BiQuinticSplineBase Class ReferenceΒΆ
Splines
|
Bi-quintic spline base class. More...
#include <SplineBiQuintic.hxx>
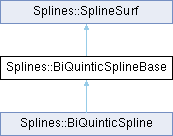
Public Member Functions | |
BiQuinticSplineBase (string const &name="Spline") | |
spline constructor | |
Estimated derivatives at interpolation nodes | |
real_type | Dx_node (integer i, integer j) const |
real_type | Dy_node (integer i, integer j) const |
real_type | Dxx_node (integer i, integer j) const |
real_type | Dyy_node (integer i, integer j) const |
real_type | Dxy_node (integer i, integer j) const |
Evaluate | |
real_type | eval (real_type x, real_type y) const override |
void | D (real_type x, real_type y, real_type d[3]) const override |
real_type | Dx (real_type x, real_type y) const override |
real_type | Dy (real_type x, real_type y) const override |
void | DD (real_type x, real_type y, real_type dd[6]) const override |
real_type | Dxx (real_type x, real_type y) const override |
real_type | Dxy (real_type x, real_type y) const override |
real_type | Dyy (real_type x, real_type y) const override |
![]() | |
SplineSurf (string const &name="Spline") | |
virtual | ~SplineSurf () |
void | clear () |
virtual void | write_to_stream (ostream_type &s) const =0 |
virtual char const * | type_name () const =0 |
virtual string | info () const |
void | info (ostream_type &stream) const |
void | dump_data (ostream_type &s) const |
bool | is_x_closed () const |
void | make_x_closed () |
void | make_x_opened () |
bool | is_y_closed () const |
void | make_y_closed () |
void | make_y_opened () |
bool | is_x_bounded () const |
void | make_x_unbounded () |
void | make_x_bounded () |
bool | is_y_bounded () const |
void | make_y_unbounded () |
void | make_y_bounded () |
string const & | name () const |
integer | num_point_x () const |
integer | num_point_y () const |
real_type | x_node (integer i) const |
real_type | y_node (integer i) const |
real_type | z_node (integer i, integer j) const |
real_type | x_min () const |
real_type | x_max () const |
real_type | y_min () const |
real_type | y_max () const |
real_type | z_min () const |
real_type | z_max () const |
void | build (real_type const x[], integer incx, real_type const y[], integer incy, real_type const z[], integer ldZ, integer nx, integer ny, bool fortran_storage=false, bool transposed=false) |
void | build (vector< real_type > const &x, vector< real_type > const &y, vector< real_type > const &z, bool fortran_storage=false, bool transposed=false) |
void | build (real_type const z[], integer ldZ, integer nx, integer ny, bool fortran_storage=false, bool transposed=false) |
void | build (vector< real_type > const &z, integer nx, integer ny, bool fortran_storage=false, bool transposed=false) |
void | setup (GenericContainer const &gc) |
void | build (GenericContainer const &gc) |
real_type | operator() (real_type x, real_type y) const |
real_type | eval_D_1 (real_type x, real_type y) const |
real_type | eval_D_2 (real_type x, real_type y) const |
real_type | eval_D_1_1 (real_type x, real_type y) const |
real_type | eval_D_1_2 (real_type x, real_type y) const |
real_type | eval_D_2_2 (real_type x, real_type y) const |
Detailed Description
Bi-quintic spline base class.
Member Function Documentation
◆ D()
|
overridevirtual |
Evaluate spline with derivative at point \( (x,y) \)
d[0]
the value of the splined[1]
the value of the splinex
derivatived[2]
the value of the spliney
derivative
Implements Splines::SplineSurf.
◆ DD()
|
overridevirtual |
Evaluate spline with derivative at point \( (x,y) \)
d[0]
the value of the splined[1]
the value of the splinex
derivatived[2]
the value of the spliney
derivatived[3]
the value of the splinex
second derivatived[4]
the value of the spliney
second derivatived[5]
the value of the splinexy
mixed derivative
Implements Splines::SplineSurf.
◆ Dx()
Evaluate spline x
derivative at point \( (x,y) \)
Implements Splines::SplineSurf.
◆ Dx_node()
Estimated x
derivatives at node (i,j)
◆ Dxx()
Evaluate spline x
second derivative at point \( (x,y) \)
Implements Splines::SplineSurf.
◆ Dxx_node()
Estimated x
second derivatives at node (i,j)
◆ Dxy()
Evaluate spline xy
mixed derivative at point \( (x,y) \)
Implements Splines::SplineSurf.
◆ Dxy_node()
Estimated mixed xy
derivatives at node (i,j)
◆ Dy()
Evaluate spline y
derivative at point \( (x,y) \)
Implements Splines::SplineSurf.
◆ Dy_node()
Estimated y
derivatives at node (i,j)
◆ Dyy()
Evaluate spline y
second derivative at point \( (x,y) \)
Implements Splines::SplineSurf.
◆ Dyy_node()
Estimated y
derivatives at node (i,j)
◆ eval()
Evaluate spline at point \( (x,y) \)
Implements Splines::SplineSurf.
The documentation for this class was generated from the following files:
- /Users/enrico/Ricerca/develop/PINS/pins-mechatronix/LibSources/submodules/Splines/src/Splines/SplineBiQuintic.hxx
- /Users/enrico/Ricerca/develop/PINS/pins-mechatronix/LibSources/submodules/Splines/src/SplinesBivariate.cc
Generated by