Biarc Class Reference
Clothoids
|
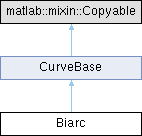
Public Member Functions | |
function | Biarc (in varargin) |
MATLAB class wrapper for the underlying C++ class. | |
function | build (in self, in x0, in y0, in theta0, in x1, in y1, in theta1) |
function | build_3P (in self, in varargin) |
function | x_middle (in self) |
function | xMiddle (in self) |
function | y_middle (in self) |
function | yMiddle (in self) |
function | theta_middle (in self) |
function | thetaMiddle (in self) |
function | kappa0 (in self) |
function | kappa1 (in self) |
function | length0 (in self) |
function | length1 (in self) |
function | getData (in self) |
function | get_circles (in self) |
function | getCircles (in self) |
function | to_nurbs (in self) |
function | find_coord (in self, in x, in y) |
function | plot (in self, in npts, in varargin) |
function | plot_curvature (in self, in npts, in varargin) |
function | plotCurvature (in self, in npts, in varargin) |
function | plot_angle (in self, in npts, in varargin) |
function | plotAngle (in self, in npts, in varargin) |
function | plot_normal (in self, in step, in len) |
function | plotNormal (in self, in step, in len) |
![]() | |
function | CurveBase (in mexName, in objectType) |
function | obj_handle (in self) |
function | is_type (in self) |
function | load (in self, in OBJ) |
function | bbox (in self, in varargin) |
function | translate (in self, in tx, in ty) |
function | trim (in self, in smin, in smax) |
function | rotate (in self, in angle, in cx, in cy) |
function | reverse (in self) |
function | scale (in self, in sc) |
function | change_origin (in self, in newX0, in newY0) |
function | changeOrigin (in self, in newX0, in newY0) |
function | evaluate (in self, in s, in varargin) |
function | eval (in self, in varargin) |
function | eval_D (in self, in varargin) |
function | eval_DD (in self, in varargin) |
function | eval_DDD (in self, in varargin) |
function | theta (in self, in s) |
function | theta_D (in self, in s) |
function | theta_DD (in self, in s) |
function | theta_DDD (in self, in s) |
function | kappa (in self, in s) |
function | kappa_D (in self, in s) |
function | kappa_DD (in self, in s) |
function | xy_begin (in self) |
function | xyBegin (in self) |
function | xy_end (in self) |
function | xyEnd (in self) |
function | x_begin (in self) |
function | xBegin (in self) |
function | x_end (in self) |
function | xEnd (in self) |
function | y_begin (in self) |
function | yBegin (in self) |
function | y_end (in self) |
function | yEnd (in self) |
function | theta_begin (in self) |
function | thetaBegin (in self) |
function | theta_end (in self) |
function | thetaEnd (in self) |
function | kappa_begin (in self) |
function | kappaBegin (in self) |
function | kappa_end (in self) |
function | kappaEnd (in self) |
function | length (in self, in varargin) |
function | points (in self) |
function | bbTriangles (in self, in varargin) |
function | closest_point (in self, in qx, in qy, in varargin) |
function | closestPoint (in self, in qx, in qy, in varargin) |
function | distance (in self, in qx, in qy, in varargin) |
function | collision (in self, in OBJ, in varargin) |
function | intersect (in self, in OBJ, in varargin) |
function | info (in self) |
function | find_coord (in self, in x, in y) |
function | yesAABBtree (in self) |
function | noAABBtree (in self) |
function | plot_tbox (in self, in P1, in P2, in P3, in varargin) |
function | plotTBox (in self, in P1, in P2, in P3, in varargin) |
function | plot_bbox (in self, in varargin) |
function | plotBBox (in self, in varargin) |
function | plot_triangles (in self, in varargin) |
function | plotTriangles (in self, in varargin) |
Additional Inherited Members | |
![]() | |
function | copyElement (in self) |
![]() | |
Property | mexName |
Property | objectHandle |
Property | call_delete |
Property | objectType |
Constructor & Destructor Documentation
◆ Biarc()
function Biarc::Biarc | ( | in | varargin | ) |
MATLAB class wrapper for the underlying C++ class.
