib::ClothoidList, G2lib::Dubins3p, G2lib::Dubins, G2lib::LineSegment, and G2lib::PolyLine.
◆ x_begin()
|
inlinevirtual |
Initial \(x\)-coordinate.
Reimplemented in G2lib::Biarc, G2lib::BiarcList, G2lib::CircleArc, G2lib::ClothoidCurve, G2lib::ClothoidList, G2lib::Dubins3p, G2lib::Dubins, G2lib::LineSegment, and G2lib::PolyLine.
◆ x_begin_ISO()
Initial \(x\)-coordinate with offset (ISO standard).
Reimplemented in G2lib::Biarc, G2lib::BiarcList, G2lib::CircleArc, G2lib::ClothoidCurve, G2lib::ClothoidList, and G2lib::LineSegment.
◆ x_begin_SAE()
Initial \(x\)-coordinate with offset (SAE standard).
◆ X_D()
\(x\)-coordinate derivative at curvilinear coordinate \(s\).
Implemented in G2lib::Biarc, G2lib::BiarcList, G2lib::CircleArc, G2lib::ClothoidCurve, G2lib::ClothoidList, G2lib::Dubins3p, G2lib::Dubins, G2lib::LineSegment, and G2lib::PolyLine.
◆ X_DD()
\(x\)-coordinate second derivative at curvilinear coordinate \(s\).
Implemented in G2lib::Biarc, G2lib::BiarcList, G2lib::CircleArc, G2lib::ClothoidCurve, G2lib::ClothoidList, G2lib::Dubins3p, G2lib::Dubins, G2lib::LineSegment, and G2lib::PolyLine.
◆ X_DDD()
\(x\)-coordinate third derivative at curvilinear coordinate \(s\).
Implemented in G2lib::Biarc, G2lib::BiarcList, G2lib::CircleArc, G2lib::ClothoidCurve, G2lib::ClothoidList, G2lib::Dubins3p, G2lib::Dubins, G2lib::LineSegment, and G2lib::PolyLine.
◆ x_end()
|
inlinevirtual |
Final \(x\)-coordinate.
Reimplemented in G2lib::Biarc, G2lib::BiarcList, G2lib::CircleArc, G2lib::ClothoidCurve, G2lib::ClothoidList, G2lib::Dubins3p, G2lib::Dubins, G2lib::LineSegment, and G2lib::PolyLine.
◆ x_end_ISO()
Final \(x\)-coordinate with offset (ISO standard).
Reimplemented in G2lib::Biarc, G2lib::BiarcList, G2lib::CircleArc, G2lib::ClothoidCurve, G2lib::ClothoidList, and G2lib::LineSegment.
◆ x_end_SAE()
Final \(y\)-coordinate with offset (SAE standard).
◆ X_ISO()
\(x\)-coordinate at curvilinear coordinate \(s\) with offset offs
(ISO).
Reimplemented in G2lib::Biarc, G2lib::BiarcList, G2lib::CircleArc, G2lib::ClothoidCurve, G2lib::ClothoidList, and G2lib::LineSegment.
◆ X_ISO_D()
\(x\)-coordinate derivative at curvilinear coordinate \(s\) with offset offs
(ISO).
Reimplemented in G2lib::Biarc, G2lib::BiarcList, G2lib::CircleArc, G2lib::ClothoidCurve, G2lib::ClothoidList, and G2lib::LineSegment.
◆ X_ISO_DD()
\(x\)-coordinate second derivative at curvilinear coordinate \(s\) with offset offs
(ISO).
Reimplemented in G2lib::Biarc, G2lib::BiarcList, G2lib::CircleArc, G2lib::ClothoidCurve, G2lib::ClothoidList, and G2lib::LineSegment.
◆ X_ISO_DDD()
\(x\)-coordinate third derivative at curvilinear coordinate \(s\) with offset offs
(ISO).
Reimplemented in G2lib::Biarc, G2lib::BiarcList, G2lib::CircleArc, G2lib::ClothoidCurve, G2lib::ClothoidList, and G2lib::LineSegment.
◆ X_SAE()
\(x\)-coordinate at curvilinear coordinate \(s\) with offset offs
(SAE).
◆ X_SAE_D()
\(x\)-coordinate derivative at curvilinear coordinate \(s\) with offset offs
(SAE).
◆ X_SAE_DD()
\(x\)-coordinate second derivative at curvilinear coordinate \(s\) with offset offs
(SAE).
◆ X_SAE_DDD()
\(x\)-coordinate third derivative at curvilinear coordinate \(s\) with offset offs
(SAE).
◆ Y()
\(y\)-coordinate at curvilinear coordinate \(s\).
Implemented in G2lib::Biarc, G2lib::BiarcList, G2lib::CircleArc, G2lib::ClothoidCurve, G2lib::ClothoidList, G2lib::Dubins3p, G2lib::Dubins, G2lib::LineSegment, and G2lib::PolyLine.
