BiarcList Class Reference
Clothoids
|
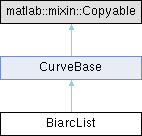
Public Member Functions | |
function | BiarcList () |
function | reserve (in self, in N) |
function | push_back (in self, in varargin) |
function | get (in self, in k) |
function | append (in self, in lst) |
function | get_XY (in self) |
function | num_segments (in self) |
function | numSegments (in self) |
function | build_G1 (in self, in varargin) |
function | build_theta (in self, in x, in y) |
function | info (in self) |
function | find_coord1 (in self, in x, in y) |
function | plot (in self, in varargin) |
function | plot_offs (in self, in offs, in npts, in varargin) |
function | plot_curvature (in self, in npts, in varargin) |
function | plotCurvature (in self, in npts, in varargin) |
function | plot_angle (in self, in npts, in varargin) |
function | plotAngle (in self, in npts, in varargin) |
function | plotNormal (in self, in step, in len) |
function | plotNormal (in self, in step, in len) |
![]() | |
function | CurveBase (in mexName, in objectType) |
function | obj_handle (in self) |
function | is_type (in self) |
function | load (in self, in OBJ) |
function | bbox (in self, in varargin) |
function | translate (in self, in tx, in ty) |
function | trim (in self, in smin, in smax) |
function | rotate (in self, in angle, in cx, in cy) |
function | reverse (in self) |
function | scale (in self, in sc) |
function | change_origin (in self, in newX0, in newY0) |
function | changeOrigin (in self, in newX0, in newY0) |
function | evaluate (in self, in s, in varargin) |
function | eval (in self, in varargin) |
function | eval_D (in self, in varargin) |
function | eval_DD (in self, in varargin) |
function | eval_DDD (in self, in varargin) |
function | theta (in self, in s) |
function | theta_D (in self, in s) |
function | theta_DD (in self, in s) |
function | theta_DDD (in self, in s) |
function | kappa (in self, in s) |
function | kappa_D (in self, in s) |
function | kappa_DD (in self, in s) |
function | xy_begin (in self) |
function | xyBegin (in self) |
function | xy_end (in self) |
function | xyEnd (in self) |
function | x_begin (in self) |
function | xBegin (in self) |
function | x_end (in self) |
function | xEnd (in self) |
function | y_begin (in self) |
function | yBegin (in self) |
function | y_end (in self) |
function | yEnd (in self) |
function | theta_begin (in self) |
function | thetaBegin (in self) |
function | theta_end (in self) |
function | thetaEnd (in self) |
function | kappa_begin (in self) |
function | kappaBegin (in self) |
function | kappa_end (in self) |
function | kappaEnd (in self) |
function | length (in self, in varargin) |
function | points (in self) |
function | bbTriangles (in self, in varargin) |
function | closest_point (in self, in qx, in qy, in varargin) |
function | closestPoint (in self, in qx, in qy, in varargin) |
function | distance (in self, in qx, in qy, in varargin) |
function | collision (in self, in OBJ, in varargin) |
function | intersect (in self, in OBJ, in varargin) |
function | info (in self) |
function | find_coord (in self, in x, in y) |
function | yesAABBtree (in self) |
function | noAABBtree (in self) |
function | plot_tbox (in self, in P1, in P2, in P3, in varargin) |
function | plotTBox (in self, in P1, in P2, in P3, in varargin) |
function | plot_bbox (in self, in varargin) |
function | plotBBox (in self, in varargin) |
function | plot_triangles (in self, in varargin) |
function | plotTriangles (in self, in varargin) |
Additional Inherited Members | |
![]() | |
function | copyElement (in self) |
![]() | |
Property | mexName |
Property | objectHandle |
Property | call_delete |
Property | objectType |
Constructor & Destructor Documentation
◆ BiarcList()
function BiarcList::BiarcList | ( | ) |
Create a new C++ class instance for the list of biarc object
Usage:
On output:
- self: reference handle to the object instance
Member Function Documentation
◆ append()
function BiarcList::append | ( | in | self, |
in | lst ) |
Append a biarc or a biarc list.
