Spline2D Class ReferenceΒΆ
Splines
|
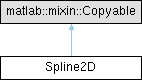
Public Member Functions | |
function | Spline2D (in name, in varargin) |
function | build (in self, in x, in y, in z) |
function | eval (in self, in x, in y) |
function | eval_Dx (in self, in x, in y) |
function | eval_Dy (in self, in x, in y) |
function | eval_Dxx (in self, in x, in y) |
function | eval_Dxy (in self, in x, in y) |
function | eval_Dyy (in self, in x, in y) |
function | make_x_opened (in self) |
function | is_x_closed (in self) |
function | make_x_bounded (in self) |
function | make_x_unbounded (in self) |
function | is_x_bounded (in self) |
function | make_y_opened (in self) |
function | is_y_closed (in self) |
function | make_y_bounded (in self) |
function | make_y_unbounded (in self) |
function | is_y_bounded (in self) |
Protected Member Functions | |
function | copyElement (in self) |
Detailed Description
MATLAB class wrapper for the underlying C++ class.
The construction of the 2D spline is done as follows
- instantiate the spline object
kind
is a string and can be any of
kind | meaning |
---|---|
'bilinear' | piecewise linear in X e Y direction |
'cubic' | piecewise cubic in X e Y direction |
'akima' | piecewise cubic with Akima non oscillatory construction |
'biquintic' | piecewise quintic in X e Y direction |
Evaluation is simple
where X
and Y
are scalar or vector or matrix of the same size. the result Z
is scalar or vector or matrix of the same size of the inputs.
example of usage
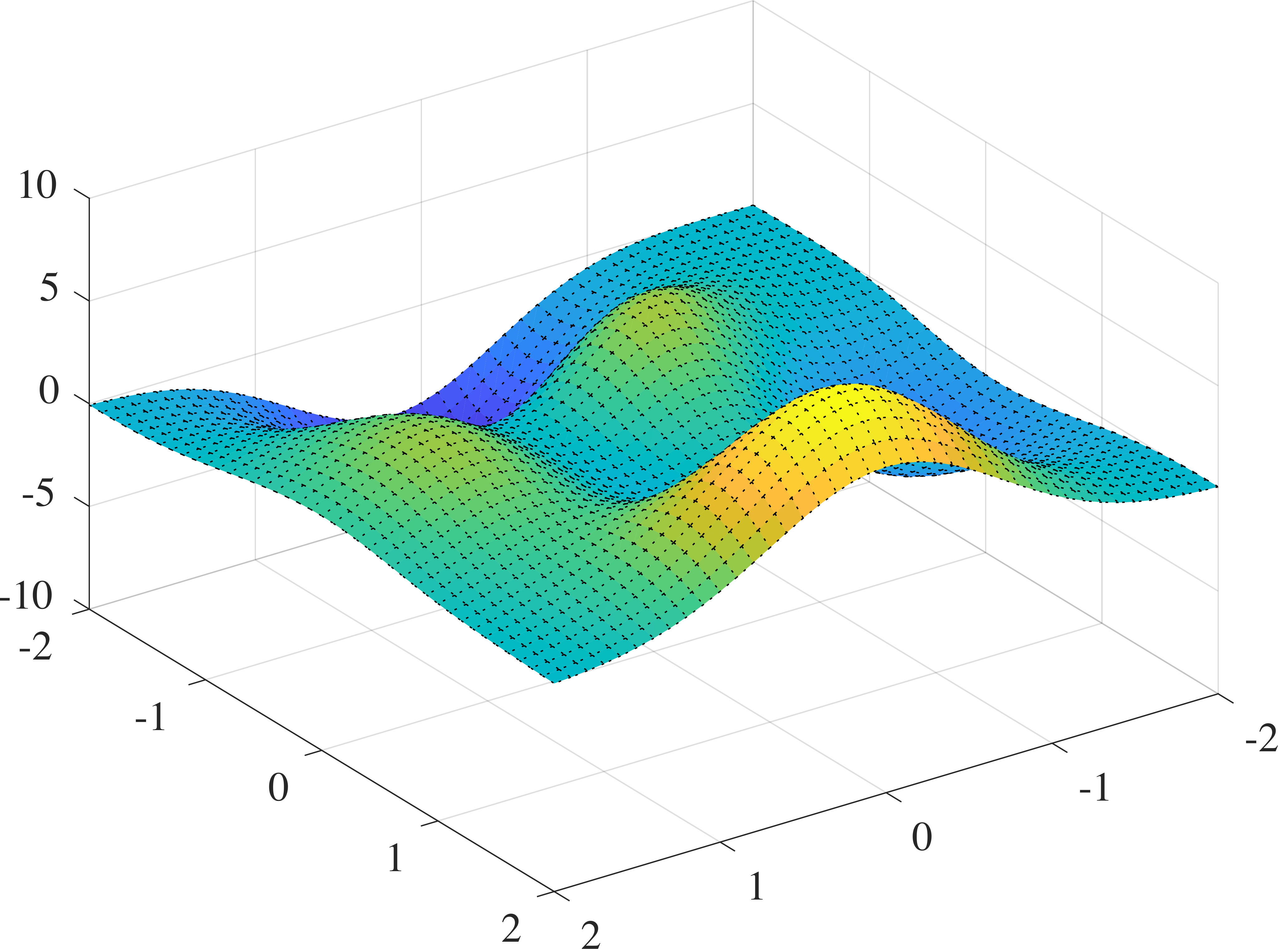
Constructor & Destructor Documentation
◆ Spline2D()
function Spline2D::Spline2D | ( | in | name, |
in | varargin ) |
Build a spline given a table of point and type:
Member Function Documentation
◆ build()
function Spline2D::build | ( | in | self, |
in | x, | ||
in | y, | ||
in | z ) |
Build a spline given a table of point and type:
X
and Y
are vector Z
is a matrix nx x ny
◆ copyElement()
|
protected |
Make a deep copy of a curve object
Usage
where A
is the curve object to be copied.
