SplineVec Class ReferenceΒΆ
Splines
|
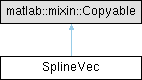
Public Member Functions | |
function | SplineVec () |
function | setup (in self, in P) |
function | knots (in self, in t) |
function | get_knots (in self) |
function | chordal (in self) |
function | centripetal (in self) |
function | CatmullRom (in self) |
function | eval (in self, in x) |
function | eval_D (in self, in x) |
function | eval_DD (in self, in x) |
function | eval_DDD (in self, in x) |
function | curvature (in self, in x) |
function | curvature_D (in self, in x) |
function | tmin (in self, in x) |
function | tmax (in self, in x) |
Protected Member Functions | |
function | copyElement (in self) |
Detailed Description
MATLAB class wrapper for the underlying C++ class
The construction of the spline is done as follows
- instantiate the spline object
- load the points as a matrix
dim x npts
wheredim
is the space dimension andnpts
is the number of poinst
- build or load the knots
- build the spline, for the moment only
Catmull Rom
Constructor & Destructor Documentation
◆ SplineVec()
function SplineVec::SplineVec | ( | ) |
Build an empty parametric spline:
Member Function Documentation
◆ CatmullRom()
function SplineVec::CatmullRom | ( | in | self | ) |
Build the spline using Catmull Rom algorithm
\html_imega{exampleVec.png,width=80%}
◆ centripetal()
function SplineVec::centripetal | ( | in | self | ) |
Build the knots using centripetal parametrization
\begin{eqnarray*} t_0 = 0, \qquad t_k = t_{k-1} + || \mathbf{p}_k - \mathbf{p}_{k-1} ||^{1/2} \end{eqnarray*}
◆ chordal()
function SplineVec::chordal | ( | in | self | ) |
Build the knots using chordal distance
\begin{eqnarray*} t_0 = 0, \qquad t_k = t_{k-1} + || \mathbf{p}_k - \mathbf{p}_{k-1} || \end{eqnarray*}
◆ copyElement()
|
protected |
Make a deep copy of a curve object
Usage
where A
is the curve object to be copied.
◆ curvature()
function SplineVec::curvature | ( | in | self, |
in | x ) |
Evaluate spline curvature at x
◆ curvature_D()
function SplineVec::curvature_D | ( | in | self, |
in | x ) |
Evaluate spline curvature derivative at x
◆ eval()
function SplineVec::eval | ( | in | self, |
in | x ) |
Evaluate spline at x
◆ eval_D()
function SplineVec::eval_D | ( | in | self, |
in | x ) |
Evaluate spline derivative at x
◆ eval_DD()
function SplineVec::eval_DD | ( | in | self, |
in | x ) |
Evaluate spline second derivative at x
◆ eval_DDD()
function SplineVec::eval_DDD | ( | in | self, |
in | x ) |
Evaluate spline third derivative at x
◆ get_knots()
function SplineVec::get_knots | ( | in | self | ) |
Get the knots of the spline
◆ knots()
function SplineVec::knots | ( | in | self, |
in | t ) |
Setup the knots of the spline
◆ setup()
function SplineVec::setup | ( | in | self, |
in | P ) |
Setup the point of the spline
◆ tmax()
function SplineVec::tmax | ( | in | self, |
in | x ) |
Return initial t-coordinate of the spline
◆ tmin()
function SplineVec::tmin | ( | in | self, |
in | x ) |
Return initial t-coordinate of the spline
The documentation for this class was generated from the following file:
- /Users/enrico/Ricerca/develop/PINS/pins-mechatronix/LibSources/submodules/Splines/toolbox/lib/SplineVec.m
Generated by