CurveBase Class Reference
Clothoids
|
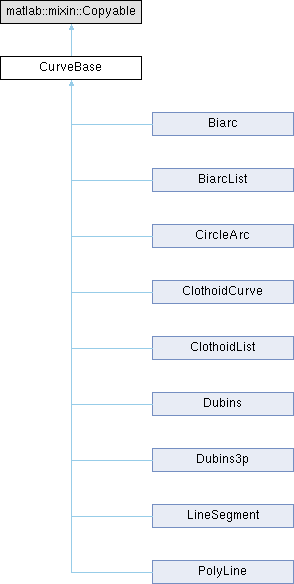
Public Member Functions | |
function | CurveBase (in mexName, in objectType) |
function | obj_handle (in self) |
function | is_type (in self) |
function | load (in self, in OBJ) |
function | bbox (in self, in varargin) |
function | translate (in self, in tx, in ty) |
function | trim (in self, in smin, in smax) |
function | rotate (in self, in angle, in cx, in cy) |
function | reverse (in self) |
function | scale (in self, in sc) |
function | change_origin (in self, in newX0, in newY0) |
function | changeOrigin (in self, in newX0, in newY0) |
function | evaluate (in self, in s, in varargin) |
function | eval (in self, in varargin) |
function | eval_D (in self, in varargin) |
function | eval_DD (in self, in varargin) |
function | eval_DDD (in self, in varargin) |
function | theta (in self, in s) |
function | theta_D (in self, in s) |
function | theta_DD (in self, in s) |
function | theta_DDD (in self, in s) |
function | kappa (in self, in s) |
function | kappa_D (in self, in s) |
function | kappa_DD (in self, in s) |
function | xy_begin (in self) |
function | xyBegin (in self) |
function | xy_end (in self) |
function | xyEnd (in self) |
function | x_begin (in self) |
function | xBegin (in self) |
function | x_end (in self) |
function | xEnd (in self) |
function | y_begin (in self) |
function | yBegin (in self) |
function | y_end (in self) |
function | yEnd (in self) |
function | theta_begin (in self) |
function | thetaBegin (in self) |
function | theta_end (in self) |
function | thetaEnd (in self) |
function | kappa_begin (in self) |
function | kappaBegin (in self) |
function | kappa_end (in self) |
function | kappaEnd (in self) |
function | length (in self, in varargin) |
function | points (in self) |
function | bbTriangles (in self, in varargin) |
function | closest_point (in self, in qx, in qy, in varargin) |
function | closestPoint (in self, in qx, in qy, in varargin) |
function | distance (in self, in qx, in qy, in varargin) |
function | collision (in self, in OBJ, in varargin) |
function | intersect (in self, in OBJ, in varargin) |
function | info (in self) |
function | find_coord (in self, in x, in y) |
function | yesAABBtree (in self) |
function | noAABBtree (in self) |
function | plot_tbox (in self, in P1, in P2, in P3, in varargin) |
function | plotTBox (in self, in P1, in P2, in P3, in varargin) |
function | plot_bbox (in self, in varargin) |
function | plotBBox (in self, in varargin) |
function | plot_triangles (in self, in varargin) |
function | plotTriangles (in self, in varargin) |
Protected Member Functions | |
function | copyElement (in self) |
Protected Attributes | |
Property | mexName |
Property | objectHandle |
Property | call_delete |
Property | objectType |
Member Function Documentation
◆ bbox()
function CurveBase::bbox | ( | in | self, |
in | varargin ) |
Return the bounding box of the curve object
Usage
- xmin: x minimum coordinate of the bounding box
- ymin: y minimum coordinate of the bounding box
- xmax: x maximum coordinate of the bounding box
- ymax: y maximum coordinate of the bounding box
Optional Arguments
- offs: offset of the curve used in the bbox computation
- 'ISO'/'SAE': use ISO or SAE orientation of the normal for offset computation
◆ bbTriangles()
function CurveBase::bbTriangles | ( | in | self, |
in | varargin ) |
Evaluate the bounding box triangles of curve.