◆ eval()
function Spline2D::eval | ( | in | self, |
in | x, | ||
in | y ) |
Evaluate spline (and its derivatives) at (x,y)
x
and y
are salar or vector or matrix of the same size
◆ eval_Dx()
function Spline2D::eval_Dx | ( | in | self, |
in | x, | ||
in | y ) |
Evaluate spline x
derivative at (x,y)
x
and y
are salar or vector or matrix of the same size
◆ eval_Dxx()
function Spline2D::eval_Dxx | ( | in | self, |
in | x, | ||
in | y ) |
Evaluate spline x
second derivative at (x,y)
x
and y
are salar or vector or matrix of the same size
◆ eval_Dxy()
function Spline2D::eval_Dxy | ( | in | self, |
in | x, | ||
in | y ) |
Evaluate spline xy
second derivative at (x,y)
x
and y
are salar or vector or matrix of the same size
◆ eval_Dy()
function Spline2D::eval_Dy | ( | in | self, |
in | x, | ||
in | y ) |
Evaluate spline y
derivative at (x,y)
x
and y
are salar or vector or matrix of the same size
◆ eval_Dyy()
function Spline2D::eval_Dyy | ( | in | self, |
in | x, | ||
in | y ) |
Evaluate spline y
second derivative at (x,y)
x
and y
are salar or vector or matrix of the same size
◆ is_x_bounded()
function Spline2D::is_x_bounded | ( | in | self | ) |
Check if spline is computable only in the x
-range where is defined. Return true if can be computed only in the range.
◆ is_x_closed()
function Spline2D::is_x_closed | ( | in | self | ) |
Return true if spline surface is of closed type in x
-direction
◆ is_y_bounded()
function Spline2D::is_y_bounded | ( | in | self | ) |
Check if spline is computable only in the y
-range where is defined. Return true if can be computed only in the range.
◆ is_y_closed()
function Spline2D::is_y_closed | ( | in | self | ) |
Return true if spline surface is of closed type in y
-direction
◆ make_x_bounded()
function Spline2D::make_x_bounded | ( | in | self | ) |
Make spline surface computable only in the x
-range where is defined. If x
is outside range an error is produced.
◆ make_x_opened()
function Spline2D::make_x_opened | ( | in | self | ) |
Set spline surface as closed in `x direction
/ / {matlab} / spl.make_x_closed(); /
/ public: function make_x_closed(in self );
/ / Set spline surface as opened in x direction @iverbatim
{matlab} spl.make_x_opened();
`
◆ make_x_unbounded()
function Spline2D::make_x_unbounded | ( | in | self | ) |
Make spline surface computable outside the x
-range is defined. If x
is outside range value is extrapolated.
◆ make_y_bounded()
function Spline2D::make_y_bounded | ( | in | self | ) |
Make spline surface computable only in the y
-range where is defined. If y
is outside range an error is produced.
◆ make_y_opened()
function Spline2D::make_y_opened | ( | in | self | ) |
Set spline surface as closed in `y direction
/ / {matlab} / spl.make_y_closed(); /
/ public: function make_y_closed(in self );
/ / Set spline surface as opened in y direction @iverbatim
{matlab} spl.make_y_opened();
`
◆ make_y_unbounded()
function Spline2D::make_y_unbounded | ( | in | self | ) |
Make spline surface computable outside the y
-range is defined. If y
is outside range value is extrapolated.
The documentation for this class was generated from the following file:
- /Users/enrico/Ricerca/develop/PINS/pins-mechatronix/LibSources/submodules/Splines/toolbox/lib/Spline2D.m
Generated by