◆ y_begin()
|
inlinevirtual |
Initial \(y\)-coordinate.
Reimplemented in G2lib::Biarc, G2lib::BiarcList, G2lib::CircleArc, G2lib::ClothoidCurve, G2lib::ClothoidList, G2lib::Dubins3p, G2lib::Dubins, G2lib::LineSegment, and G2lib::PolyLine.
◆ y_begin_ISO()
Initial \(y\)-coordinate with offset (ISO standard).
Reimplemented in G2lib::Biarc, G2lib::BiarcList, G2lib::CircleArc, G2lib::ClothoidCurve, G2lib::ClothoidList, and G2lib::LineSegment.
◆ y_begin_SAE()
Initial \(y\)-coordinate with offset (SAE standard).
◆ Y_D()
\(y\)-coordinate derivative at curvilinear coordinate \(s\).
Implemented in G2lib::Biarc, G2lib::BiarcList, G2lib::CircleArc, G2lib::ClothoidCurve, G2lib::ClothoidList, G2lib::Dubins3p, G2lib::Dubins, G2lib::LineSegment, and G2lib::PolyLine.
◆ Y_DD()
\(y\)-coordinate second derivative at curvilinear coordinate \(s\).
Implemented in G2lib::Biarc, G2lib::BiarcList, G2lib::CircleArc, G2lib::ClothoidCurve, G2lib::ClothoidList, G2lib::Dubins3p, G2lib::Dubins, G2lib::LineSegment, and G2lib::PolyLine.
◆ Y_DDD()
\(y\)-coordinate third derivative at curvilinear coordinate \(s\).
Implemented in G2lib::Biarc, G2lib::BiarcList, G2lib::CircleArc, G2lib::ClothoidCurve, G2lib::ClothoidList, G2lib::Dubins3p, G2lib::Dubins, G2lib::LineSegment, and G2lib::PolyLine.
◆ y_end()
|
inlinevirtual |
Final \(y\)-coordinate.
Reimplemented in G2lib::Biarc, G2lib::BiarcList, G2lib::CircleArc, G2lib::ClothoidCurve, G2lib::ClothoidList, G2lib::Dubins3p, G2lib::Dubins, G2lib::LineSegment, and G2lib::PolyLine.
◆ y_end_ISO()
Final \(y\)-coordinate with offset (ISO standard).
Reimplemented in G2lib::Biarc, G2lib::BiarcList, G2lib::CircleArc, G2lib::ClothoidCurve, G2lib::ClothoidList, and G2lib::LineSegment.
◆ y_end_SAE()
Final \(y\)-coordinate with offset (ISO standard).
◆ Y_ISO()
\(y\)-coordinate at curvilinear coordinate \(s\) with offset offs
(ISO).
Reimplemented in G2lib::Biarc, G2lib::BiarcList, G2lib::CircleArc, G2lib::ClothoidCurve, G2lib::ClothoidList, and G2lib::LineSegment.
◆ Y_ISO_D()
\(y\)-coordinate derivative at curvilinear coordinate \(s\) with offset offs
(ISO).
Reimplemented in G2lib::Biarc, G2lib::BiarcList, G2lib::CircleArc, G2lib::ClothoidCurve, G2lib::ClothoidList, and G2lib::LineSegment.
◆ Y_ISO_DD()
\(y\)-coordinate second derivative at curvilinear coordinate \(s\) with offset offs
(ISO).
Reimplemented in G2lib::Biarc, G2lib::BiarcList, G2lib::CircleArc, G2lib::ClothoidCurve, G2lib::ClothoidList, and G2lib::LineSegment.
◆ Y_ISO_DDD()
\(y\)-coordinate third derivative at curvilinear coordinate \(s\) with offset offs
(ISO).
Reimplemented in G2lib::Biarc, G2lib::BiarcList, G2lib::CircleArc, G2lib::ClothoidCurve, G2lib::ClothoidList, and G2lib::LineSegment.
◆ Y_SAE()
\(y\)-coordinate at curvilinear coordinate \(s\) with offset offs
(SAE).
◆ Y_SAE_D()
\(y\)-coordinate derivative at curvilinear coordinate \(s\) with offset offs
(SAE).
◆ Y_SAE_DD()
\(y\)-coordinate second derivative at curvilinear coordinate \(s\) with offset offs
(SAE).
◆ Y_SAE_DDD()
\(y\)-coordinate third derivative at curvilinear coordinate \(s\) with offset offs
(SAE).
The documentation for this class was generated from the following file:
- /Users/enrico/Ricerca/develop/PINS/pins-mechatronix/LibSources/submodules/Clothoids/src/Clothoids/BaseCurve.hxx
Generated by