Usage
Optional Arguments
max_angle
: maximum curve angle variation admitted in a trianglemax_size
: maximum triangles sizeoffs
: offset of the curve used in computation- 'ISO'/'SAE': use ISO or SAE orientation of the normal for the offset
Output
P1
:2 x n
matrix with the first points of the trianglesP2
:2 x n
matrix with the second points of the trianglesP3
:2 x n
matrix with the third points of the triangles
◆ change_origin()
function CurveBase::change_origin | ( | in | self, |
in | newX0, | ||
in | newY0 ) |
Translate the curve in such a way the origin is at (newX0,newY0)
.
Usage
◆ changeOrigin()
function CurveBase::changeOrigin | ( | in | self, |
in | newX0, | ||
in | newY0 ) |
- Deprecated
- whill be removed in future version
◆ closest_point()
function CurveBase::closest_point | ( | in | self, |
in | qx, | ||
in | qy, | ||
in | varargin ) |
Evaluate the point at minimum distance of another point on the curve. qx
and qy
may be vectors so that the return values are vectors too.
Usage
Optional Arguments
- offs: offset of the curve used in computation
- 'ISO'/'SAE': use ISO or SAE orientation of the normal for the offset
Output
x
,y
: Point at minimum distance from(qx,qy)
on the curve.s
,t
: Curvilinear coordinates of the point(qx,qy)
.iflag
:iflag < 0
some error in computation, iflag >0 is the numer of segment containing the point at minimum distance.dst
: point curve distance.
◆ closestPoint()
function CurveBase::closestPoint | ( | in | self, |
in | qx, | ||
in | qy, | ||
in | varargin ) |
- Deprecated
- whill be removed in future version
◆ collision()
function CurveBase::collision | ( | in | self, |
in | OBJ, | ||
in | varargin ) |
Check if two curve collide.
Usage
Optional Arguments
offs
,offs1
: offset of the curves used in computation- 'ISO'/'SAE': use ISO or SAE orientation of the normal for the offsets
◆ copyElement()
|
protected |
Make a deep copy of a curve object
Usage
where A
is the curve object to be copied.
◆ distance()
function CurveBase::distance | ( | in | self, |
in | qx, | ||
in | qy, | ||
in | varargin ) |
Evaluate the distance of a point (qx,qy)
to the curve. qx
and qy
may be vectors so that the return values are vectors too.
Usage
Optional Arguments
offs
: offset of the curve used in computation- 'ISO'/'SAE': use ISO or SAE orientation of the normal for the offset
◆ eval()
function CurveBase::eval | ( | in | self, |
in | varargin ) |
Evaluate the curve at curvilinear coordinate s
. Argument s
may be a vector for multiple evaluations.
Usage
Optional Arguments
- offs: offset of the curve used compiutation
- 'ISO'/'SAE': use ISO or SAE orientation of the normal for offset computation
Output
- XY: matrix
2 x n
of the evaluated points - X: vector of the x-coordinates of the evaluated points
- Y: vector of the y-coordinates of the evaluated points
◆ eval_D()
function CurveBase::eval_D | ( | in | self, |
in | varargin ) |
Evaluate the first derivatives of the curve at curvilinear coordinate s
. Argument s
may be a vector for multiple evaluations.
Usage
Optional Arguments
offs
: offset of the curve used in computation- 'ISO'/'SAE': use ISO or SAE orientation of the normal for offset computation
Output
XY
: matrix2 x n
of the evaluated pointsX
: vector of the x-coordinates of the evaluated point derivativesY
: vector of the y-coordinates of the evaluated point derivatives
◆ eval_DD()
function CurveBase::eval_DD | ( | in | self, |
in | varargin ) |
Evaluate the second derivatives of the curve at curvilinear coordinate s
. Argument s
may be a vector for multiple evaluations.
Usage
Optional Arguments
offs
: offset of the curve used in computation- 'ISO'/'SAE': use ISO or SAE orientation of the normal for offset computation
Output
XY
: matrix2 x n
of the evaluated pointsX
: vector of the x-coordinates of the evaluated point derivativesY
: vector of the y-coordinates of the evaluated point derivatives
◆ eval_DDD()
function CurveBase::eval_DDD | ( | in | self, |
in | varargin ) |
Evaluate the third derivatives of the curve at curvilinear coordinate s
. Argument s
may be a vector for multiple evaluations.
