Spline1D Class ReferenceΒΆ
Splines
|
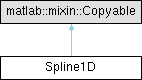
Public Member Functions | |
function | Spline1D (in kind, in varargin) |
function | build (in self, in varargin) |
function | eval_coeffs (in self) |
x, y [, yp or subtype] | |
function | eval_y_min_max (in self) |
function | eval (in self, in x) |
function | eval_D (in self, in x) |
function | eval_DD (in self, in x) |
function | eval_DDD (in self, in x) |
function | eval_DDDD (in self, in x) |
function | eval_DDDDD (in self, in x) |
function | make_closed (in self) |
function | make_opened (in self) |
function | is_closed (in self) |
function | make_bounded (in self) |
function | make_unbounded (in self) |
function | is_bounded (in self) |
function | make_extended_constant (in self) |
function | make_extended_not_constant (in self) |
function | is_extended_constant (in self) |
function | x_begin (in self) |
function | xBegin (in self) |
function | xEnd (in self) |
function | x_end (in self) |
function | y_begin (in self) |
function | yBegin (in self) |
function | y_end (in self) |
function | yEnd (in self) |
function | x_min (in self) |
function | xMin (in self) |
function | x_max (in self) |
function | xMax (in self) |
function | y_min (in self) |
function | yMin (in self) |
function | y_max (in self) |
function | yMax (in self) |
Protected Member Functions | |
function | copyElement (in self) |
Detailed Description
MATLAB class wrapper for the underlying C++ class
Constructor & Destructor Documentation
◆ Spline1D()
function Spline1D::Spline1D | ( | in | kind, |
in | varargin ) |
Build a spline given a table of point and type:
Kind is a string and can be any of
kind | meaning |
---|---|
'linear' | linear spline (only continuous) |
'cubic' | cubic spline ( \( C^2 \) function) |
'akima' | Akima non oscillatory spline ( \( C^1 \) function) |
'bessel' | bessel non oscillatory spline ( \( C^1 \) function) |
'pchip' | Monotone \( C^1 \) function |
'hermite' | Hermite spline (set \( p_k \) and \( p'_k \)) |
'quintic' | Quintic spline ( \( C^3 \) function) |
Member Function Documentation
◆ build()
function Spline1D::build | ( | in | self, |
in | varargin ) |
Build a spline given a table of point and type:
◆ copyElement()
|
protected |
Make a deep copy of a curve object
Usage
where A
is the curve object to be copied.
◆ eval()
function Spline1D::eval | ( | in | self, |
in | x ) |
Evaluate spline at x
returning vaues and derivatives
◆ eval_coeffs()
function Spline1D::eval_coeffs | ( | in | self | ) |
x, y [, yp or subtype]
Return the coeffs of the polynomial of the spline
- [out] coeffs matrix which rows are the polynomial coeffs
- [out] nodes where the spline is defined
◆ eval_D()
function Spline1D::eval_D | ( | in | self, |
in | x ) |
Evaluate spline derivative at x
◆ eval_DD()
function Spline1D::eval_DD | ( | in | self, |
in | x ) |
Evaluate spline second derivative at x
◆ eval_DDD()
function Spline1D::eval_DDD | ( | in | self, |
in | x ) |
Evaluate spline third derivative at x
◆ eval_DDDD()
function Spline1D::eval_DDDD | ( | in | self, |
in | x ) |
Evaluate spline 4th derivative at x
◆ eval_DDDDD()
function Spline1D::eval_DDDDD | ( | in | self, |
in | x ) |
Evaluate spline 5th derivative at x
◆ eval_y_min_max()
function Spline1D::eval_y_min_max | ( | in | self | ) |
Search the max and min values of y
along the spline with the corresponding x
position
- [out] i_min_pos where is the minimum (interval)
- [out] x_min_pos where is the minimum
- [out] y_min the minimum value
- [out] i_max_pos where is the maximum (interval)
- [out] x_max_pos where is the maximum
- [out] y_max the maximum value
- [out] SS.i_min_pos vector where is the local minimum (interval)
- [out] SS.x_min_pos vector where is the local minimum
- [out] SS.y_min vector where is the local minimum value
- [out] SS.i_max_pos vector where is the local maximum (interval)
- [out] SS.x_max_pos vector where is the local maximum
- [out] SS.y_max vector where is the local maximum value
◆ is_bounded()
function Spline1D::is_bounded | ( | in | self | ) |
Check if spline is computable only in the range is defined. Return true if can be computed only in the range.
◆ is_closed()
function Spline1D::is_closed | ( | in | self | ) |
Return true if spline is of closed type
◆ is_extended_constant()
function Spline1D::is_extended_constant | ( | in | self | ) |
Check if extrapolation outside the range as a polynomial
◆ make_bounded()
function Spline1D::make_bounded | ( | in | self | ) |
Make spline computable only in the range is defined. If x
is outside range an error is produced.
◆ make_closed()
function Spline1D::make_closed | ( | in | self | ) |
Set spline as closed
◆ make_extended_constant()
function Spline1D::make_extended_constant | ( | in | self | ) |
Set extrapolation outside the range as a constant spline
◆ make_extended_not_constant()
function Spline1D::make_extended_not_constant | ( | in | self | ) |
Set extrapolation outside the range as a polynomial
◆ make_opened()
function Spline1D::make_opened | ( | in | self | ) |
Set spline as opened
◆ make_unbounded()
function Spline1D::make_unbounded | ( | in | self | ) |
Make spline computable outside the range is defined. If x
is outside range value is extrapolated.
◆ x_begin()
function Spline1D::x_begin | ( | in | self | ) |
Return initial x-coordinate of the spline
◆ x_end()
function Spline1D::x_end | ( | in | self | ) |
- Deprecated
- will be removed in future version
◆ x_max()
function Spline1D::x_max | ( | in | self | ) |
Return maximum x-coordinate of the spline
◆ x_min()
function Spline1D::x_min | ( | in | self | ) |
Return minimum x-coordinate of the spline
◆ xBegin()
function Spline1D::xBegin | ( | in | self | ) |
- Deprecated
- will be removed in future version
◆ xEnd()
function Spline1D::xEnd | ( | in | self | ) |
Return final x-coordinate of the spline
◆ xMax()
function Spline1D::xMax | ( | in | self | ) |
- Deprecated
- will be removed in future version
◆ xMin()
function Spline1D::xMin | ( | in | self | ) |
- Deprecated
- will be removed in future version
◆ y_begin()
function Spline1D::y_begin | ( | in | self | ) |
Return initial y-coordinate of the spline
◆ y_end()
function Spline1D::y_end | ( | in | self | ) |
Return final y-coordinate of the spline
◆ y_max()
function Spline1D::y_max | ( | in | self | ) |
Return maximum y-coordinate of the spline
◆ y_min()
function Spline1D::y_min | ( | in | self | ) |
Return minimum y-coordinate of the spline
◆ yBegin()
function Spline1D::yBegin | ( | in | self | ) |
- Deprecated
- will be removed in future version
◆ yEnd()
function Spline1D::yEnd | ( | in | self | ) |
- Deprecated
- will be removed in future version
◆ yMax()
function Spline1D::yMax | ( | in | self | ) |
- Deprecated
- will be removed in future version
◆ yMin()
function Spline1D::yMin | ( | in | self | ) |
- Deprecated
- will be removed in future version
The documentation for this class was generated from the following file:
- /Users/enrico/Ricerca/develop/PINS/pins-mechatronix/LibSources/submodules/Splines/toolbox/lib/Spline1D.m
Generated by