Create a new C++ class instance for the Biarc object
Usage:
Optional Arguments:
x0
,y0
: coordinate of initial pointtheta0
: orientation of the clothoid at initial pointx1
,y1
: coordinate of final pointtheta1
: orientation of the clothoid at final point
On output:
- self: reference handle to the object instance
Member Function Documentation
◆ build()
function Biarc::build | ( | in | self, |
in | x0, | ||
in | y0, | ||
in | theta0, | ||
in | x1, | ||
in | y1, | ||
in | theta1 ) |
Build the interpolating G1 biarc
Usage:
On input:
x0
,y0
: coordinate of initial pointtheta0
: orientation of the clothoid at initial pointx1
,y1
: coordinate of final pointtheta1
: orientation of the clothoid at final point
◆ build_3P()
function Biarc::build_3P | ( | in | self, |
in | varargin ) |
Build the interpolating biarc by 3 points
Usage:
On input:
x0
,y0
: coordinate of initial pointx1
,y1
: coordinate of middle pointx2
,y2
: coordinate of final point
alternative
p0: coordinate of initial point p1: coordinate of middle point p2: coordinate of final point
◆ find_coord()
function Biarc::find_coord | ( | in | self, |
in | x, | ||
in | y ) |
Get the curvilinear coordinates of the point (x,y)
Usage:
s
: curvilinear coordinate along the curvet
: curvilinear coordinate along the normal of the curve
◆ get_circles()
function Biarc::get_circles | ( | in | self | ) |
Get the two circle arc composing a biarc
Usage:
◆ getData()
function Biarc::getData | ( | in | self | ) |
Get the biarc G1 data
Usage:
x0
,y0
: initial point of the biarctheta0
: initial angle of the biarcx1
,y1
: final point of the biarctheta1
: final angle of the biarc
◆ kappa0()
function Biarc::kappa0 | ( | in | self | ) |
Get curvature of the first arc of the biarc
Usage:
◆ kappa1()
function Biarc::kappa1 | ( | in | self | ) |
Get curvature of the second arc of the biarc
Usage:
◆ length0()
function Biarc::length0 | ( | in | self | ) |
Get length of the first arc of the biarc
Usage:
◆ length1()
function Biarc::length1 | ( | in | self | ) |
Get length of the second arc of the biarc
Usage:
◆ plot()
function Biarc::plot | ( | in | self, |
in | npts, | ||
in | varargin ) |
Plot the biarc
Usage:
npts
: number of sampling points for plottingfmt1
: format of the first arcfmt2
: format of the second arc
◆ plot_angle()
function Biarc::plot_angle | ( | in | self, |
in | npts, | ||
in | varargin ) |
Plot the angle of the biarc
Usage:
npts
: number of sampling points for plottingfmt1
: format of the first arcfmt2
: format of the second arc
◆ plot_curvature()
function Biarc::plot_curvature | ( | in | self, |
in | npts, | ||
in | varargin ) |
Plot the curvature of the biarc
Usage:
npts
: number of sampling points for plottingfmt1
: format of the first arcfmt2
: format of the second arc
◆ plot_normal()
function Biarc::plot_normal | ( | in | self, |
in | step, | ||
in | len ) |
Plot the normal of the biarc
Usage:
step
: number of sampling normalslen
: length of the plotted normal
◆ plotAngle()
function Biarc::plotAngle | ( | in | self, |
in | npts, | ||
in | varargin ) |
- Deprecated
- whill be removed in future version
◆ plotCurvature()
function Biarc::plotCurvature | ( | in | self, |
in | npts, | ||
in | varargin ) |
- Deprecated
- whill be removed in future version
◆ plotNormal()
function Biarc::plotNormal | ( | in | self, |
in | step, | ||
in | len ) |
- Deprecated
- whill be removed in future version
◆ theta_middle()
function Biarc::theta_middle | ( | in | self | ) |
Get junction point angle of the biarc
Usage:
◆ thetaMiddle()
function Biarc::thetaMiddle | ( | in | self | ) |
- Deprecated
- whill be removed in future version
◆ to_nurbs()
function Biarc::to_nurbs | ( | in | self | ) |
Return the nurbs represantation of the two arc composing the biarc
Usage:
arc0
: the nurbs of the first arcarc1
: the nurbs of the second arc
◆ x_middle()
function Biarc::x_middle | ( | in | self | ) |
Get junction point of the biarc x-coordinate
Usage:
◆ xMiddle()
function Biarc::xMiddle | ( | in | self | ) |
- Deprecated
- whill be removed in future version
◆ y_middle()
function Biarc::y_middle | ( | in | self | ) |
Get junction point of the biarc y-coordinate
Usage:
◆ yMiddle()
function Biarc::yMiddle | ( | in | self | ) |
- Deprecated
- whill be removed in future version
The documentation for this class was generated from the following file:
- /Users/enrico/Ricerca/develop/PINS/pins-mechatronix/LibSources/submodules/Clothoids/toolbox/lib/Biarc.m
Generated by