CircleArc Class Reference
Clothoids
|
CircleArc Class Reference
Inheritance diagram for CircleArc:
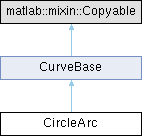
Public Member Functions | |
function | CircleArc (in varargin) |
function | build (in self, in x0, in y0, in theta0, in k0, in L) |
function | build_G1 (in self, in varargin) |
function | build_3P (in self, in varargin) |
function | scale (in self, in sc) |
function | change_curvilinear_origin (in self, in s0, in L) |
function | changeCurvilinearOrigin (in self, in s0, in L) |
function | to_nurbs (in self) |
return a nurbs representation of the circle arc | |
function | plot (in self, in npts, in fmt) |
function | export (in self) |
function | plot_polygon (in self, in varargin) |
function | plotPolygon (in self, in varargin) |
![]() | |
function | CurveBase (in mexName, in objectType) |
function | obj_handle (in self) |
function | is_type (in self) |
function | load (in self, in OBJ) |
function | bbox (in self, in varargin) |
function | translate (in self, in tx, in ty) |
function | trim (in self, in smin, in smax) |
function | rotate (in self, in angle, in cx, in cy) |
function | reverse (in self) |
function | scale (in self, in sc) |
function | change_origin (in self, in newX0, in newY0) |
function | changeOrigin (in self, in newX0, in newY0) |
function | evaluate (in self, in s, in varargin) |
function | eval (in self, in varargin) |
function | eval_D (in self, in varargin) |
function | eval_DD (in self, in varargin) |
function | eval_DDD (in self, in varargin) |
function | theta (in self, in s) |
function | theta_D (in self, in s) |
function | theta_DD (in self, in s) |
function | theta_DDD (in self, in s) |
function | kappa (in self, in s) |
function | kappa_D (in self, in s) |
function | kappa_DD (in self, in s) |
function | xy_begin (in self) |
function | xyBegin (in self) |
function | xy_end (in self) |
function | xyEnd (in self) |
function | x_begin (in self) |
function | xBegin (in self) |
function | x_end (in self) |
function | xEnd (in self) |
function | y_begin (in self) |
function | yBegin (in self) |
function | y_end (in self) |
function | yEnd (in self) |
function | theta_begin (in self) |
function | thetaBegin (in self) |
function | theta_end (in self) |
function | thetaEnd (in self) |
function | kappa_begin (in self) |
function | kappaBegin (in self) |
function | kappa_end (in self) |
function | kappaEnd (in self) |
function | length (in self, in varargin) |
function | points (in self) |
function | bbTriangles (in self, in varargin) |
function | closest_point (in self, in qx, in qy, in varargin) |
function | closestPoint (in self, in qx, in qy, in varargin) |
function | distance (in self, in qx, in qy, in varargin) |
function | collision (in self, in OBJ, in varargin) |
function | intersect (in self, in OBJ, in varargin) |
function | info (in self) |
function | find_coord (in self, in x, in y) |
function | yesAABBtree (in self) |
function | noAABBtree (in self) |
function | plot_tbox (in self, in P1, in P2, in P3, in varargin) |
function | plotTBox (in self, in P1, in P2, in P3, in varargin) |
function | plot_bbox (in self, in varargin) |
function | plotBBox (in self, in varargin) |
function | plot_triangles (in self, in varargin) |
function | plotTriangles (in self, in varargin) |
Additional Inherited Members | |
![]() | |
function | copyElement (in self) |
![]() | |
Property | mexName |
Property | objectHandle |
Property | call_delete |
Property | objectType |
Constructor & Destructor Documentation
◆ CircleArc()
function CircleArc::CircleArc | ( | in | varargin | ) |
Create a new C++ class instance for the circle arc object
Usage:
ref = CircleArc() % create empty circle
ref = CircleArc( x0, y0, theta0, k0, L ) % circle passing from (x0,y0)
% at angle theta0 with curvature k0
% and length L
On input:
x0
,y0
: coordinate of initial pointtheta0
: orientation of the circle at initial pointk0
: curvature of the circle at initial pointL
: length of curve from initial to final point
On output:
- ref: reference handle to the object instance
Member Function Documentation
◆ build()
function CircleArc::build | ( | in | self, |
in | x0, | ||
in | y0, | ||
in | theta0, | ||
in | k0, | ||
in | L ) |
Build the circle from known parameters
Usage:
ref.build( x0, y0, theta0, k0, L )
build a circle passing from (x0,y0) at angle theta0 with curvature and length
On input:
x0
,y0
: coordinate of initial pointtheta0
: orientation of the circle at initial pointk0
: curvature of the circle at initial pointL
: length of curve from initial to final point
◆ build_3P()
function CircleArc::build_3P | ( | in | self, |
in | varargin ) |
Build the circle arc given 3 points. The point can be alingned in this case a degenerate straight arc if build.
Usage:
ref.build_3P( x0, y0, x1, y1, x2, y2 )
ref.build_3P( p0, p1, p2 )
◆ build_G1()
function CircleArc::build_G1 | ( | in | self, |
in | varargin ) |
Build the circle from known parameters
Usage:
ref.build( x0, y0, theta0, x1, y1 ); % circle passing to [x0,y0] and [x1,y1]
% with angle theta0 at [x0,y0]
ref.build( p0, theta0, p1 ); % circle passing to p0 and p1 with angle theta0 at p0
On input:
x0
,y0
: coordinate of initial pointtheta0
: orientation of the circle at initial pointk0
: curvature of the circle at initial pointL
: length of curve from initial to final pointp0
: 2D pointp1
: 2D point
◆ change_curvilinear_origin()
function CircleArc::change_curvilinear_origin | ( | in | self, |
in | s0, | ||
in | L ) |
Change the origin of the circle curve to s0
and set arc lenght to L
Usage:
ref.change_curvilinear_origin( s0, L );
◆ changeCurvilinearOrigin()
function CircleArc::changeCurvilinearOrigin | ( | in | self, |
in | s0, | ||
in | L ) |
- Deprecated
- whill be removed in future version
◆ export()
function CircleArc::export | ( | in | self | ) |
Export circle parameters
Usage:
S = ref.export();
S.x0
,S.y0
: initial point of the circle arcS.x1
,S.y1
: final point of the circle arcS.theta0
: initial angle of the circle arcS.theta1
: final angle of the circle arcS.kappa
: curvature of the circle arcS.L
: length of the clothoid arc
◆ plot()
function CircleArc::plot | ( | in | self, |
in | npts, | ||
in | fmt ) |
Plot the arc
Usage:
ref.plot( npts );
fmt = {'Color','blue','Linewidth',2};
ref.plot( npts, fmt );
npts
: number of sampling points for plottingfmt
: format of the arc
◆ plot_polygon()
function CircleArc::plot_polygon | ( | in | self, |
in | varargin ) |
Plot the polygon of the NURBS for the arc
Usage:
ref.plotPolygon();
ref.plotPolygon( 'Color','blue','Linewidth',2 );
fmt
: format of the arc
◆ plotPolygon()
function CircleArc::plotPolygon | ( | in | self, |
in | varargin ) |
- Deprecated
- whill be removed in future version
◆ scale()
function CircleArc::scale | ( | in | self, |
in | sc ) |
Scale circle by sc
factor
Usage:
ref.scale( sc );
The documentation for this class was generated from the following file:
- /Users/enrico/Ricerca/develop/PINS/pins-mechatronix/LibSources/submodules/Clothoids/toolbox/lib/CircleArc.m
Generated by