Usage
Optional Arguments
offs
: offset of the curve used in computation- 'ISO'/'SAE': use ISO or SAE orientation of the normal for offset computation
Output
XY
: matrix2 x n
of the evaluated pointsX
: vector of the x-coordinates of the evaluated point derivativesY
: vector of the y-coordinates of the evaluated point derivatives
◆ evaluate()
function CurveBase::evaluate | ( | in | self, |
in | s, | ||
in | varargin ) |
Evaluate the curve at curvilinear coordinate s
. Argument s
may be a vector for multiple evaluations.
Usage
Optional Arguments
- offs: offset of the curve used in computation
- 'ISO'/'SAE': use ISO or SAE orientation of the normal for offset computation
◆ find_coord()
function CurveBase::find_coord | ( | in | self, |
in | x, | ||
in | y ) |
Get the curvilinear coordinates of the point (x,y)
Usage:
s
: curvilinear coordinate along the curvet
: curvilinear coordinate along the normal of the curve
◆ info()
function CurveBase::info | ( | in | self | ) |
Print on the console some information on the stored curve.
Usage
◆ intersect()
function CurveBase::intersect | ( | in | self, |
in | OBJ, | ||
in | varargin ) |
Intersect two curves.
Usage
s1
: curvilinear coordinates of the intersections on the first curves2
: curvilinear coordinates of the intersections on the second curve
Optional Argument
offs
,offs1
: offset of the curves used in computation- 'ISO'/'SAE': use ISO or SAE orientation of the normal for the offsets
◆ kappa()
function CurveBase::kappa | ( | in | self, |
in | s ) |
Evaluate the curvature of the curve at curvilinear coordinate s
. Argument s
may be a vector for multiple evaluations.
Usage
◆ kappa_begin()
function CurveBase::kappa_begin | ( | in | self | ) |
Evaluate initial curvature of the curve.
Usage
◆ kappa_D()
function CurveBase::kappa_D | ( | in | self, |
in | s ) |
Evaluate the curvature derivative of the curve at curvilinear coordinate s
. Argument s
may be a vector for multiple evaluations.
Usage
◆ kappa_DD()
function CurveBase::kappa_DD | ( | in | self, |
in | s ) |
Evaluate the curvature second derivative of the curve at curvilinear coordinate s
. Argument s
may be a vector for multiple evaluations.
Usage
◆ kappa_end()
function CurveBase::kappa_end | ( | in | self | ) |
Evaluate final curvature of the curve.
Usage
◆ kappaBegin()
function CurveBase::kappaBegin | ( | in | self | ) |
- Deprecated
- whill be removed in future version
◆ kappaEnd()
function CurveBase::kappaEnd | ( | in | self | ) |
- Deprecated
- whill be removed in future version
◆ length()
function CurveBase::length | ( | in | self, |
in | varargin ) |
Return the length of the curve.
Usage
◆ noAABBtree()
function CurveBase::noAABBtree | ( | in | self | ) |
Deactivate the use of AABB three in intersection/collision computations
◆ obj_handle()
function CurveBase::obj_handle | ( | in | self | ) |
Return the pointer
of the interbal stored c++ object
Usage
◆ plot_bbox()
function CurveBase::plot_bbox | ( | in | self, |
in | varargin ) |
Plot the bounding box of the curve
Usage:
◆ plot_tbox()
function CurveBase::plot_tbox | ( | in | self, |
in | P1, | ||
in | P2, | ||
in | P3, | ||
in | varargin ) |
Plot a triangle BBOX
Usage:
◆ plot_triangles()
function CurveBase::plot_triangles | ( | in | self, |
in | varargin ) |
Plot the covering triangles of the curve
Usage:
◆ plotBBox()
function CurveBase::plotBBox | ( | in | self, |
in | varargin ) |
- Deprecated
- whill be removed in future version
◆ plotTBox()
function CurveBase::plotTBox | ( | in | self, |
in | P1, | ||
in | P2, | ||
in | P3, | ||
in | varargin ) |
- Deprecated
- whill be removed in future version
◆ plotTriangles()
function CurveBase::plotTriangles | ( | in | self, |
in | varargin ) |
- Deprecated
- whill be removed in future version
◆ reverse()
function CurveBase::reverse | ( | in | self | ) |
Reverse the direction of travel of the curve.