◆ build_G1()
function BiarcList::build_G1 | ( | in | self, |
in | varargin ) |
Build a biarc list given a set of points and if available the angles at the points. If the angles are missing the angle at a node is computed by building the circle passing by 3 consecutive points. The node is the middle point.
x
,y
: vectors ofx
andy
coordinates of the nodesthetas
: angles at the nodes
◆ build_theta()
function BiarcList::build_theta | ( | in | self, |
in | x, | ||
in | y ) |
Build the angles a the list of nodes. The angle at a node is computed by building the circle passing by 3 consecutive points. The node is the middle point.
x
,y
: vectors ofx
andy
coordinates of the nodes
◆ find_coord1()
function BiarcList::find_coord1 | ( | in | self, |
in | x, | ||
in | y ) |
Find curvilinear coordinates of inputs points
Input:
x
,y
: vectors ofx
andy
coordinates of the poinst
Output:
s
,t
: curvilinar coordinates of the pointsipos
: the segment with point at minimal distance, otherwise -(idx+1) if (x,y) cannot be projected orthogonally on the segment
◆ get()
function BiarcList::get | ( | in | self, |
in | k ) |
Get the biarc at position k
. The biarc is returned as a biarc object or the data defining the biarc.
◆ get_XY()
function BiarcList::get_XY | ( | in | self | ) |
Return the list of points (initial and final) of the biarcs
◆ num_segments()
function BiarcList::num_segments | ( | in | self | ) |
Return number of biarc in the list
◆ numSegments()
function BiarcList::numSegments | ( | in | self | ) |
- Deprecated
- whill be removed in future version
◆ plot()
function BiarcList::plot | ( | in | self, |
in | varargin ) |
Plot the biarc list
Usage:
npts
: number of sampling points for plottingfmt1
: format of the first arcfmt2
: format of the second arc
◆ plot_angle()
function BiarcList::plot_angle | ( | in | self, |
in | npts, | ||
in | varargin ) |
Plot the angle of the biarc list
Usage:
npts
: number of sampling points for plottingfmt1
: format of the first arcfmt2
: format of the second arc
◆ plot_curvature()
function BiarcList::plot_curvature | ( | in | self, |
in | npts, | ||
in | varargin ) |
Plot the curvature of the biarc list
Usage:
npts
: number of sampling points for plottingfmt1
: format of the first arcfmt2
: format of the second arc
◆ plot_offs()
function BiarcList::plot_offs | ( | in | self, |
in | offs, | ||
in | npts, | ||
in | varargin ) |
Plot the biarc list with offset
Usage:
npts
: number of sampling points for plottingfmt1
: format of the first arcfmt2
: format of the second arcoffs
: offset used in the plotting
◆ plotAngle()
function BiarcList::plotAngle | ( | in | self, |
in | npts, | ||
in | varargin ) |
- Deprecated
- whill be removed in future version
◆ plotCurvature()
function BiarcList::plotCurvature | ( | in | self, |
in | npts, | ||
in | varargin ) |
- Deprecated
- whill be removed in future version
◆ plotNormal() [1/2]
function BiarcList::plotNormal | ( | in | self, |
in | step, | ||
in | len ) |
Plot the normal of the biarc list
Usage:
step
: number of sampling normalslen
: length of the plotted normal
◆ plotNormal() [2/2]
function BiarcList::plotNormal | ( | in | self, |
in | step, | ||
in | len ) |
- Deprecated
- whill be removed in future version
◆ push_back()
function BiarcList::push_back | ( | in | self, |
in | varargin ) |
Append to the BiarcList another biarc. The biarc is obtained setting the final postion and angle while the initial position and angle are taken from the last biarc on the biarc list. Another possibility is to push a biarc is obtained by passing initial and final positions, initial and final angles.
Usage:
x0
,y0
: initial position of the biarc to be appendedtheta0
: initial angle of the biarc to be appendedx1
,y1
: final position of the biarc to be appendedtheta1
: final angle of the biarc to be appended
◆ reserve()
function BiarcList::reserve | ( | in | self, |
in | N ) |
Reserve memory for N
biarc
Usage:
The documentation for this class was generated from the following file:
- /Users/enrico/Ricerca/develop/PINS/pins-mechatronix/LibSources/submodules/Clothoids/toolbox/lib/BiarcList.m
Generated by