Usage
◆ rotate()
function CurveBase::rotate | ( | in | self, |
in | angle, | ||
in | cx, | ||
in | cy ) |
Rotate the curve by angle angle
around point (cx, cy)
Usage
◆ scale()
function CurveBase::scale | ( | in | self, |
in | sc ) |
Scale the curve by factor sc
Usage
◆ theta()
function CurveBase::theta | ( | in | self, |
in | s ) |
Evaluate the angle of the curve at curvilinear coordinate s
. Argument s
may be a vector for multiple evaluations.
Usage
◆ theta_begin()
function CurveBase::theta_begin | ( | in | self | ) |
Evaluate initial angle of the curve.
Usage
◆ theta_D()
function CurveBase::theta_D | ( | in | self, |
in | s ) |
Evaluate the angle derivatives (curvature) of the curve at curvilinear coordinate s
. Argument s
may be a vector for multiple evaluations.
Usage
◆ theta_DD()
function CurveBase::theta_DD | ( | in | self, |
in | s ) |
Evaluate the angle second derivatuve of the curve at curvilinear coordinate s
. Argument s
may be a vector for multiple evaluations.
Usage
◆ theta_DDD()
function CurveBase::theta_DDD | ( | in | self, |
in | s ) |
Evaluate the angle third derivative of the curve at curvilinear coordinate s
. Argument s
may be a vector for multiple evaluations.
Usage
◆ theta_end()
function CurveBase::theta_end | ( | in | self | ) |
Evaluate final angle of the curve.
Usage
◆ thetaBegin()
function CurveBase::thetaBegin | ( | in | self | ) |
- Deprecated
- whill be removed in future version
◆ thetaEnd()
function CurveBase::thetaEnd | ( | in | self | ) |
- Deprecated
- whill be removed in future version
◆ translate()
function CurveBase::translate | ( | in | self, |
in | tx, | ||
in | ty ) |
Translate the curve by (tx,ty)
Usage
◆ trim()
function CurveBase::trim | ( | in | self, |
in | smin, | ||
in | smax ) |
Cut the curve at the curvilinear parameter smin
up to smax
Usage
ref.trim( smin, smax );
◆ x_begin()
function CurveBase::x_begin | ( | in | self | ) |
Evaluate initial x-coordinate of the curve.
Usage
◆ x_end()
function CurveBase::x_end | ( | in | self | ) |
Evaluate final x-coordinate of the curve.
Usage
◆ xBegin()
function CurveBase::xBegin | ( | in | self | ) |
- Deprecated
- whill be removed in future version
◆ xEnd()
function CurveBase::xEnd | ( | in | self | ) |
- Deprecated
- whill be removed in future version
◆ xy_begin()
function CurveBase::xy_begin | ( | in | self | ) |
Evaluate initial point of the curve.
Usage
◆ xy_end()
function CurveBase::xy_end | ( | in | self | ) |
Evaluate final point of the curve.
Usage
◆ xyBegin()
function CurveBase::xyBegin | ( | in | self | ) |
- Deprecated
- whill be removed in future version
◆ xyEnd()
function CurveBase::xyEnd | ( | in | self | ) |
- Deprecated
- whill be removed in future version
◆ y_begin()
function CurveBase::y_begin | ( | in | self | ) |
Evaluate initial y-coordinate of the curve.
Usage
◆ y_end()
function CurveBase::y_end | ( | in | self | ) |
Evaluate final y-coordinate of the curve.
Usage
◆ yBegin()
function CurveBase::yBegin | ( | in | self | ) |
- Deprecated
- whill be removed in future version
◆ yEnd()
function CurveBase::yEnd | ( | in | self | ) |
- Deprecated
- whill be removed in future version
◆ yesAABBtree()
function CurveBase::yesAABBtree | ( | in | self | ) |
Activate the use of AABB three in intersection/collision computations
The documentation for this class was generated from the following file:
- /Users/enrico/Ricerca/develop/PINS/pins-mechatronix/LibSources/submodules/Clothoids/toolbox/lib/CurveBase.m
Generated by